Computer Applications
Write a program in Java to calculate the monthly electricity bill of a consumer according to the units consumed. The tariff is given below:
Units Consumed | Charge |
---|---|
Upto 100 units | ₹1.25 per unit |
For next 100 units | ₹1.50 per unit |
More than 200 units | ₹1.80 per unit |
Unit consumed = Present reading - Previous reading
Use a function named cal(int u) and print the information in the main function as per the given format:
Consumer No. Name Units Consumed Amount
xxx xxx xxx xxx
Java
Java Conditional Stmts
58 Likes
Answer
import java.util.Scanner;
public class KboatBill
{
public double cal(int u) {
double amt = 0;
if (u <= 100) {
amt = 1.25 * u;
}
else if (u <= 200) {
amt = 1.25 * 100 + (u - 100) * 1.5;
}
else {
amt = 1.25 * 100 + 1.5 * 100 + (u - 200) * 1.8;
}
return amt;
}
public static void main(String args[]) {
KboatBill obj = new KboatBill();
Scanner in = new Scanner(System.in);
System.out.print("Enter Name: ");
String name = in.nextLine();
System.out.print("Enter Consumer No.: ");
int cNo = in.nextInt();
System.out.print("Enter present reading: ");
int curr = in.nextInt();
System.out.print("Enter previous reading: ");
int prev = in.nextInt();
int used = curr - prev;
double amount = obj.cal(used);
System.out.println("Consumer No.\tName\tUnits Consumed\tAmount");
System.out.println(cNo + "\t\t" + name + "\t" + used + "\t" + amount);
}
}
Output
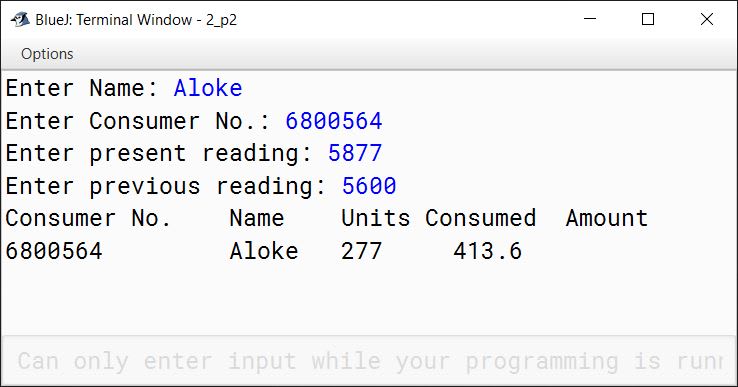
Answered By
19 Likes