Computer Applications
Write a program to input a sentence and arrange each word of the string in alphabetical order.
Java
Java String Handling
7 Likes
Answer
import java.util.Scanner;
public class KboatWordsSort
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
str += " ";
int len = str.length();
String word = "";
int wordCount = 0;
char ch;
// calculating no. of words
for (int i = 0; i < len; i++)
if (str.charAt(i) == ' ')
wordCount++;
String wordArr[] = new String[wordCount];
int index = 0;
// storing words in array
for (int i = 0; i < len; i++) {
ch = str.charAt(i);
if (ch != ' ') {
word = word + ch;
}
else {
wordArr[index++] = word;
word = "";
}
}
//bubble sort
for (int i = 0; i < wordCount - 1; i++) {
for (int j = 0; j < wordCount - i - 1; j++) {
if (wordArr[j].compareTo(wordArr[j + 1]) > 1) {
String t = wordArr[j];
wordArr[j] = wordArr[j+1];
wordArr[j+1] = t;
}
}
}
// printing
for(int i = 0; i < wordCount; i++)
System.out.print(wordArr[i] + " ");
}
}
Output
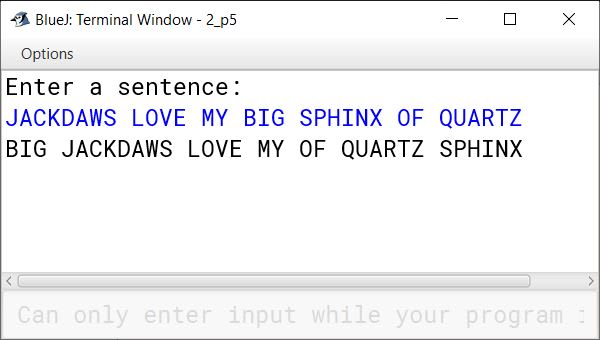
Answered By
4 Likes
Related Questions
Differentiate between compareTo() and compareToIgnore().
Write a program to input a sentence and print each word of the string along with its length in tabular form.
Write a program to input a sentence and arrange words of the string in order of their lengths from shortest to longest.
Write a program to input a sentence. Count and display the frequency of each letter of the sentence in alphabetical order.
Sample Input: COMPUTER APPLICATIONS
Sample Output:Character Frequency Character Frequency A 2 O 2 C 2 P 3 I 1 R 1 L 2 S 1 M 1 T 2 N 1 U 1