Computer Applications
Using the switch statement, write a menu driven program to print the following patterns based on user's choice:
Pattern 1:
11
12 22
13 23 33
14 24 34 44
15 25 35 45 55
Pattern 2:
1
0 1
1 0 1
0 1 0 1
1 0 1 0 1
Java
Java Nested for Loops
5 Likes
Answer
import java.util.Scanner;
public class KboatPattern
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter 1 for Pattern 1");
System.out.println("Enter 2 for Pattern 3");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
switch(ch) {
case 1:
for (int i = 11, j = 1; j <= 5; i++, j++) {
int t = i;
for (int k = 1; k <= j; k++) {
System.out.print(t + " ");
t += 10;
}
System.out.println();
}
break;
case 2:
for (int i = 1; i <= 5; i++) {
int t;
if (i % 2 == 0)
t = 0;
else
t = 1;
for (int j = 1; j <= i; j++) {
System.out.print(t + " ");
if (t == 1)
t = 0;
else
t = 1;
}
System.out.println();
}
break;
default:
System.out.println("Wrong Choice");
break;
}
}
}
Output
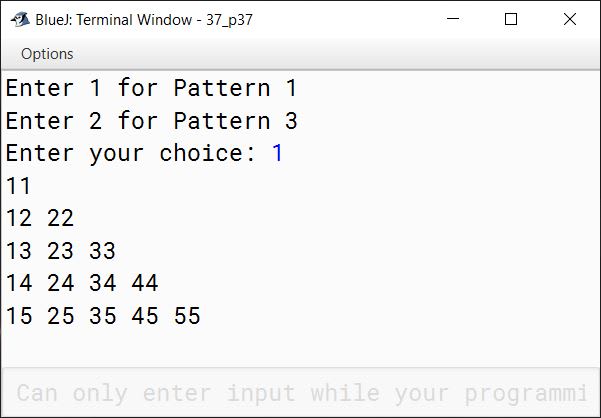
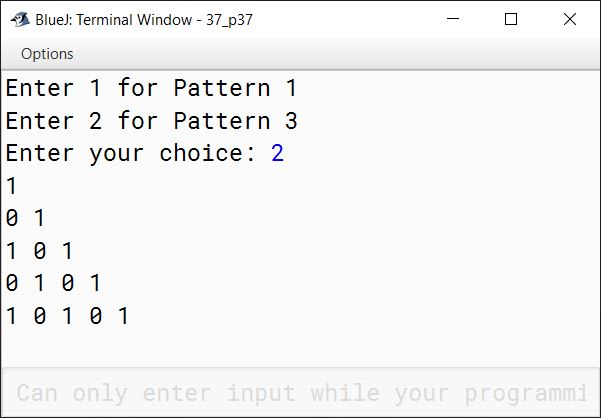
Answered By
1 Like