Computer Applications
Write a program in Java that takes input using the Scanner class to calculate the Simple Interest and the Compound Interest with the given values:
i. Principle Amount = Rs.1,00,000
ii. Rate = 11.5%
iii. Time = 5 years
Display the following output:
i. Simple interest
ii. Compound interest
iii. Absolute value of the difference between the simple and compound interest.
Java
Input in Java
33 Likes
Answer
import java.util.Scanner;
public class KboatInterest
{
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Principal: ");
double p = in.nextDouble();
System.out.print("Enter Rate: ");
double r = in.nextDouble();
System.out.print("Enter Time: ");
int t = in.nextInt();
double si = p * r * t / 100.0;
double cAmt = (p * Math.pow(1 + (r / 100), t));
double ci = cAmt - p;
double diff = Math.abs(si - ci);
System.out.println("Simple interest = " + si);
System.out.println("Compound interest = " + ci);
System.out.println("Absolute Difference = " + diff);
in.close();
}
}
Output
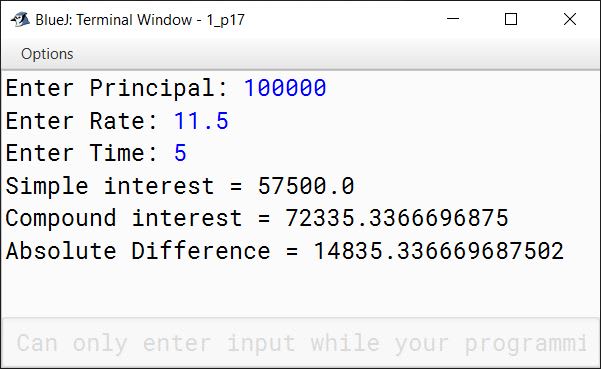
Answered By
15 Likes
Related Questions
Write a program that takes the distance of the commute in kilometres, the car fuel consumption rate in kilometre per gallon, and the price of a gallon of petrol as input. The program should then display the cost of the commute.
Write a statement to let the user enter an integer or a double value from the keyboard.
Write a program to compute the Time Period (T) of a Simple Pendulum as per the following formula:
T = 2π√(L/g)
Input the value of L (Length of Pendulum) and g (gravity) using the Scanner class.
Write a code that creates a Scanner object and sets its delimiter to the dollar sign.