Computer Applications
Write a program in Java to read three integers and display them in descending order.
Java
Java Conditional Stmts
18 Likes
Answer
import java.util.Scanner;
public class KboatDescending
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter first number: ");
int a = in.nextInt();
System.out.print("Enter second number: ");
int b = in.nextInt();
System.out.print("Enter third number: ");
int c = in.nextInt();
int max = -1, mid = -1, min = -1;
if (a > b) {
if (a > c) {
max = a;
if (b > c) {
mid = b;
min = c;
}
else {
mid = c;
min = b;
}
}
else {
max = c;
mid = a;
min = b;
}
}
else {
if (b > c) {
max = b;
if (a > c) {
mid = a;
min = c;
}
else {
{
mid = c;
min = a;
}
}
}
else {
max = c;
mid = b;
min = a;
}
}
System.out.println("Numbers in Descending order:");
System.out.println(max + " " + mid + " " + min);
}
}
Output
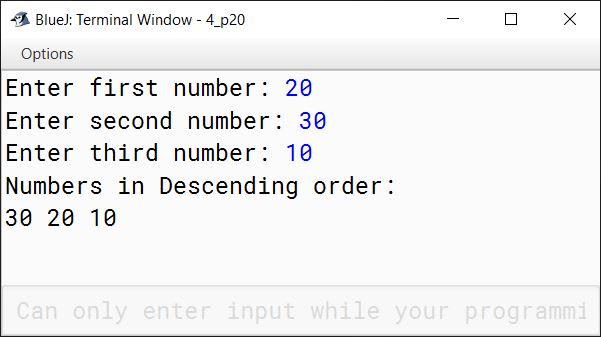
Answered By
6 Likes
Related Questions
Write a program in Java that reads a word and checks whether it begins with a vowel or not.
Write a program that reads a month number and displays it in words.
Using the ternary operator, create a program to find the largest of three numbers.
Create a program to find out if the number entered by the user is a two, three or four digits number.
Sample input: 1023
Sample output: 1023 is a 4 digit number.