Computer Applications
Write a program to input a number and count the number of digits. The program further checks whether the number contains odd number of digits or even number of digits.
Sample Input: 749
Sample Output: Number of digits=3
The number contains odd number of digits.
Java
Java Iterative Stmts
128 Likes
Answer
import java.util.Scanner;
public class KboatDigitCount
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number: ");
int n = in.nextInt();
int dc = 0;
while (n != 0) {
dc++;
n /= 10;
}
System.out.println("Number of digits = " + dc);
if (dc % 2 == 0)
System.out.println("The number contains even number of digits");
else
System.out.println("The number contains odd number of digits");
}
}
Variable Description Table
Program Explanation
Output
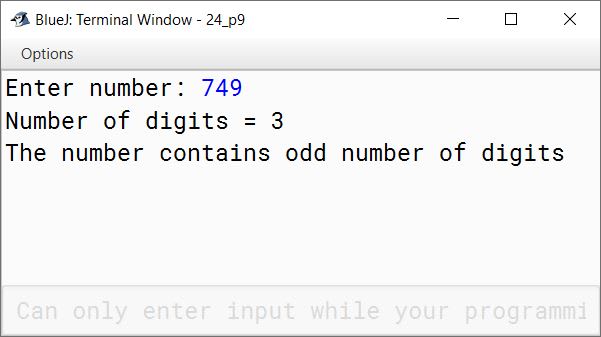
Answered By
46 Likes
Related Questions
Write a program in Java to find the sum of the given series:
2 - 4 + 6 - 8 + …… - 20
Write a program in Java to find the sum of the given series:
(1*2) + (2*3) + …… + (19*20)
Write a program to input a number and display the new number after reversing the digits of the original number. The program also displays the absolute difference between the original number and the reversed number.
Sample Input: 194
Sample Output: 491
Absolute Difference= 297The Greatest Common Divisor (GCD) of two integers is calculated by the continued division method. Divide the larger number by the smaller, the remainder then divides the previous divisor. The process repeats unless the remainder reaches to zero. The last divisor results in GCD.
Sample Input: 45, 20
Sample Output: GCD=5