Computer Applications
Write a program to display the Mathematical Table from 5 to 10 for 10 iterations in the given format:
Sample Output: Table of 5
5*1 = 5
5*2 =10
--------
--------
5*10 = 50
Java
Java Nested for Loops
83 Likes
Answer
public class KboatTables
{
public static void main(String args[]) {
for (int i = 5; i <= 10; i++) {
System.out.println("Table of " + i);
for (int j = 1; j <= 10; j++) {
System.out.println(i + "*" + j + " = " + (i*j));
}
}
}
}
Variable Description Table
Program Explanation
Output
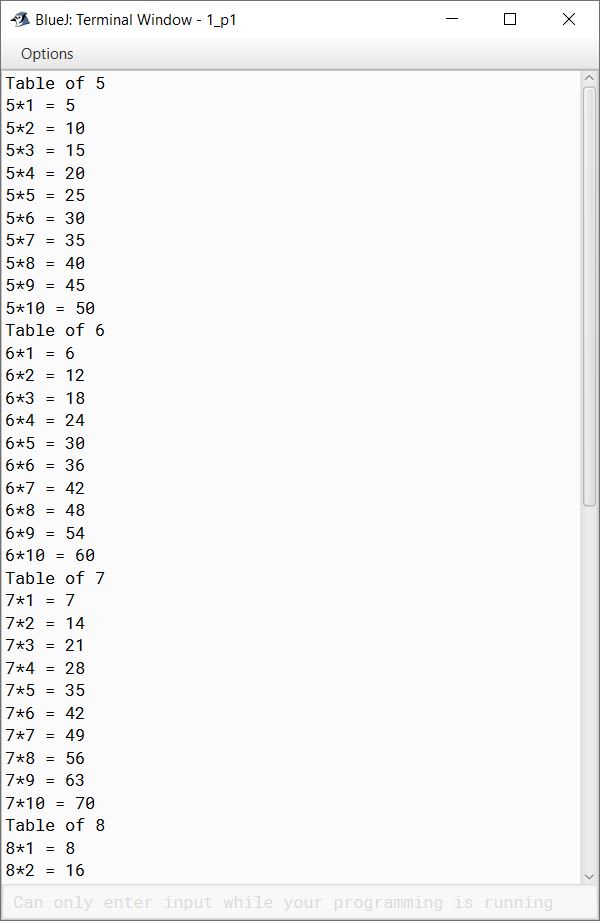
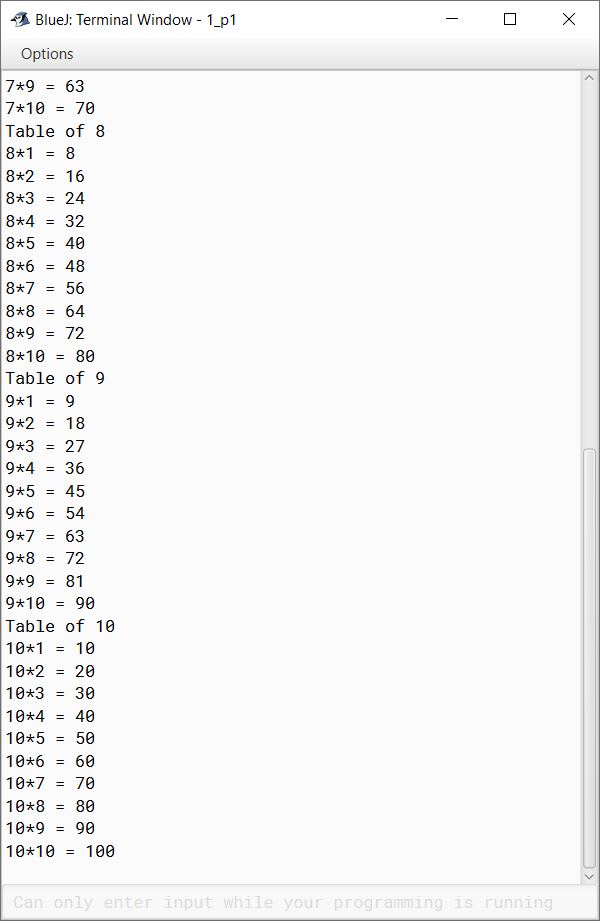
Answered By
44 Likes
Related Questions
Write a program to accept any 20 numbers and display only those numbers which are prime.
Hint: A number is said to be prime if it is only divisible by 1 and the number itself.
Write two separate Java programs to generate the following patterns using iteration (loop) statements:
*
* #
* # *
* # * #
* # * # *Write two separate Java programs to generate the following patterns using iteration (loop) statements:
5 4 3 2 1
5 4 3 2
5 4 3
5 4
5Write a program to compute and display the sum of the following series:
S = (1 + 2) / (1 * 2) + (1 + 2 + 3) / (1 * 2 * 3) + -------- + (1 + 2 + 3 + ----- + n ) / (1 * 2 * 3 * ----- * n)