Computer Science
Write a program to accept a sentence which may be terminated by either '.', '?' or '!' only. The words are to be separated by a single blank space and are in UPPER CASE.
Perform the following tasks:
- Check for the validity of the accepted sentence only for the terminating character.
- Arrange the words in ascending order of their length. If two or more words have the same length, then sort them alphabetically.
- Display the original sentence along with the converted sentence.
Test your program for the following data and some random data:
Example 1:
INPUT:
AS YOU SOW SO SHALL YOU REAP.
OUTPUT:
AS YOU SOW SO SHALL YOU REAP.
AS SO SOW YOU YOU REAP SHALL
Example 2:
INPUT:
SELF HELP IS THE BEST HELP.
OUTPUT:
SELF HELP IS THE BEST HELP.
IS THE BEST HELP HELP SELF
Example 3:
INPUT:
BE KIND TO OTHERS.
OUTPUT:
BE KIND TO OTHERS.
BE TO KIND OTHERS
Example 4:
INPUT:
NOTHING IS IMPOSSIBLE#
OUTPUT:
INVALID INPUT
Java
Java String Handling
ICSE Prac 2020
29 Likes
Answer
import java.util.*;
public class StringCheck
{
public static String sortString(String ipStr) {
StringTokenizer st = new StringTokenizer(ipStr);
int wordCount = st.countTokens();
String strArr[] = new String[wordCount];
for (int i = 0; i < wordCount; i++) {
strArr[i] = st.nextToken();
}
for (int i = 0; i < wordCount - 1; i++) {
for (int j = 0; j < wordCount - i - 1; j++) {
if (strArr[j].length() > strArr[j + 1].length()) {
String t = strArr[j];
strArr[j] = strArr[j+1];
strArr[j+1] = t;
}
if (strArr[j].length() == strArr[j + 1].length()
&&(strArr[j].compareTo(strArr[j+1]) > 0))
{
String t = strArr[j];
strArr[j] = strArr[j+1];
strArr[j+1] = t;
}
}
}
StringBuffer sb = new StringBuffer();
for (int i = 0; i < wordCount; i++) {
sb.append(strArr[i]);
sb.append(" ");
}
/*
* trim will remove the extra space added
* to the end of the string in the loop above
*/
return sb.toString().trim();
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
int len = str.length();
System.out.println();
if (str.charAt(len - 1) != '.'
&& str.charAt(len - 1) != '?'
&& str.charAt(len - 1) != '!') {
System.out.println("INVALID INPUT");
return;
}
/*
* str.substring(0, len - 1) removes the
* '.', '?', '!' at the end of the string
*/
String sortedStr = sortString(str.substring(0, len - 1));
System.out.println(str);
System.out.println(sortedStr);
}
}
Output
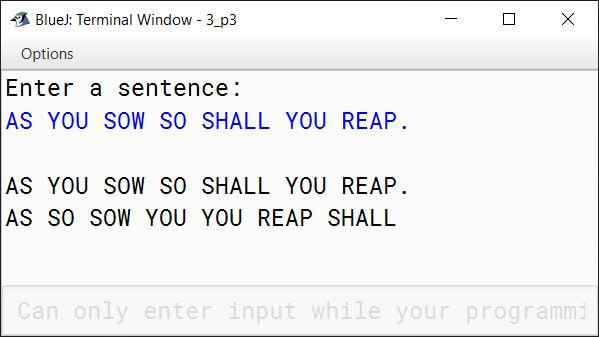
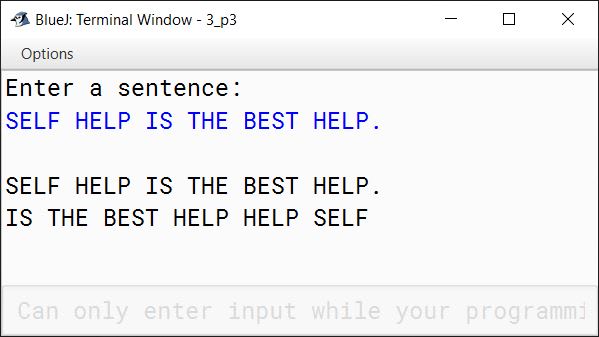
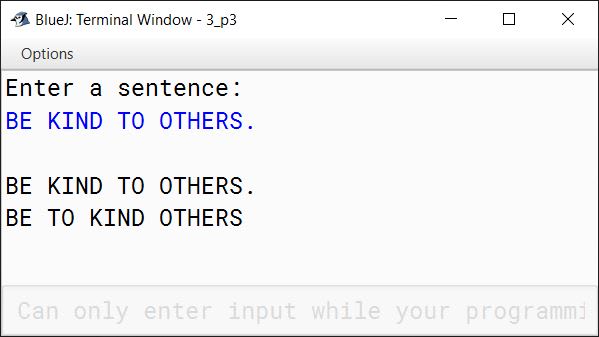
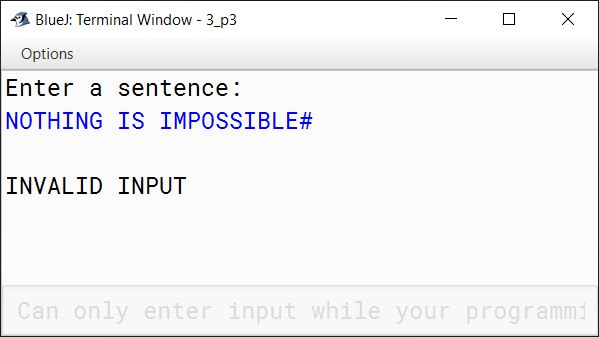
Answered By
8 Likes
Related Questions
A shopping website offers a special discount if the order ID has the sequence 555 anywhere in it. For example, 158545553031, 198555267140, …. .
Fill in the blanks (a) and (b) in the given Java Method to convert the order ID (a long integer) into a string and check if the sequence 555 is present in it.
void checkOrder(long oid) { String str = _______(a)_________; if(______(b)_______) { System.out.println("Special Discount Eligible: " + oid); } }
Define a class to accept the gmail id and check for its validity.
A gmail id is valid only if it has:
→ @
→ .(dot)
→ gmail
→ com
Example:
icse2024@gmail.com
is a valid gmail idWrite the output of the following String methods:
String x= "Galaxy", y= "Games";
(a) System.out.println(x.charAt(0)==y.charAt(0));
(b) System.out.println(x.compareTo(y));
Consider the following program segment in which the statements are jumbled, choose the correct order of statements to check if a given word is Palindrome or not.
boolean palin(String w) { boolean isPalin; w = w.toUpperCase(); int l = w.length(); isPalin = false; // Stmt (1) for (int i = 0; i < l / 2; i++) { char c1 = w.charAt(i), c2 = w.charAt(l - 1 - i); // Stmt (2) if (c1 != c2) { break; // Stmt (3) isPalin = true; // Stmt (4) } } return isPalin; }