Computer Science
Write a program to accept a date in the string format dd/mm/yyyy and accept the name of the day on 1st of January of the corresponding year. Find the day for the given date.
Example:
Input:
Date: 5/7/2001
Day on 1st January : MONDAY
Output:
Day on 5/7/2001 : THURSDAY
Run the program on the following inputs:
Input Date | Day on 1st January | Output day for |
---|---|---|
04/9/1998 | THURSDAY | FRIDAY |
31/8/1999 | FRIDAY | TUESDAY |
06/12/2000 | SATURDAY | WEDNESDAY |
The program should include the part for validating the inputs namely the date and day on 1st January of that year.
Java
Java Arrays
14 Likes
Answer
import java.util.*;
public class KboatDayName
{
public static void main(String args[]) {
int monthDays[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
String dayNames[] = {"MONDAY", "TUESDAY", "WEDNESDAY", "THURSDAY",
"FRIDAY", "SATURDAY", "SUNDAY"};
Scanner in = new Scanner(System.in);
System.out.print("Enter Date(dd/mm/yyyy): ");
String dateStr = in.nextLine();
StringTokenizer st = new StringTokenizer(dateStr, "/");
int tokenCount = st.countTokens();
if (tokenCount <= 0 || tokenCount > 3) {
System.out.println("Invalid Date");
return;
}
int day = Integer.parseInt(st.nextToken());
int month = Integer.parseInt(st.nextToken());
int year = Integer.parseInt(st.nextToken());
boolean leapYear = isLeapYear(year);
if (leapYear) {
monthDays[1] = 29;
}
if (month < 1 || month > 12) {
System.out.println("Invalid Month");
return;
}
if (day < 1 || day > monthDays[month - 1]) {
System.out.println("Invalid Day");
return;
}
System.out.print("Day on 1st January: ");
String startDayName = in.nextLine();
int startDayIdx = -1;
for (int i = 0; i < dayNames.length; i++) {
if (dayNames[i].equalsIgnoreCase(startDayName)) {
startDayIdx = i;
break;
}
}
if (startDayIdx == -1) {
System.out.println("Invalid Day Name");
return;
}
//Calculate total days
int tDays = 0;
for (int i = 0; i < month - 1; i++) {
tDays += monthDays[i];
}
tDays += day;
int currDayIdx = tDays % 7 + startDayIdx - 1;
if (currDayIdx >= 7) {
currDayIdx -= 7;
}
System.out.println("Day on " + dateStr + " : " + dayNames[currDayIdx]);
}
public static boolean isLeapYear(int y) {
boolean ret = false;
if (y % 400 == 0) {
ret = true;
}
else if (y % 100 == 0) {
ret = false;
}
else if (y % 4 == 0) {
ret = true;
}
else {
ret = false;
}
return ret;
}
}
Output
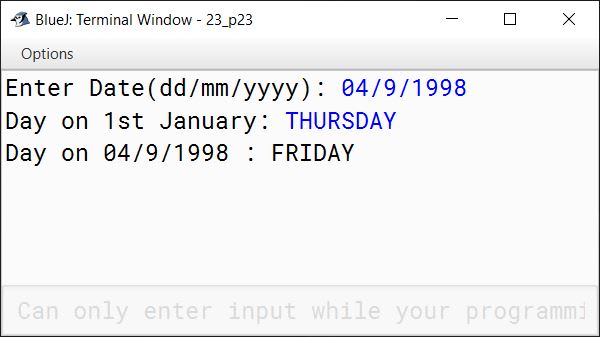
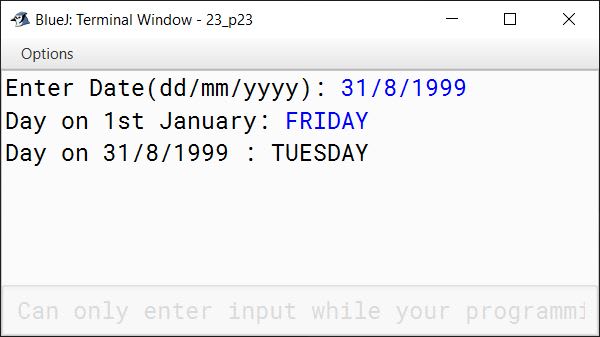
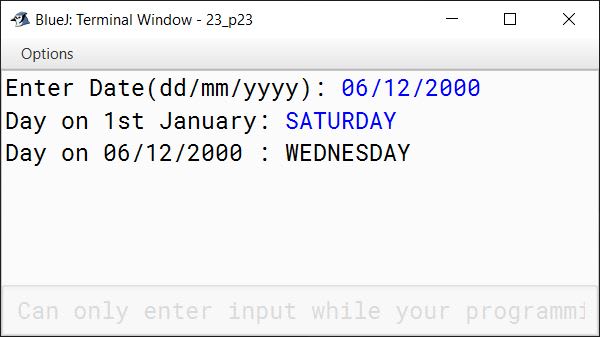
Answered By
3 Likes
Related Questions
A wondrous square is an n by n grid which fulfils the following conditions:
- It contains integers from 1 to n2, where each integer appears only once.
- The sum of integers in any row or column must add up to 0.5 x n x (n2 + 1).
For example, the following grid is a wondrous square where the sum of each row or column is 65 when n=5.
17 24 1 8 15 23 5 7 14 16 4 6 13 20 22 10 12 19 21 3 11 18 25 2 9 Write a program to read n (2 <= n <= 10) and the values stored in these n by n cells and output if the grid represents a wondrous square.
Also output all the prime numbers in the grid along with their row index and column index as shown in the output. A natural number is said to be prime if it has exactly two divisors. For example, 2, 3, 5, 7, 11 The first element of the given grid i.e. 17 is stored at row index 0 and column index 0 and the next element in the row i.e. 24 is stored at row index 0 and column index 1.
Test your program for the following data and some random data:
Input:
n = 416 15 1 2 6 4 10 14 9 8 12 5 3 7 11 13 Output:
Yes, it represents a wondrous squarePrime Row Index Column Index 2 0 3 3 3 0 5 2 3 7 3 1 11 3 2 13 3 3 15 0 1 Input:
n = 31 2 4 3 7 5 8 9 6 Output:
Not a wondrous squarePrime Row Index Column Index 2 0 1 3 1 0 5 1 2 7 1 1 Input:
n = 22 3 3 2 Output: Not a wondrous square
Prime Row Index Column Index 2 0 0 2 1 1 3 0 1 3 1 0 The manager of a company wants to analyze the machine usage from the records to find the utilization of the machine. He wants to know how long each user used the machine. When the user wants to use the machine, he must login to the machine and after finishing the work, he must logoff the machine.
Each log record consists of:
User Identification number
Login time and date
Logout time and dateTime consists of:
Hours
MinutesDate consists of:
Day
MonthYou may assume all logins and logouts are in the same year and there are 100 users at the most. The time format is 24 hours.
Design a program:
(a) To find the duration for which each user logged. Output all records along with the duration in hours (format hours: minutes).
(b) Output the record of the user who logged for the longest duration. You may assume that no user will login for more than 48 hours.
Test your program for the following data and some random data.
Sample Data
Input:
Number of users: 3
User IdentificationLogin Time and Date Logout Time and Date 20:10 20-12 2:50 21-12 12:30 20-12 12:30 21-12 16:20 20-12 16:30 20-12 Output:
User Identification Login Time and Date Logout Time and Date Duration
Hours:Minutes149 20:10 20-12 2:50 21-12 6:40 173 12:30 20-12 12:30 21-12 24:00 142 16:20 20-12 16:30 20-12 00:10 The user who logged in for longest duration:
173 12:30 20-12 12:30 21-12 24:00 Write a program to input two valid dates, each comprising of Day (2 digits), Month (2 digits) and Year (4 digits) and calculate the days elapsed between both the dates.
Test your program for the following data values:
(a)
FIRST DATE:
Day: 24
Month: 09
Year: 1960SECOND DATE:
Day: 08
Month: 12
Year: 1852Output: xxxxxxxx
(these are actual number of days elapsed)(b)
FIRST DATE:
Day: 10
Month: 01
Year: 1952SECOND DATE:
Day: 16
Month: 10
Year: 1952Output: xxxxxxxx
(these are actual number of days elapsed)Numbers have different representations depending on the bases on which they are expressed. For example, in base 3, the number 12 is written as 110 (1 x 32 + 1 x 31 + 0 x 30) but base 8 it is written as 14 (1 x 81 + 4 x 80).
Consider for example, the integers 12 and 5. Certainly these are not equal if base 10 is used for each. But suppose, 12 was a base 3 number and 5 was a base 6 number then, 12 base 3 = 1 x 31 + 2 x 30, or 5 base 6 or base 10 (5 in any base is equal to 5 base 10). So, 12 and 5 can be equal if you select the right bases for each of them.
Write a program to input two integers x and y and calculate the smallest base for x and smallest base for y (likely different from x) so that x and y represent the same value. The base associated with x and y will be between 1 and 20 (both inclusive). In representing these numbers, the digits 0 to 9 have their usual decimal interpretations. The upper case letters from A to J represent digits 10 to 19 respectively.
Test your program for the following data and some random data.
Sample Data
Input:
x=12, y=5Output:
12 (base 3)=5 (base 6)Input:
x=10, y=AOutput:
10 (base 10)=A (base 11)Input:
x=12, y=34Output:
~~12 (base 8) = 34 (base 2)~~
12 (base 17) = 34 (base 5)
[∵ 34 (base 2) is not valid as only 0 & 1 are allowed in base 2]Input:
x=123, y=456Output:
123 is not equal to 456 in any base between 2 to 20Input:
x=42, y=36Output:
42 (base 7) = 36 (base 8)