Computer Applications
Shasha Travels Pvt. Ltd. gives the following discount to its customers:
Ticket Amount | Discount |
---|---|
Above Rs. 70000 | 18% |
Rs. 55001 to Rs. 70000 | 16% |
Rs. 35001 to Rs. 55000 | 12% |
Rs. 25001 to Rs. 35000 | 10% |
Less than Rs. 25001 | 2% |
Write a program to input the name and ticket amount for the customer and calculate the discount amount and net amount to be paid. Display the output in the following format for each customer:
Sl. No. Name Ticket Charges Discount Net Amount
(Assume that there are 15 customers, first customer is given the serial no (SI. No.) 1, next customer 2 …….. and so on)
Java
Java Conditional Stmts
ICSE 2010
80 Likes
Answer
import java.util.Scanner;
public class KboatShashaTravels
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
String names[] = new String[15];
int amounts[] = new int[15];
for (int i = 0; i < 15; i++) {
System.out.print("Enter " + "Customer " + (i+1) + " Name: ");
names[i] = in.nextLine();
System.out.print("Enter " + "Customer " + (i+1) + " Ticket Charges: ");
amounts[i] = in.nextInt();
in.nextLine();
}
System.out.println("Sl. No.\tName\t\tTicket Charges\tDiscount\t\tNet Amount");
for (int i = 0; i < 15; i++) {
int dp = 0;
int amt = amounts[i];
if (amt > 70000)
dp = 18;
else if (amt >= 55001)
dp = 16;
else if (amt >= 35001)
dp = 12;
else if (amt >= 25001)
dp = 10;
else
dp = 2;
double disc = amt * dp / 100.0;
double net = amt - disc;
System.out.println((i+1) + "\t" + names[i]
+ "\t" + amounts[i] + "\t\t"
+ disc + "\t\t" + net);
}
}
}
Output
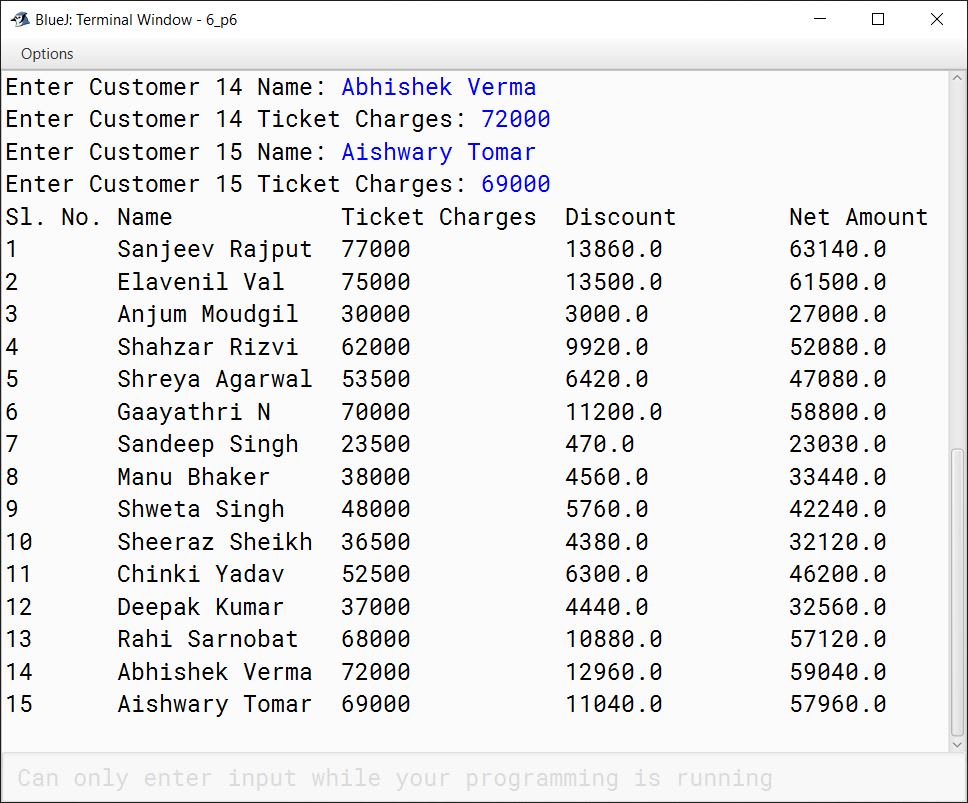
Answered By
31 Likes