Computer Applications
Write a program to enter any 50 numbers and check whether they are divisible by 5 or not. If divisible then perform the following tasks:
(a) Display all the numbers ending with the digit 5.
(b) Count those numbers ending with 0 (zero).
Java
Java Iterative Stmts
96 Likes
Answer
import java.util.Scanner;
public class KboatDivisibleBy5
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int n, c = 0;
System.out.println("Enter 50 numbers");
for (int i = 1; i <= 50; i++) {
n = in.nextInt();
if (n % 5 == 0) {
if (n % 10 == 5)
System.out.println("Number end with the digit 5");
if (n % 10 == 0)
c++;
}
}
System.out.println("Count of numbers ending with 0: " + c);
}
}
Variable Description Table
Program Explanation
Output
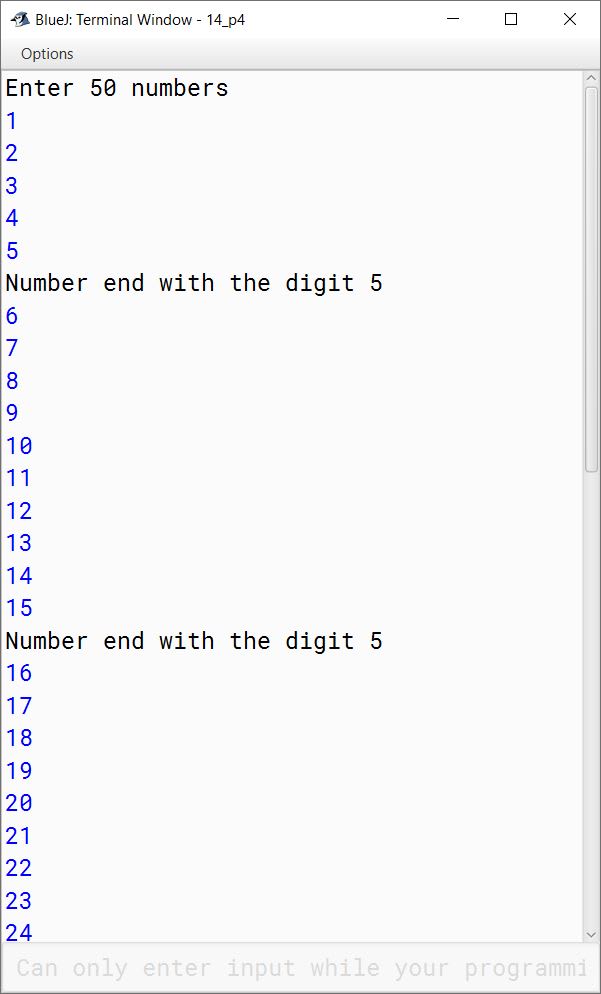
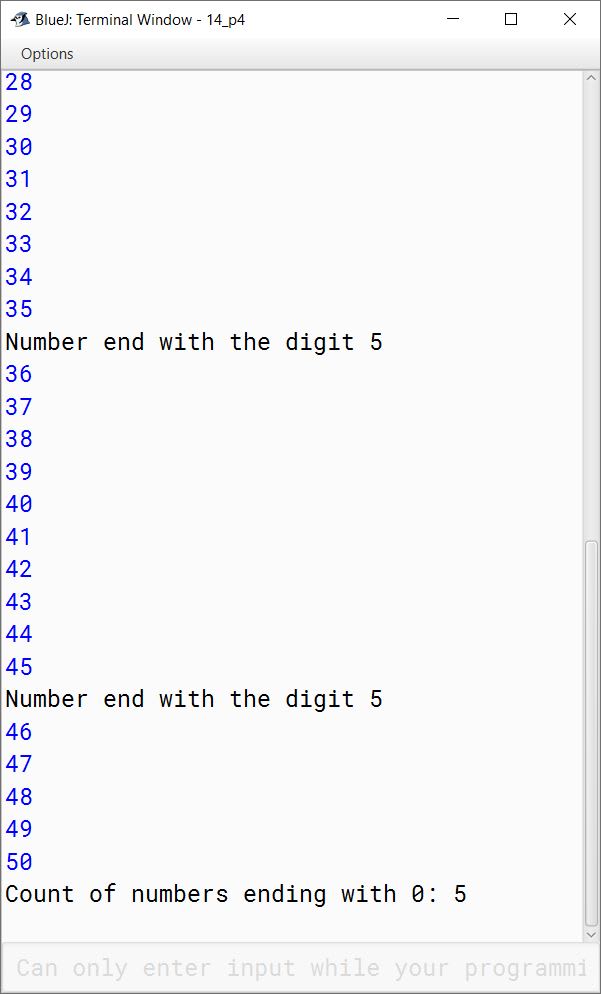
Answered By
31 Likes
Related Questions
Write a program to input any 50 numbers (including positive and negative). Perform the following tasks:
(a) Count the positive numbers
(b) Count the negative numbers
(c) Sum of positive numbers
(d) Sum of negative numbers
Write a program to calculate the sum of all odd numbers and even numbers between a range of numbers from m to n (both inclusive) where m < n. Input m and n (where m < n).
Write a program to display all the 'Buzz Numbers' between p and q (where p<q). A 'Buzz Number' is the number which ends with 7 or is divisible by 7.
Write a program to display all the numbers between m and n input from the keyboard (where m<n, m>0, n>0), check and print the numbers that are perfect square. e.g. 25, 36, 49, are said to be perfect square numbers.