Computer Applications
Write a program to input integer elements into an array of size 20 and perform the following operations:
- Display largest number from the array
- Display smallest number from the array
- Display sum of all the elements of the array
Java
Java Arrays
ICSE 2017
190 Likes
Answer
import java.util.Scanner;
public class KboatSDAMinMaxSum
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[] = new int[20];
System.out.println("Enter 20 numbers:");
for (int i = 0; i < 20; i++) {
arr[i] = in.nextInt();
}
int min = arr[0], max = arr[0], sum = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i] < min)
min = arr[i];
if (arr[i] > max)
max = arr[i];
sum += arr[i];
}
System.out.println("Largest Number = " + max);
System.out.println("Smallest Number = " + min);
System.out.println("Sum = " + sum);
}
}
Variable Description Table
Program Explanation
Output
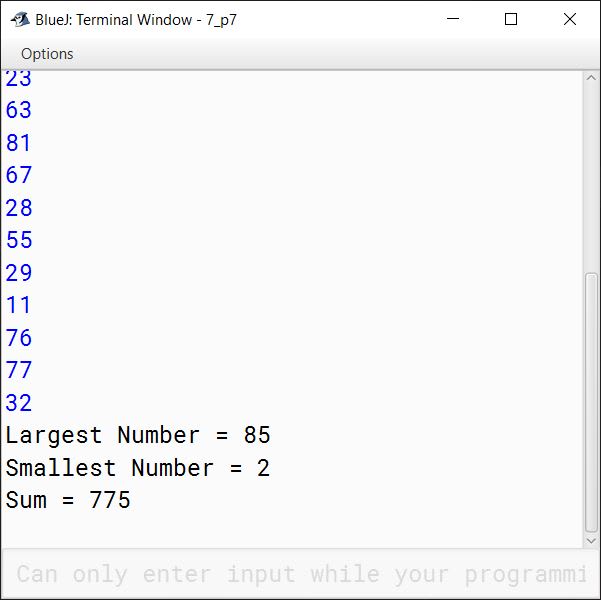
Answered By
67 Likes
Related Questions
Why does Binary Search need a sorted array to perform the search operation?
How does the binary search find the presence of an element quicker than the linear search?
Declare and instantiate a one dimensional int array named evenNums with five elements. Use an initialiser list that contains the first five even integers, starting with 11.
Suppose x is an array of type int[] with 50 elements. Write a code segment that will count and print the frequency of number 42 in the array.