Computer Applications
Write a Java program to print name, purchase amount and final payable amount after discount as per given table:
Purchase Amount | Discount |
---|---|
upto ₹10000/- | 15% |
₹10000 to ₹ 20000/- | 20% |
Above ₹20000/- | 30% |
Java
Java Conditional Stmts
38 Likes
Answer
import java.util.Scanner;
public class KboatDiscount
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Name: ");
String name = in.nextLine();
System.out.print("Enter Purchase Amount: ");
double amt = in.nextInt();
int d = 0;
if (amt <= 10000)
d = 15;
else if (amt <= 20000)
d = 20;
else
d = 30;
double discAmt = amt * d / 100.0;
double finalAmt = amt - discAmt;
System.out.println("Name: " + name);
System.out.println("Purchase Amount: " + amt);
System.out.println("Final Payable Amount: " + finalAmt);
}
}
Output
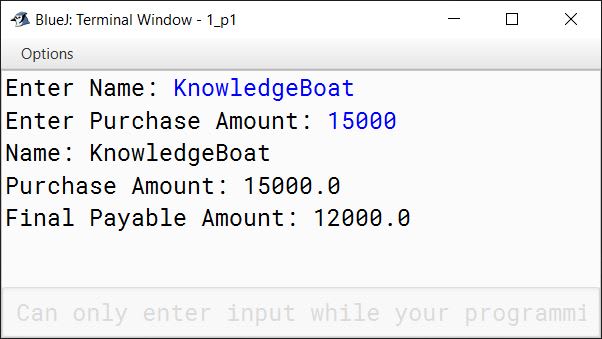
Answered By
12 Likes
Related Questions
The statement that brings the control back to the calling method is:
- break
- System.exit(0)
- continue
- return
A student executes the following program segment and gets an error. Identify the statement which has an error, correct the same to get the output as WIN.
boolean x = true; switch(x) { case 1: System.out.println("WIN"); break; case 2: System.out.println("LOOSE"); }
Rewrite the following code using single if statement.
if(code=='g') System.out.println("GREEN"); else if(code=='G') System.out.println("GREEN");
An air-conditioned bus charges fare from the passengers based on the distance travelled as per the tariff given below:
Distance Travelled Fare Up to 10 km Fixed charge ₹80 11 km to 20 km ₹6/km 21 km to 30 km ₹5/km 31 km and above ₹4/km Design a program to input distance travelled by the passenger. Calculate and display the fare to be paid.