Computer Applications
Write a program in java to input any two variables through constructor parameters and swap and print their values.
Java
Java Constructors
1 Like
Answer
import java.util.Scanner;
public class KboatSwap {
int a;
int b;
public KboatSwap(int x, int y) {
a = x;
b = y;
}
public void swap() {
int t = a;
a = b;
b = t;
}
public void display() {
System.out.println("a=" + a);
System.out.println("b=" + b);
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("Enter first number: ");
int p = in.nextInt();
System.out.print("Enter second number: ");
int q = in.nextInt();
KboatSwap obj = new KboatSwap(p, q);
System.out.println("Values before swap:");
obj.display();
obj.swap();
System.out.println("Values after swap:");
obj.display();
}
}
Output
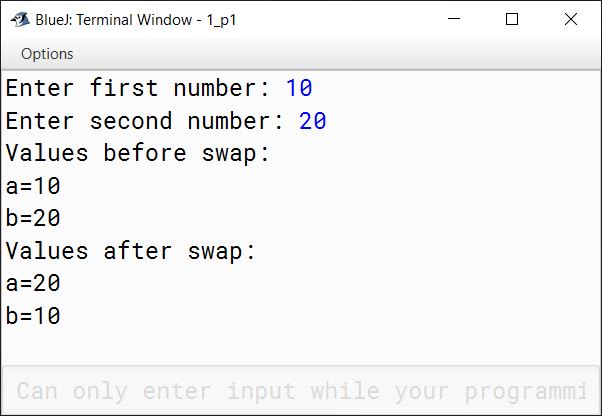
Answered By
2 Likes
Related Questions
Fill in the blanks:
The _________ refers to the current object.
Consider the given program and answer the questions given below:
class temp { int a; temp() { a=10; } temp(int z) { a=z; } void print() { System.out.println(a); } void main() { temp t = new temp(); temp x = new temp(30); t.print(); x.print(); } }
(a) What concept of OOPs is depicted in the above program with two constructors?
(b) What is the output of the method main()?
Define a class named FruitJuice with the following description:
Data Members Purpose int product_code stores the product code number String flavour stores the flavour of the juice (e.g., orange, apple, etc.) String pack_type stores the type of packaging (e.g., tera-pack, PET bottle, etc.) int pack_size stores package size (e.g., 200 mL, 400 mL, etc.) int product_price stores the price of the product Member Methods Purpose FruitJuice() constructor to initialize integer data members to 0 and string data members to "" void input() to input and store the product code, flavour, pack type, pack size and product price void discount() to reduce the product price by 10 void display() to display the product code, flavour, pack type, pack size and product price Fill in the blanks:
A _________ constructor creates objects by passing value to it.