Computer Applications
Java Calculator Program: Write a calculator program in Java that takes as input two numbers and a mathematical operator to perform mathematical operation and prints the calculated result. Based on the operator entered, perform the calculation, that is, '+' for addition, '-' for subtraction, '*' for product and '/' for division. For operators other than these, print the message "INVALID OPERATOR". (Make use of switch-case construct).
Java
Java Conditional Stmts
30 Likes
Answer
import java.util.Scanner;
public class KboatCalculator
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter first number: ");
int a = in.nextInt();
System.out.println("Enter second number: ");
int b = in.nextInt();
System.out.println("Enter operator: ");
char op = in.next().charAt(0);
int r = 0;
switch (op) {
case '+':
r = a + b;
System.out.println(a + " + " + b + " = " + r);
break;
case '-':
r = a - b;
System.out.println(a + " - " + b + " = " + r);
break;
case '*':
r = a * b;
System.out.println(a + " * " + b + " = " + r);
break;
case '/':
r = a / b;
System.out.println(a + " / " + b + " = " + r);
break;
default:
System.out.println("INVALID OPERATOR");
}
}
}
Output
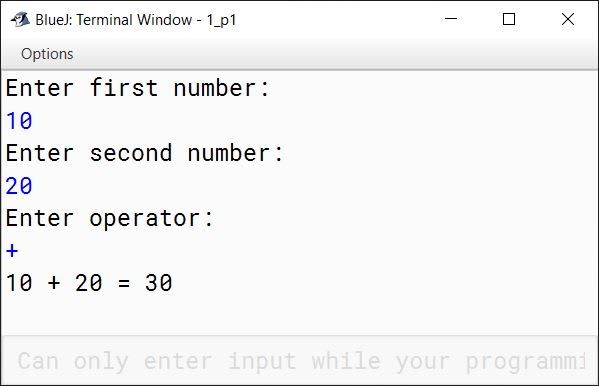
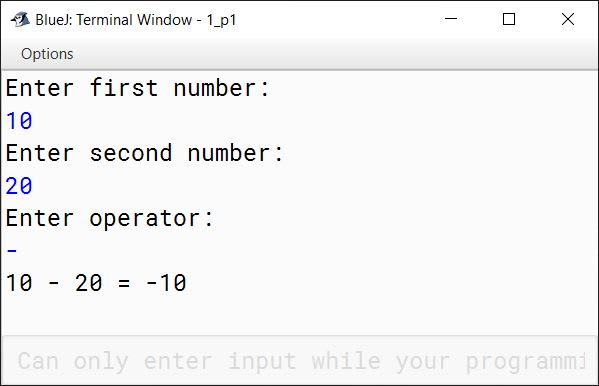
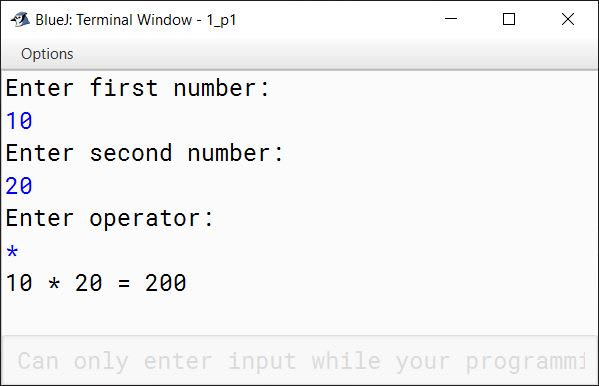
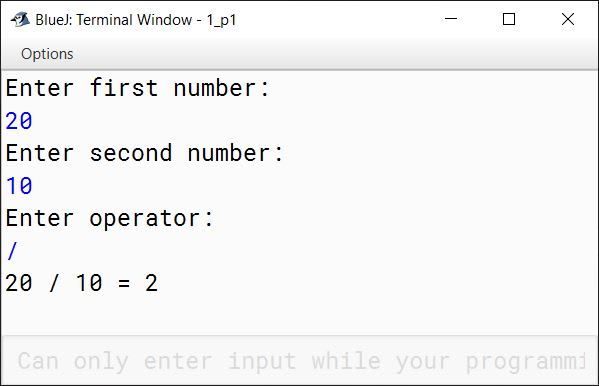
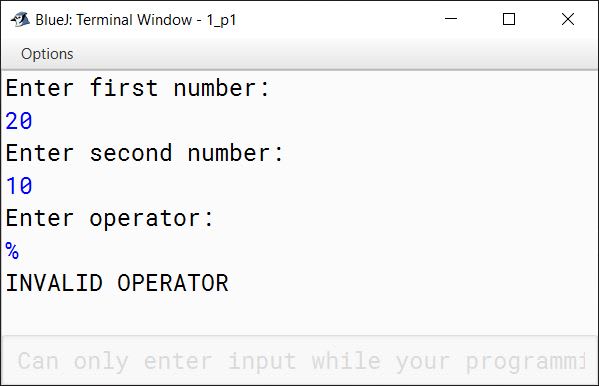
Answered By
11 Likes