Computer Applications
Write a program in Java to find the sum of the following series:
S = (2/a) + (3/a2) + (5/a3) + (7/a4) + ……. to n
Java
Java Nested for Loops
25 Likes
Answer
import java.util.Scanner;
public class KboatSeries
{
public void computeSum() {
Scanner in = new Scanner(System.in);
System.out.print("Enter a: ");
int a = in.nextInt();
System.out.print("Enter n: ");
int n = in.nextInt();
double sum = 0;
int lastPrime = 1;
for (int i = 1; i <= n; i++) {
for (int j = lastPrime + 1; j <= Integer.MAX_VALUE; j++) {
boolean isPrime = true;
for (int k = 2; k <= j / 2; k++) {
if (j % k == 0) {
isPrime = false;
break;
}
}
if (isPrime) {
sum += j / Math.pow(a, i);
lastPrime = j;
break;
}
}
}
System.out.println("Sum=" + sum);
}
}
Variable Description Table
Program Explanation
Output
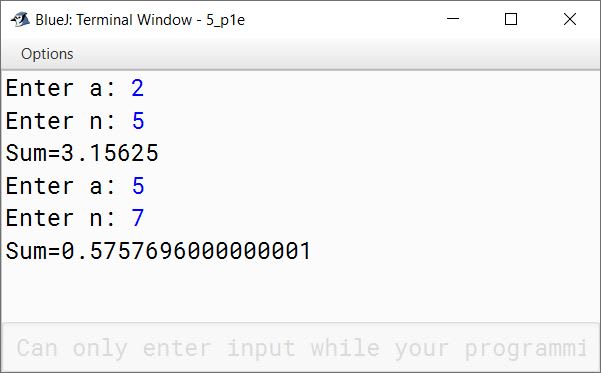
Answered By
11 Likes
Related Questions
Write a program in Java to find the sum of the following series:
S = (a/2!) - (a/3!) + (a/4!) - (a/5!) + ……. + (a/10!)
Write a program in Java to find the sum of the following series:
S = a - (a/2!) + (a/3!) - (a/4!) + ……. to n
Write a program to input two numbers and check whether they are twin prime numbers or not.
Hint: Twin prime numbers are the prime numbers whose difference is 2.
For example: (5,7), (11,13), ……. and so on.Write a program to display all the numbers between 100 and 200 which don't contain zeros at any position.
For example: 111, 112, 113, ……. , 199