Computer Applications
In an examination, you have appeared for three subjects i.e. Physics, Chemistry and Biology. Write a program in Java to calculate the average mark obtained and finally display the marks in rounded-off form. Take Physics, Chemistry. and Biology marks as inputs.
Java
Java Math Lib Methods
131 Likes
Answer
import java.util.Scanner;
public class KboatAvgMarks
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter Marks");
System.out.print("Physics: ");
int p = in.nextInt();
System.out.print("Chemistry: ");
int c = in.nextInt();
System.out.print("Biology: ");
int b = in.nextInt();
double avg = (p + c + b) / 3.0;
long roundAvg = Math.round(avg);
System.out.println("Rounded Off Avg Marks = " + roundAvg);
}
}
Variable Description Table
Program Explanation
Output
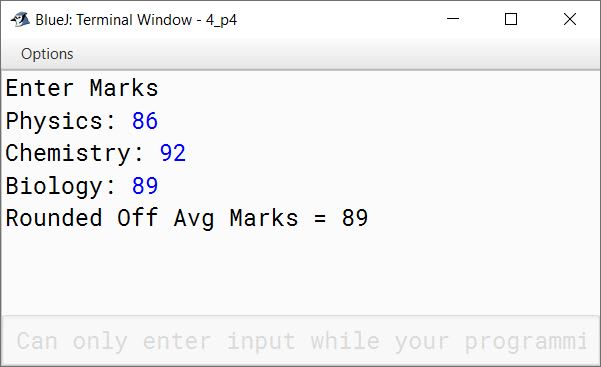
Answered By
57 Likes
Related Questions
Write a program to input a number and evaluate the results based on the number entered by the user:
(a) Natural logarithm of the number
(b) Absolute value of the number
(c) Square root of the number
(d) Cube of the number
(e) Random numbers between 0 (zero) and 1 (one).
Write a program in Java to calculate and display the hypotenuse of a Right-Angled Triangle by taking perpendicular and base as inputs.
Hint: h = √p2 + b2
You want to calculate the radius of a circle by using the formula:
Area = (22/7) * r2; where r = radius of a circle
Hence the radius can be calculated as:
r = √((7 * area) / 22)
Write a program in Java to calculate and display the radius of a circle by taking area as an input.
Write a program in Java to input three numbers and display the greatest and the smallest of the two numbers.
Hint: Use Math.min( ) and Math.max( )
Sample Input: 87, 65, 34
Sample Output: Greatest Number 87
Smallest number 34