Computer Applications
Write a program in Java to accept a number. Check and print whether it is a prime number or not.
A prime number is a number which is divisible by 1 and itself only. For example 2, 3, 5, 7, 11, 13 are all prime numbers.
Java
Java Iterative Stmts
171 Likes
Answer
import java.util.Scanner;
public class KboatPrimeCheck
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number: ");
int n = in.nextInt();
int c = 0;
for (int i = 1; i <= n; i++) {
if (n % i == 0) {
c++;
}
}
if (c == 2) {
System.out.println(n + " is a prime number");
}
else {
System.out.println(n + " is not a prime number");
}
}
}
Output
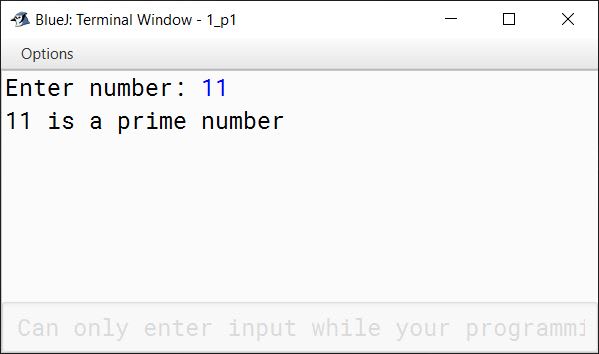
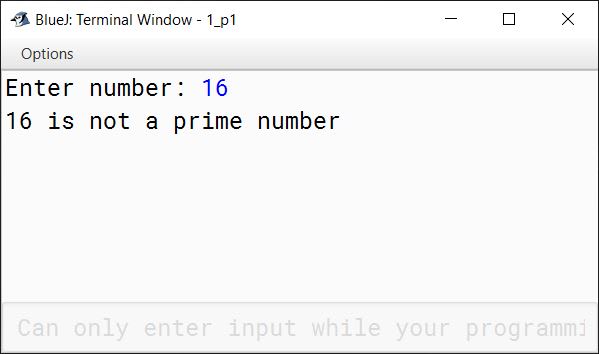
Answered By
71 Likes
Related Questions
Define a class to accept a number and check whether it is a SUPERSPY number or not. A number is called SUPERSPY if the sum of the digits equals the number of the digits.
Example1:
Input: 1021 output: SUPERSPY number [SUM OF THE DIGITS = 1+0+2+1 = 4, NUMBER OF DIGITS = 4 ]
Example2:
Input: 125 output: Not an SUPERSPY number [1+2+5 is not equal to 3]
Define a class to accept a four digit number and check whether it is a Framul Number or not. A number is called a Framul Number if the product of all its digits equals fives times the sum of its first and last digit.
Example 1:
Input: 1325
Product of all digits = 1 x 3 x 2 x 5 = 30
Five times sum of first & last digit = 5 x (5 + 1) = 5 x 6 = 30Output: Framul Number
Example 2:
Input: 2981
Product of all digits = 2 x 9 x 8 x 1 = 144
Five times sum of first & last digit = 5 x (1 + 2) = 5 x 3 = 15Output: Not a Framul Number
If the entered number is not of four digits, the message "Please enter a four digit number" should be shown.
import java.util.Scanner; public class KboatFramulNumber { public static void main(String args[]) { Scanner sc = _____(1)_____ System.out.print("Enter a four-digit number: "); int num = _____(2)_____ _______(3)_______ { System.out.println("Please enter a four-digit number"); return; } int d = 0; int f = num % 10; int p = 1; _______(4)_______ { _____(5)_____ _____(6)_____ _____(7)_____ } int s = _____(8)_____ if (p == s) { _______(9)_______ } else { _______(10)_______ } } }
To execute a loop 5 times, which of the following is correct?
How many times will the following loop execute? Write the output of the code:
int a = 5; while (a > 0) { System.out.println(a-- + 2); if (a % 3 == 0) break; }