Computer Applications
Write a program in Java to find the Fibonacci series within a range entered by the user.
Sample Input:
Enter the minimum value: 10
Enter the maximum value: 20
Sample Output:
13
Java
Java Iterative Stmts
8 Likes
Answer
import java.util.Scanner;
public class KboatFibonacciRange
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the minimum value: ");
int m = in.nextInt();
System.out.print("Enter the maximum value: ");
int n = in.nextInt();
int a = 0, b = 1;
while (a <= n) {
if (a >= m)
System.out.println(a);
int c = a + b;
a = b;
b = c;
}
}
}
Output
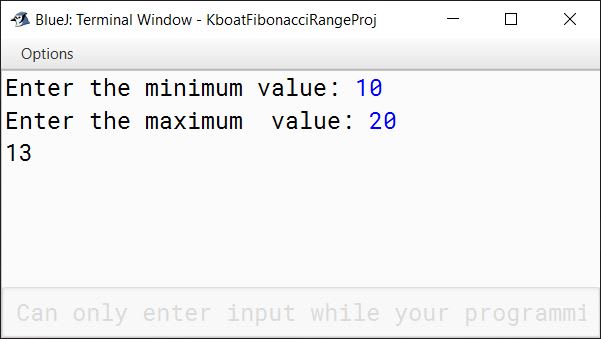
Answered By
2 Likes