Computer Applications
Using the switch case statement, write a menu driven program for the following:
(a) To input a number and display only those factors of the numbers which are prime.
Sample Input: 84
Sample Output: 2, 3, 7
(b) A program that displays the multiplication table from 1 to 10, as shown:
1 2 3 4 5 6 7 8 9 10
2 4 6 8 10 12 14 16 18 20
3 6 9 12 15 18 21 24 27 30
……………………………..
……………………………..
……………………………..
9 18 27 36 45 54 63 72 81 90
10 20 30 40 50 60 70 80 90 100
Java
Java Nested for Loops
10 Likes
Answer
import java.util.Scanner;
public class KboatPrimeFactorsNTables
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter 1 for prime factors");
System.out.println("Enter 2 for multiplication tables");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
switch (ch) {
case 1:
System.out.print("Enter a number: ");
int num = in.nextInt();
for(int i = 2; i <= num/2; i++) {
int c = 0;
if(num % i == 0) {
for (int j = 1; j <= i; j++) {
if(i % j == 0)
c++;
}
if(c == 2)
System.out.print(i + " ");
}
}
break;
case 2:
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++)
System.out.print((i * j) + " ");
System.out.println();
}
break;
default:
System.out.println("Incorrect Choice");
}
}
}
Variable Description Table
Program Explanation
Output
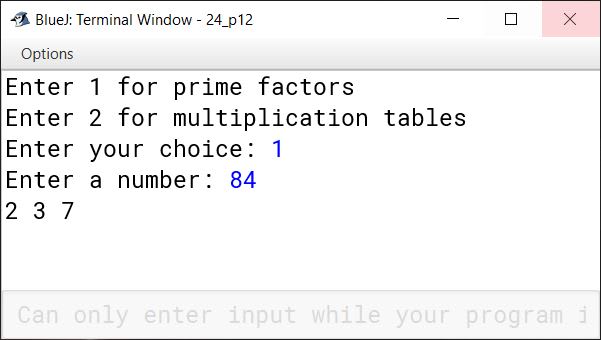
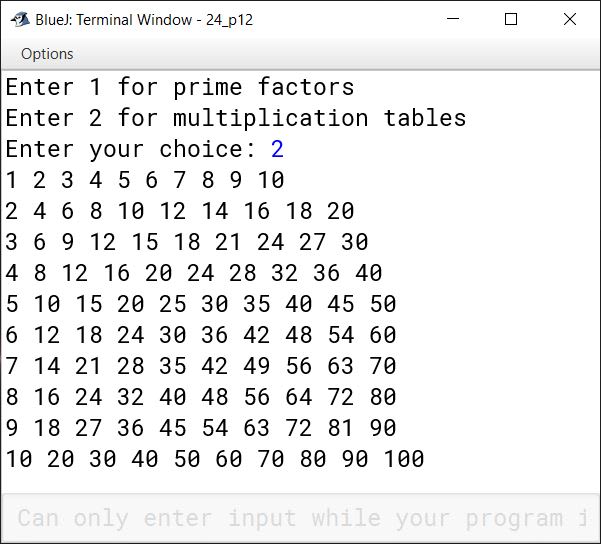
Answered By
4 Likes
Related Questions
Write a program in Java to display the following pattern:
5 4 3 2 1
5 4 3 2
5 4 3
5 4
5Write a program in Java to display the following pattern:
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15Write a program to generate a triangle or an inverted triangle till n terms based upon the user's choice.
Example 1:
Input: Type 1 for a triangle and
Type 2 for an inverted triangle
Enter your choice 1
Enter the number of terms 5
Sample Output:
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5Example 2:
Input: Type 1 for a triangle and
Type 2 for an inverted triangle
Enter your choice 2
Enter the number of terms 6
Sample Output:
6 6 6 6 6 6
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1Using the switch statement, write a menu driven program for the following:
(a) To print the Floyd's triangle:
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15(b) To display the following pattern:
I
I C
I C S
I C S EFor an incorrect option, an appropriate error message should be displayed.