Computer Applications
Write a program to accept the year of graduation from school as an integer value from the user. Using the binary search technique on the sorted array of integers given below, output the message "Record exists" if the value input is located in the array. If not, output the message "Record does not exist".
Sample Input:
n[0] | n[1] | n[2] | n[3] | n[4] | n[5] | n[6] | n[7] | n[8] | n[9] |
---|---|---|---|---|---|---|---|---|---|
1982 | 1987 | 1993 | 1996 | 1999 | 2003 | 2006 | 2007 | 2009 | 2010 |
Java
Java Arrays
ICSE 2014
123 Likes
Answer
import java.util.Scanner;
public class KboatGraduationYear
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int n[] = {1982, 1987, 1993, 1996, 1999, 2003, 2006, 2007, 2009, 2010};
System.out.print("Enter graduation year to search: ");
int year = in.nextInt();
int l = 0, h = n.length - 1, idx = -1;
while (l <= h) {
int m = (l + h) / 2;
if (n[m] == year) {
idx = m;
break;
}
else if (n[m] < year) {
l = m + 1;
}
else {
h = m - 1;
}
}
if (idx == -1)
System.out.println("Record does not exist");
else
System.out.println("Record exists");
}
}
Variable Description Table
Program Explanation
Output
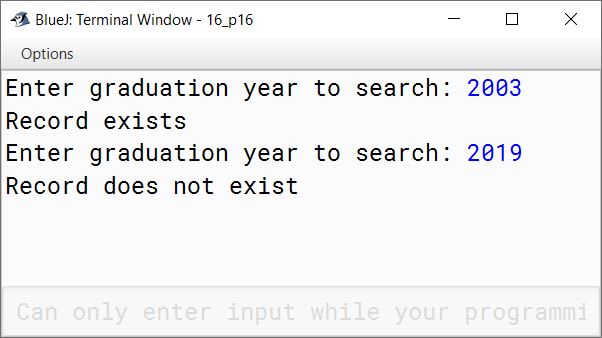
Answered By
42 Likes
Related Questions
The annual examination result of 50 students in a class is tabulated in a Single Dimensional Array (SDA) is as follows:
Roll No. Subject A Subject B Subject C ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. Write a program to read the data, calculate and display the following:
(a) Average marks obtained by each student.
(b) Print the roll number and the average marks of the students whose average is above. 80.
(c) Print the roll number and the average marks of the students whose average is below 40.A double dimensional array is defined as N[4][4] to store numbers. Write a program to find the sum of all even numbers and product of all odd numbers of the elements stored in Double Dimensional Array (DDA).
Sample Input:12 10 15 17 30 11 32 71 17 14 29 31 41 33 40 51
Sample Output:
Sum of all even numbers: ……….
Product of all odd numbers: ……….Write a program to store 6 elements in an array P and 4 elements in an array Q. Now, produce a third array R, containing all the elements of array P and Q. Display the resultant array.
Input Input Output P[ ] Q[ ] R[ ] 4 19 4 6 23 6 1 7 1 2 8 2 3 3 10 10 19 23 7 8 Write a program to input and store roll numbers, names and marks in 3 subjects of n number of students in five single dimensional arrays and display the remark based on average marks as given below:
Average Marks Remark 85 — 100 Excellent 75 — 84 Distinction 60 — 74 First Class 40 — 59 Pass Less than 40 Poor