Computer Applications
Write a program to input a sentence and print each word of the string along with its length in tabular form.
Java
Java String Handling
20 Likes
Answer
import java.util.Scanner;
public class KboatWordsLengthTable
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
str += " ";
int len = str.length();
String word = "";
int wLen = 0;
System.out.println("Word Length");
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (ch != ' ') {
word = word + ch;
wLen++;
}
else {
System.out.println(word + "\t" + wLen);
word = "";
wLen = 0;
}
}
}
}
Output
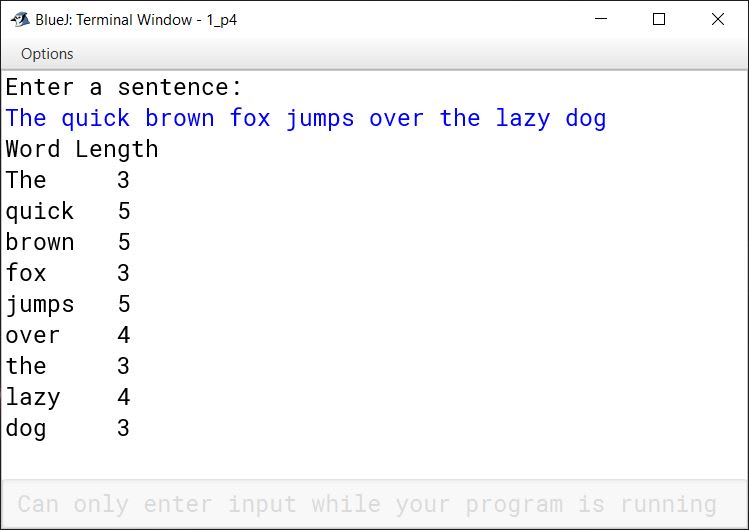
Answered By
9 Likes
Related Questions
Write a program to input a sentence and arrange each word of the string in alphabetical order.
Write a program to input a sentence and arrange words of the string in order of their lengths from shortest to longest.
Differentiate between indexOf() and lastIndexOf().
Differentiate between compareTo() and compareToIgnore().