Computer Applications
In order to reach the top of a pole, a monkey in his first attempt reaches to a height of 5 feet and in the subsequent jumps, he slips down by 2% of the height attained in the previous jump. The process repeats and finally the monkey reaches the top of the pole. Write a program to input height of the pole. Calculate and display the number of attempts the monkey makes to reach the top of the pole.
Java
Java Iterative Stmts
45 Likes
Answer
import java.util.Scanner;
public class KboatMonkeyPole
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter height of the pole: ");
double poleHt = in.nextDouble();
double jumpHt = 5.0;
int numAttempts = 1;
while (jumpHt < poleHt) {
jumpHt += 5.0;
jumpHt -= 2 * jumpHt / 100.0;
numAttempts++;
}
System.out.println("Number of Attempts = " + numAttempts);
}
}
Variable Description Table
Program Explanation
Output
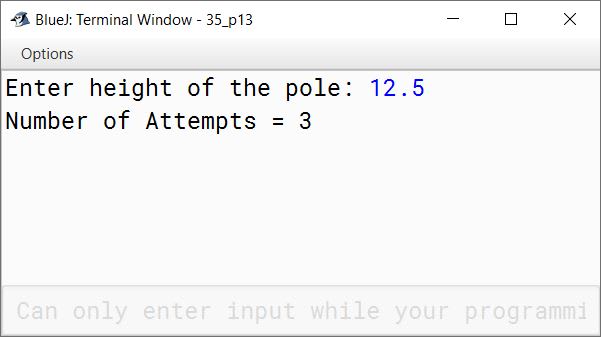
Answered By
19 Likes
Related Questions
Write a program in Java to find the sum of the given series:
S = 1/a + 1/a2 + 1/a3 + …… + 1/an
Write a program in Java to find the sum of the given series:
S = x/2 + x/5 + x/8 + x/11 + …… + x/20
Write a program to input Principal (p), Rate (r) and Time (t). Calculate and display the amount, which is compounded annually for each year by using the formula:
Simple Interest:
(si) = (prt) / 100
p = p + si
[Hint: The amount after each year is the Principal for the next year]
Write a menu driven program to input two positive numbers m and n (where m>n) and perform the following tasks:
(a) Find the sum of two numbers without using '+' operator.
(b) Find the product of two numbers without using '*' operator.
(c) Find the quotient and remainder of two numbers without using '/' and '%' operator.
[Hint: The last value obtained after each subtraction is the remainder and the number of iterations results in quotient.]
Sample Input: m=5, n=2
5 - 2 =3
3 - 2 = 1, thus Quotient = 2 and Remainder = 1