Java Series Programs
Write a menu driven program to perform the following tasks by using Switch case statement:
(a) To print the series:
0, 3, 8, 15, 24, ………… to n terms. (value of 'n' is to be an input by the user)
(b) To find the sum of the series:
S = (1/2) + (3/4) + (5/6) + (7/8) + ……….. + (19/20)
Java
Java Iterative Stmts
ICSE 2011
174 Likes
Answer
import java.util.Scanner;
public class KboatSeriesMenu
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type 1 to print series");
System.out.println("0, 3, 8, 15, 24,....to n terms");
System.out.println();
System.out.println("Type 2 to find sum of series");
System.out.println("(1/2) + (3/4) + (5/6) + (7/8) +....+ (19/20)");
System.out.println();
System.out.print("Enter your choice: ");
int choice = in.nextInt();
switch (choice) {
case 1:
System.out.print("Enter n: ");
int n = in.nextInt();
for (int i = 1; i <= n; i++)
System.out.print(((i * i) - 1) + " ");
System.out.println();
break;
case 2:
double sum = 0;
for (int i = 1; i <= 19; i = i + 2)
sum += i / (double)(i + 1);
System.out.println("Sum = " + sum);
break;
default:
System.out.println("Incorrect Choice");
break;
}
}
}
Variable Description Table
Program Explanation
Output
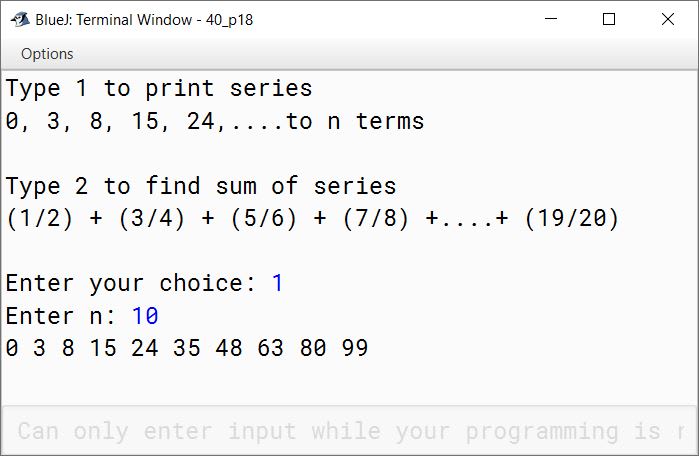
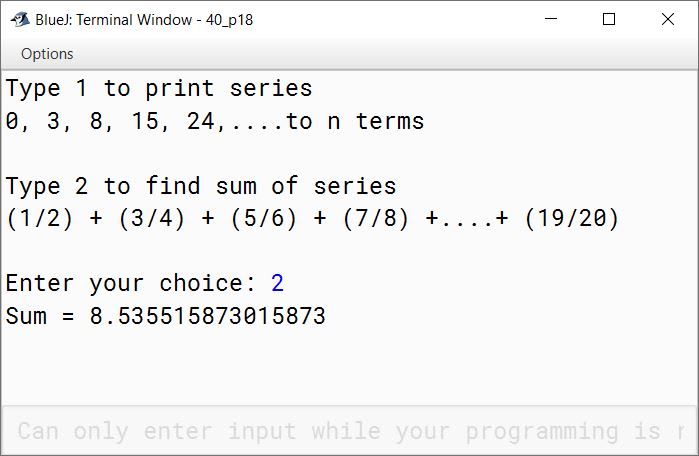
Answered By
51 Likes