Computer Science
Write a program to accept a sentence which may be terminated by either '.', '?' or '!' only. The words are to be separated by a single blank space and are in UPPER CASE.
Perform the following tasks:
- Check for the validity of the accepted sentence only for the terminating character.
- Arrange the words in ascending order of their length. If two or more words have the same length, then sort them alphabetically.
- Display the original sentence along with the converted sentence.
Test your program for the following data and some random data:
Example 1:
INPUT:
AS YOU SOW SO SHALL YOU REAP.
OUTPUT:
AS YOU SOW SO SHALL YOU REAP.
AS SO SOW YOU YOU REAP SHALL
Example 2:
INPUT:
SELF HELP IS THE BEST HELP.
OUTPUT:
SELF HELP IS THE BEST HELP.
IS THE BEST HELP HELP SELF
Example 3:
INPUT:
BE KIND TO OTHERS.
OUTPUT:
BE KIND TO OTHERS.
BE TO KIND OTHERS
Example 4:
INPUT:
NOTHING IS IMPOSSIBLE#
OUTPUT:
INVALID INPUT
Java
Java String Handling
ICSE Prac 2020
29 Likes
Answer
import java.util.*;
public class StringCheck
{
public static String sortString(String ipStr) {
StringTokenizer st = new StringTokenizer(ipStr);
int wordCount = st.countTokens();
String strArr[] = new String[wordCount];
for (int i = 0; i < wordCount; i++) {
strArr[i] = st.nextToken();
}
for (int i = 0; i < wordCount - 1; i++) {
for (int j = 0; j < wordCount - i - 1; j++) {
if (strArr[j].length() > strArr[j + 1].length()) {
String t = strArr[j];
strArr[j] = strArr[j+1];
strArr[j+1] = t;
}
if (strArr[j].length() == strArr[j + 1].length()
&&(strArr[j].compareTo(strArr[j+1]) > 0))
{
String t = strArr[j];
strArr[j] = strArr[j+1];
strArr[j+1] = t;
}
}
}
StringBuffer sb = new StringBuffer();
for (int i = 0; i < wordCount; i++) {
sb.append(strArr[i]);
sb.append(" ");
}
/*
* trim will remove the extra space added
* to the end of the string in the loop above
*/
return sb.toString().trim();
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
int len = str.length();
System.out.println();
if (str.charAt(len - 1) != '.'
&& str.charAt(len - 1) != '?'
&& str.charAt(len - 1) != '!') {
System.out.println("INVALID INPUT");
return;
}
/*
* str.substring(0, len - 1) removes the
* '.', '?', '!' at the end of the string
*/
String sortedStr = sortString(str.substring(0, len - 1));
System.out.println(str);
System.out.println(sortedStr);
}
}
Output
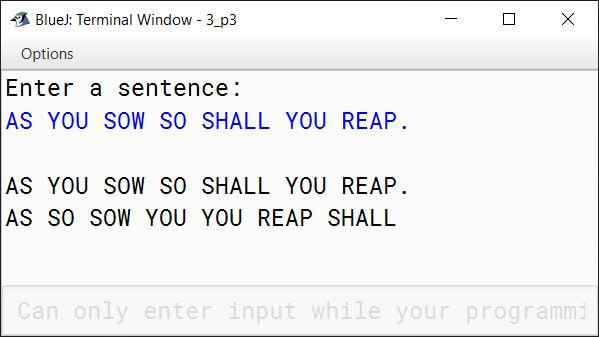
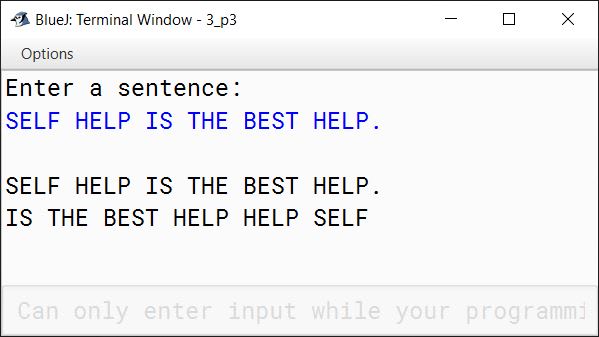
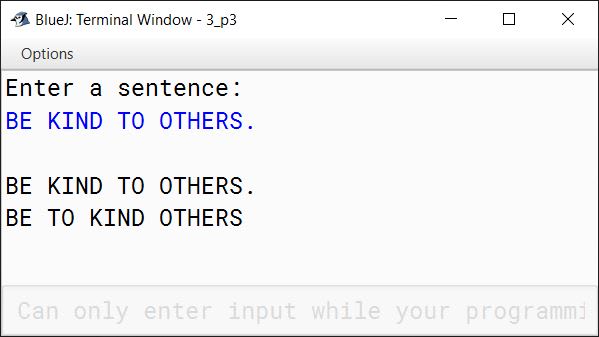
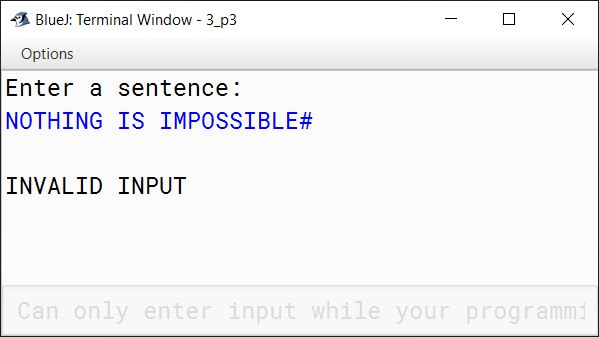
Answered By
8 Likes
Related Questions
Two strings,
city1
andcity2
, are compared usingcity1.compareTo(city2)
, and the result is less than zero. What does this indicate?Write a program in Java to enter any sentence. Also ask the user to enter a word. Print the number of times the word entered is present in the sentence. If the word is not present in the sentence, then print an appropriate message.
Write the output of the following String methods:
String x= "Galaxy", y= "Games";
(a) System.out.println(x.charAt(0)==y.charAt(0));
(b) System.out.println(x.compareTo(y));
A university student's registration number follows the format:
<CourseCode><Year><CollegeCode><RollNumber>
where
Component Description CourseCode A 3-letter code representing the course (e.g., CSE for Computer Science, ECE for Electronics & Communication) Year The last two digits of the admission year. CollegeCode A 3-digit code representing the college RollNumber A 4-digit unique student roll number. Examples
Registration Number Course Code Admission Year College Code Roll Number CSE240011023 CSE 24 001 1023 ECE252104297 ECE 25 210 4297 ASE230277259 ASE 23 027 7259 Define a class that accepts a student's registration number as input, extracts the relevant details, and displays them in the specified format.
import java.util.Scanner; public class KboatStuRegNum { public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Enter reg. no.: "); _______(1)_________ int l = n.length(); if (l != 12) { System.out.println("Invalid reg. no."); System.exit(0); } _______(2)_________ _______(3)_________ _______(4)_________ _______(5)_________ System.out.println("Course Code : " + cc); System.out.println("Admission Year : " + yr); System.out.println("College Code : " + cl); System.out.println("Roll Number : " + rNo); } }