Computer Applications
Write a program in Java to enter any sentence. Also ask the user to enter a word. Print the number of times the word entered is present in the sentence. If the word is not present in the sentence, then print an appropriate message.
Java
Java String Handling
12 Likes
Answer
import java.util.Scanner;
public class KboatWordFrequency
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
System.out.println("Enter a word:");
String ipWord = in.nextLine();
str += " ";
String word = "";
int count = 0;
int len = str.length();
for (int i = 0; i < len; i++) {
if (str.charAt(i) == ' ') {
if (word.equalsIgnoreCase(ipWord))
count++ ;
word = "";
}
else {
word += str.charAt(i);
}
}
if (count > 0) {
System.out.println(ipWord + " is present " + count + " times.");
}
else {
System.out.println(ipWord + " is not present in sentence.");
}
}
}
Output
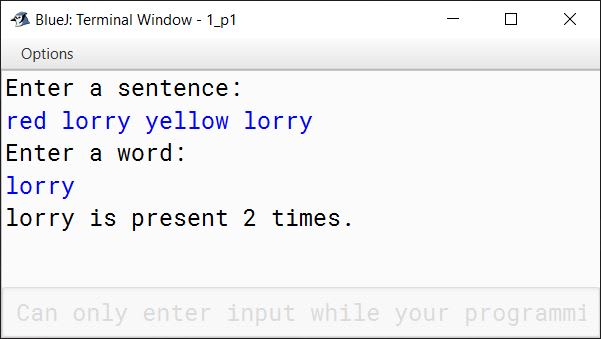
Answered By
7 Likes
Related Questions
The output of the statement "talent".compareTo("genius") is:
- 11
- –11
- 0
- 13
Define a class to accept the gmail id and check for its validity.
A gmail id is valid only if it has:
→ @
→ .(dot)
→ gmail
→ com
Example:
icse2024@gmail.com
is a valid gmail idThe following code to compare two strings is compiled, the following syntax error was displayed – incompatible types – int cannot be converted to boolean.
Identify the statement which has the error and write the correct statement. Give the output of the program segment.
void calculate() { String a = "KING", b = "KINGDOM"; boolean x = a.compareTo(b); System.out.println(x); }
Two strings,
city1
andcity2
, are compared usingcity1.compareTo(city2)
, and the result is less than zero. What does this indicate?