Computer Applications
Write a program that reads a 4 x 5 two dimensional array and then prints the column sums of the array along with the array printed in matrix form.
Java
Java Arrays
7 Likes
Answer
import java.util.Scanner;
public class KboatDDAColSum
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[][] = new int[4][5];
System.out.println("Enter the 2D array:");
for (int i = 0; i < 4; i++) {
System.out.println("Enter elements of row " + (i + 1));
for (int j = 0; j < 5; j++) {
arr[i][j] = in.nextInt();
}
}
System.out.println("Input array :");
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 5; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
//Compute column-wise sum
for (int i = 0; i < 5; i++) {
int cSum = 0;
for (int j = 0; j < 4; j++) {
cSum += arr[j][i];
}
System.out.println("Column " + (i + 1)
+ " sum = " + cSum);
}
}
}
Variable Description Table
Program Explanation
Output
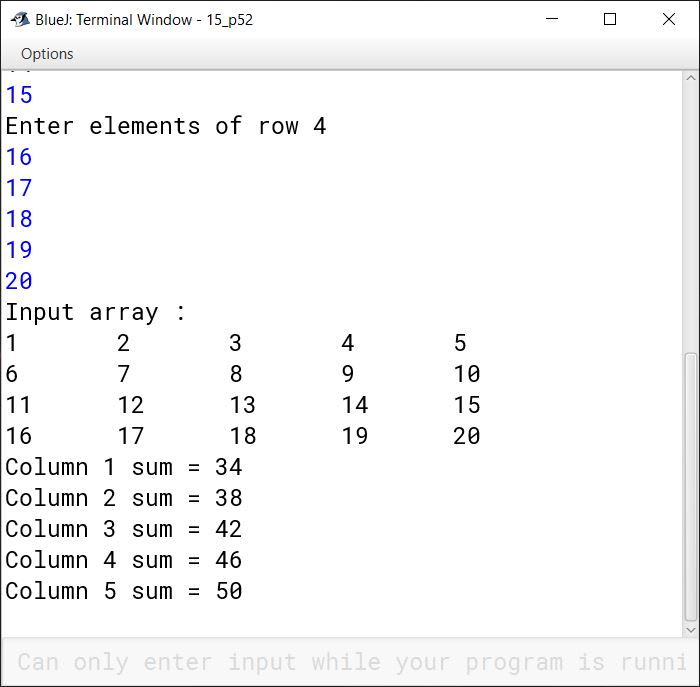
Answered By
2 Likes
Related Questions
Write a program to input integer elements into an array of size 20 and perform the following operations:
- Display largest number from the array
- Display smallest number from the array
- Display sum of all the elements of the array
For the same array mentioned above in previous question, write a program to sort the above array using bubble sort technique. Give the array-status after every iteration.
Write a program to input forty words in an array. Arrange these words in descending order of alphabets, using selection sort technique. Print the sorted array.
Write a program that inputs a 2D array and displays how the array elements will look in row major form and column major form.