Computer Applications
Write a java program to accept an integer. From it create a new integer by removing all zeros from it. For example, for the integer 20180 the new integer after removing all zeros from it will be 218. Print the original as well as the new integer.
Java
Java Iterative Stmts
29 Likes
Answer
import java.util.Scanner;
public class Kboat
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number: ");
int num = in.nextInt();
int idx = 0;
int newNum = 0;
int n = num;
while (n != 0) {
int d = n % 10;
if (d != 0) {
newNum += (int)(d * Math.pow(10, idx));
idx++;
}
n /= 10;
}
System.out.println("Original number = " + num);
System.out.println("New number = " + newNum);
}
}
Output
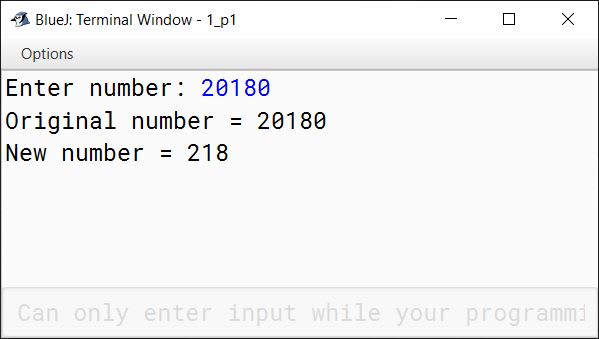
Answered By
10 Likes