Computer Applications
Using switch case, write a program to convert temperature from Fahrenheit to Celsius and Celsius to Fahrenheit.
Fahrenheit to Celsius formula: (32°F - 32) x 5/9
Celsius to Fahrenheit formula: (0°C x 9/5) + 32
Java
Java Conditional Stmts
17 Likes
Answer
import java.util.Scanner;
public class KboatTemperature
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type 1 for Fahrenheit to Celsius");
System.out.println("Type 2 for Celsius to Fahrenheit");
int choice = in.nextInt();
double ft = 0.0, ct = 0.0;
switch (choice) {
case 1:
System.out.print("Enter temperature in Fahrenheit: ");
ft = in.nextDouble();
ct = (ft - 32) * 5 / 9.0;
System.out.println("Temperature in Celsius: " + ct);
break;
case 2:
System.out.print("Enter temperature in Celsius: ");
ct = in.nextDouble();
ft = 9.0 / 5.0 * ct + 32;
System.out.println("Temperature in Fahrenheit: " + ft);
break;
default:
System.out.println("Incorrect Choice");
break;
}
}
}
Output
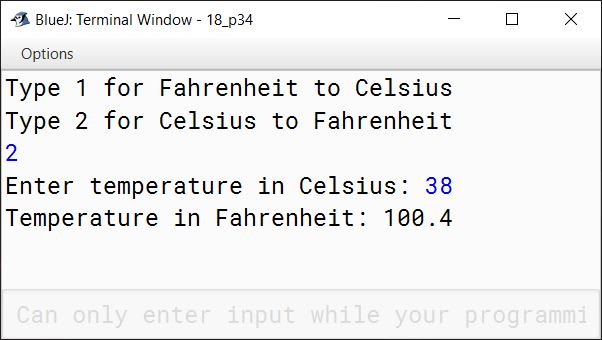
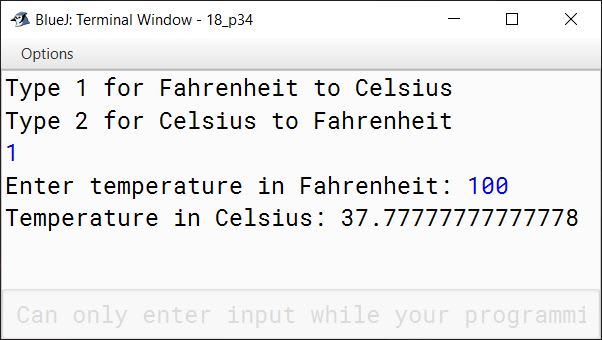
Answered By
7 Likes
Related Questions
A cloth showroom has announced the following festival discounts on the purchase of items based on the total cost of the items purchased:
Total Cost Discount Rate Less than Rs. 2000 5% Rs. 2000 to less than Rs. 5000 25% Rs. 5000 to less than Rs. 10,000 35% Rs. 10,000 and above 50% Write a program to input the total cost and to compute and display the amount to be paid by the customer availing the the discount.
Write a program in Java to accept three numbers and check whether they are Pythagorean Triplet or not. The program must display the message accordingly. [Hint: h2=p2+b2]
Star mall is offering discount on various types of products purchased by its customers. Following table shows different type of products and their respective code along with the discount offered. Based on the code entered, the mall is calculating the total amount after deducting the availed discount. Create a program to calculate total amount to be paid by the customer.
Item Item Code Discount Laptop L 5% LCD D 7% XBox X 10% Printer P 11% Write a Java program in which you input students name, class, roll number, and marks in 5 subjects. Find out the total marks, percentage, and grade according to the following table.
Percentage Grade >= 90 A+ >= 80 and < 90 A >= 70 and < 80 B+ >= 60 and < 70 B >= 50 and < 60 C >= 40 and < 50 D < 40 E