Computer Applications
Write a program to generate random integers in the following ranges:
i. 10 to 20 (both inclusive)
ii. 25 to 50 (both inclusive)
Java
Java Math Lib Methods
6 Likes
Answer
public class KboatRandom
{
public static void main(String args[]) {
int min = 10, max = 20;
int range = max - min + 1;
int num = (int)(range * Math.random() + min);
System.out.println("Random number in the range 10 to 20: " + num);
min = 25;
max = 50;
range = max - min + 1;
num = (int)(range * Math.random() + min);
System.out.println("Random number in the range 25 to 50: " + num);
}
}
Output
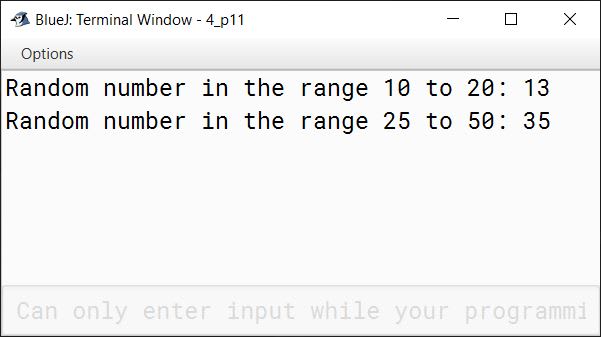
Answered By
5 Likes
Related Questions
Write the equivalent Java statements for the following, by using the mathematical functions:
i. Print the positive value of -999.
ii. Store the value -3375 in a variable and print its cube root.
iii. Store the value 999.99 in a variable and convert it into its closest integer that is greater than or equal to 999.99.
Write a program to compute and display the value of expression:
where, the values of x, y and z are entered by the user.
Write a program that accepts a number x and then prints:
x0, x1, x2, x3, x4, x5
Write a program to print:
i. x to the power y
ii. the square root of y
The values of x and y are 81 and 3, respectively.