Computer Applications
Write a program to input marks in English, Maths and Science of 40 students who have passed ICSE Examination 2014. Now, perform the following tasks:
(a) Number of students, who have secured 95% or more in all the subjects.
(b) Number of students, who have secured 90% or more in English, Maths and Science.
Java
Java Iterative Stmts
83 Likes
Answer
import java.util.Scanner;
public class KboatExamResult
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int ta = 0, te = 0, tm = 0, ts = 0;
for (int i = 1; i <= 40; i++) {
System.out.println("Enter marks of student " + i);
System.out.print("English: ");
int eng = in.nextInt();
System.out.print("Maths: ");
int maths = in.nextInt();
System.out.print("Science: ");
int sci = in.nextInt();
if (eng >= 95 && maths >= 95 && sci >= 95)
ta++;
if (eng >= 90)
te++;
if (maths >= 90)
tm++;
if (sci >= 90)
ts++;
}
System.out.println("No. of students >= 95% in all subjects: " + ta);
System.out.println("No. of students >= 90% in English: " + te);
System.out.println("No. of students >= 90% in Maths: " + tm);
System.out.println("No. of students >= 90% in Science: " + ts);
}
}
Variable Description Table
Program Explanation
Output
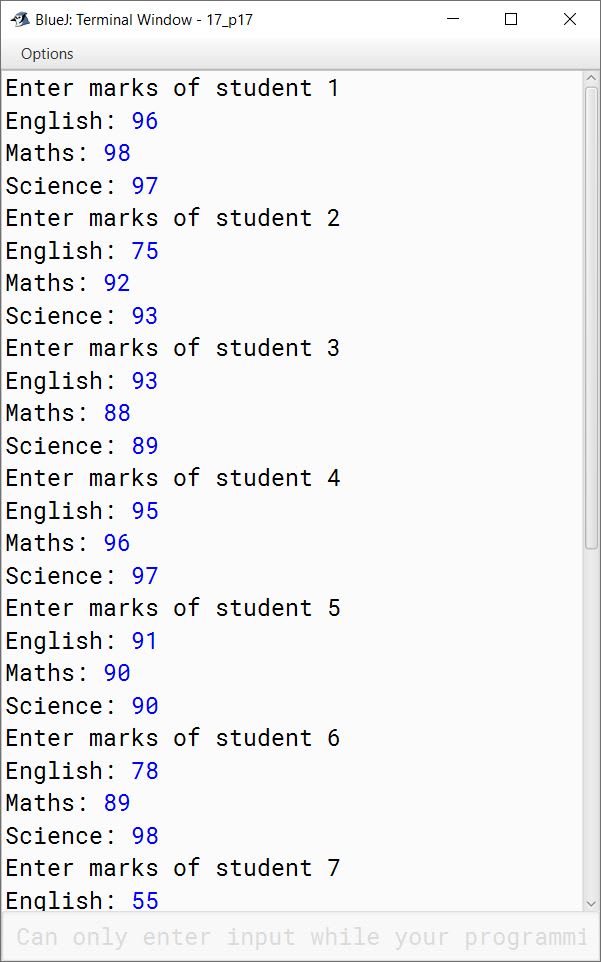
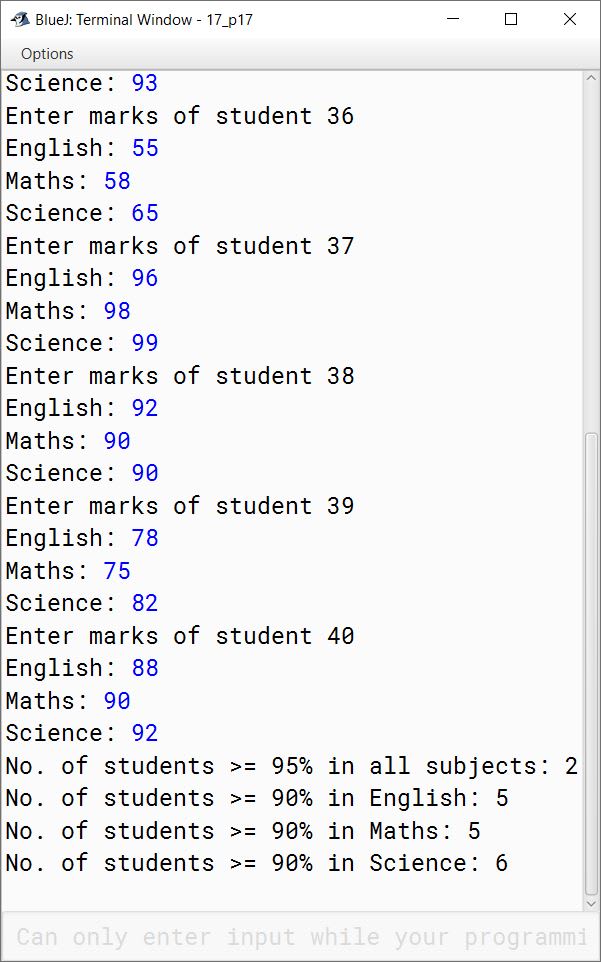
Answered By
29 Likes
Related Questions
Write a program in Java to find the sum of the given series:
1 + (1/2) + (1/3) + …… + (1/20)
Write a program to display all the 'Buzz Numbers' between p and q (where p<q). A 'Buzz Number' is the number which ends with 7 or is divisible by 7.
Write a program in Java to find the sum of the given series:
1 + 4 + 9 + …… + 400
Write a program to display all the numbers between m and n input from the keyboard (where m<n, m>0, n>0), check and print the numbers that are perfect square. e.g. 25, 36, 49, are said to be perfect square numbers.