Computer Applications
Write a program to input 10 integer elements in an array and sort them in descending order using bubble sort technique.
Java
Java Arrays
ICSE 2013
230 Likes
Answer
import java.util.Scanner;
public class KboatBubbleSortDsc
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int n = 10;
int arr[] = new int[n];
System.out.println("Enter the elements of the array:");
for (int i = 0; i < n; i++) {
arr[i] = in.nextInt();
}
//Bubble Sort
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] < arr[j + 1]) {
int t = arr[j];
arr[j] = arr[j+1];
arr[j+1] = t;
}
}
}
System.out.println("Sorted Array:");
for (int i = 0; i < n; i++) {
System.out.print(arr[i] + " ");
}
}
}
Output
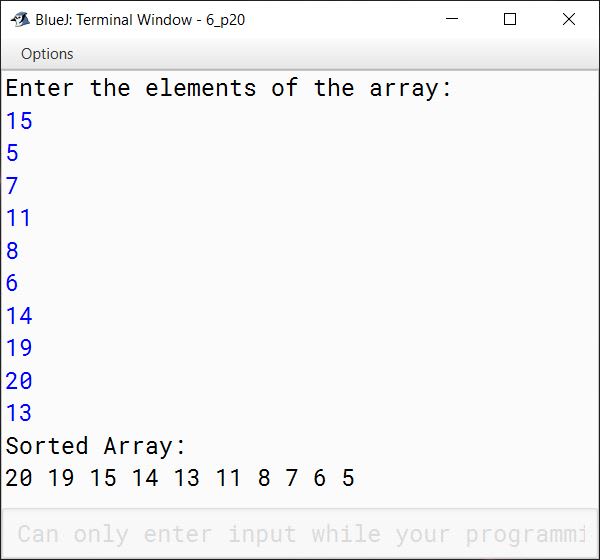
Answered By
84 Likes
Related Questions
Write a program to store 6 elements in an array P and 4 elements in an array Q. Now, produce a third array R, containing all the elements of array P and Q. Display the resultant array.
Input Input Output P[ ] Q[ ] R[ ] 4 19 4 6 23 6 1 7 1 2 8 2 3 3 10 10 19 23 7 8 Write a program to perform binary search on a list of integers given below, to search for an element input by the user. If it is found display the element along with its position, otherwise display the message "Search element not found".
5, 7, 9, 11, 15, 20, 30, 45, 89, 97
Write a program that reads ten integers and displays them in the reverse order in which they were read.
Write a program that reads a long number, counts and displays the occurrences of each digit in it.