Computer Applications
Write a program that inputs a number and tests if the given number is a multiple of both 3 and 5.
Java
Java Conditional Stmts
9 Likes
Answer
import java.util.Scanner;
public class KboatMultipleCheck
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number: ");
int num = in.nextInt();
if (num % 3 == 0 && num % 5 == 0)
System.out.println("Multiple of 3 and 5");
else
System.out.println("Not a multiple of 3 and 5");
}
}
Variable Description Table
Program Explanation
Output
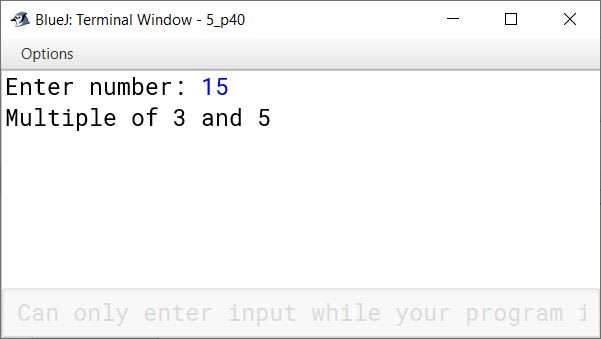
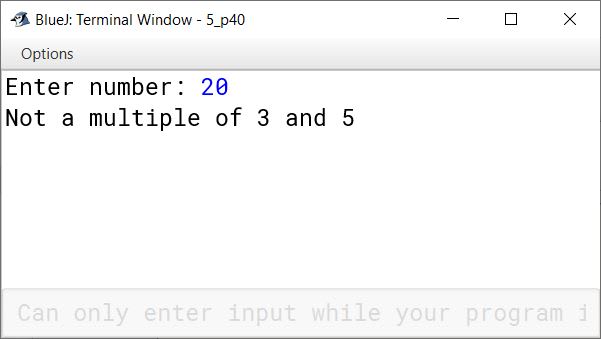
Answered By
4 Likes
Related Questions
Find the error:
class Test { public static void main (String args[]) { int x = 10; if(x == 10) { int y = 20; System.out.println("x and y: "+ x +" "+ y); x = y * 2; } y = 100; System.out.println("x is"+ x); } }
Find the error :
x = 3; y = 4; z = math.power(x*x, y/2);
Write a program that inputs a character and prints if the typed character is in uppercase or lowercase.
Write a program that inputs a character and prints if the user has typed a digit or an alphabet or a special character.