Computer Applications
Write a program in Java to store 20 numbers (even and odd numbers) in a Single Dimensional Array (SDA). Calculate and display the sum of all even numbers and all odd numbers separately.
Java
Java Arrays
317 Likes
Answer
import java.util.Scanner;
public class KboatSDAOddEvenSum
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[] = new int[20];
System.out.println("Enter 20 numbers");
for (int i = 0; i < arr.length; i++) {
arr[i] = in.nextInt();
}
int oddSum = 0, evenSum = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i] % 2 == 0)
evenSum += arr[i];
else
oddSum += arr[i];
}
System.out.println("Sum of Odd numbers = " + oddSum);
System.out.println("Sum of Even numbers = " + evenSum);
}
}
Variable Description Table
Program Explanation
Output
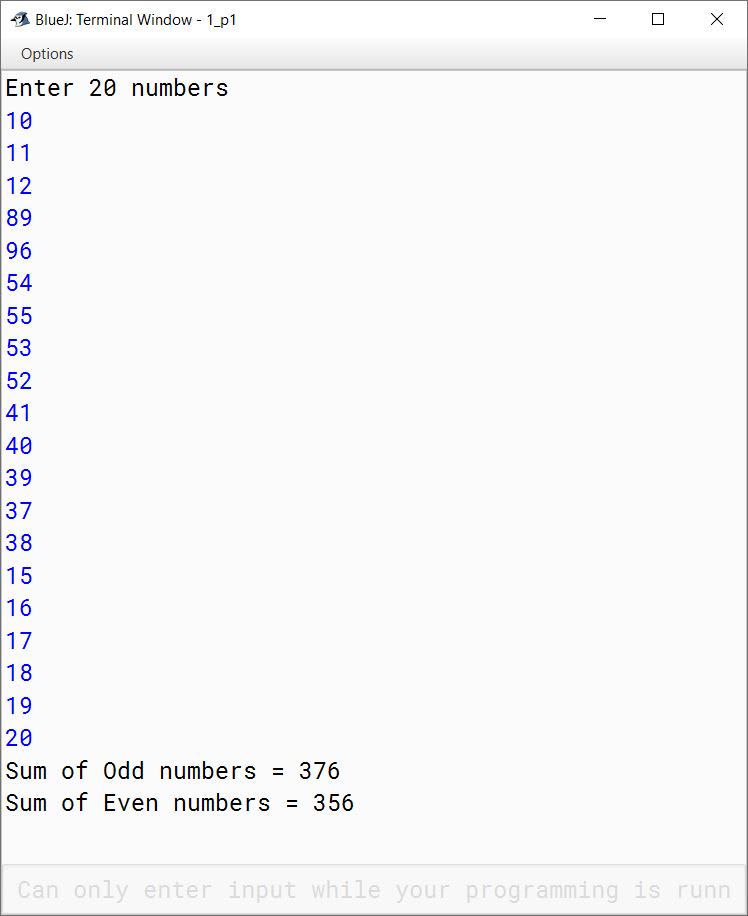
Answered By
141 Likes
Related Questions
Write a program in Java to store 10 numbers (including positive and negative numbers) in a Single Dimensional Array (SDA). Display all the negative numbers followed by the positive numbers without changing the order of the numbers.
Sample Input:n[0] n[1] n[2] n[3] n[4] n[5] n[6] n[7] n[8] n[9] 15 21 -32 -41 54 61 71 -19 -44 52 Sample Output: -32, -41, -19, 44, 15, 21, 54, 61, 71, 52
Write a program in Java to store 20 numbers in a Single Dimensional Array (SDA). Display the numbers which are prime.
Sample Input:n[0] n[1] n[2] n[3] n[4] n[5] … n[16] n[17] n[18] n[19] 45 65 77 71 90 67 … 82 19 31 52 Sample Output: 71, 67, 19, 31
Write a program in Java to store 20 temperatures in °F in a Single Dimensional Array (SDA) and display all the temperatures after converting them into °C.
Hint: (c/5) = (f - 32) / 9Write a program to accept name and total marks of N number of students in two single subscript arrays name[ ] and totalmarks[ ].
Calculate and print:
(i) The average of the total marks obtained by N number of students.
[average = (sum of total marks of all the students)/N]
(ii) Deviation of each student's total marks with the average.
[deviation = total marks of a student - average]