Computer Applications
Define a class to overload the method generate() with the following variations:
void generate(int n, char ch) — Prints a pattern of n rows based on the value of
ch
:- If
ch
is'*'
, print a pattern of stars (*
).
n = 5
* * * * * * * * * * * * * * *
- If
ch
is'N'
, print a pattern of numbers.
n = 5
1 2 2 3 3 3 4 4 4 4 5 5 5 5 5
- For any other value of
ch
printInvalid Input
.
- If
double generate(int n) — Calculates and returns the sum of the following series:
1 - $\dfrac{1}{2}$ + $\dfrac{1}{3}$ - $\dfrac{1}{4}$ ….. to n termsdouble generate(double p, double q, double r) — Calculates and returns the value of z, where:
z = p2 + q2 - r2
public class KboatOverload
{
_______(1)_________ {
if (ch != '*'
&& Character.toLowerCase(ch) != 'n') {
System.out.println("Invalid Input");
}
else {
_______(2)_________
_______(3)_________
_______(4)_________
System.out.print("* ");
}
else {
System.out.print(i + " ");
}
}
System.out.println();
}
}
}
double generate(int n) {
int a = 1;
double sum = 0;
_______(5)_________
double t = _______(6)_________
t *= a;
_______(7)_________
a *= -1;
}
return sum;
}
_______(8)_________ {
double z = _______(9)_________
_______(10)_________
}
}
User Defined Methods
3 Likes
Answer
void generate(int n, char ch)
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
if (ch == '*') {
for (int i = 1; i <= n; i++) {
1.0 / i;
sum += t;
double generate(double p, double q, double r)
(p * p) + (q * q) - (r * r);
return z;
Explanation
public class KboatOverload
{
void generate(int n, char ch) {
if (ch != '*'
&& Character.toLowerCase(ch) != 'n') {
System.out.println("Invalid Input");
}
else {
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
if (ch == '*') {
System.out.print("* ");
}
else {
System.out.print(i + " ");
}
}
System.out.println();
}
}
}
double generate(int n) {
int a = 1;
double sum = 0;
for (int i = 1; i <= n; i++) {
double t = 1.0 / i;
t *= a;
sum += t;
a *= -1;
}
return sum;
}
double generate(double p, double q, double r) {
double z = (p * p) + (q * q) - (r * r);
return z;
}
}
Variable Description Table
Method: void generate(int n, char ch)
Variable Name | Type | Purpose |
---|---|---|
n | int | The number of rows to be printed in the pattern. |
ch | char | A character that determines the type of pattern: '*' for stars, 'N' for numbers. |
i | int | A loop variable used to iterate over the number of rows in the pattern. |
j | int | A loop variable used to iterate over the number of elements in a particular row of the pattern. |
Method: double generate(int n)
Variable Name | Type | Purpose |
---|---|---|
n | int | The number of terms in the series to be calculated. |
a | int | A multiplier that alternates the sign of the series terms to create alternating addition and subtraction. |
sum | double | Accumulated sum of the series terms to be returned as the result. |
t | double | The current term in the series, calculated as 1.0/i and adjusted by a for the alternating pattern. |
Method: double generate(double p, double q, double r)
Variable Name | Data Type | Purpose |
---|---|---|
p | double | The first component in the expression for z . |
q | double | The second component in the expression for z . |
r | double | The third component in the expression for z . |
z | double | The calculated result of the expression z = p2 + q2 - r2, which is returned by the method. |
Program Explanation
Let's go through the Java program step by step to understand how it works:
Method 1: generate(int n, char ch)
1. Input Validation:
- The method takes two parameters: an integer
n
(the number of rows) and a characterch
(which determines the type of pattern). - The method initially checks if the character
ch
is neither an asterisk ('*'
) nor the letter'N'
.- This condition is evaluated using
if (ch != '*' && Character.toLowerCase(ch) != 'n')
. - If the character
ch
does not match'*'
or'n'
, it prints "Invalid Input" and exits the method because the patterns are defined only for these characters.
- This condition is evaluated using
2. Pattern Generation:
- If
ch
is'*'
or'N'
, the method proceeds to generate a pattern involving multiple rows, based on the numbern
.
3. Nested Loop Construction:
Outer Loop:
for (int i = 1; i <= n; i++)
iterates from 1 throughn
, each iteration representing a new row of the pattern.- The value of
i
signifies the current row number and dictates how many elements will be printed in that row.
Inner Loop:
for (int j = 1; j <= i; j++)
iterates from 1 toi
, determining the number of characters to be printed in thei-th
row.- The inner loop runs precisely
i
times for each iteration of the outer loop, allowing for the growing number of elements in the pattern across consecutive rows.
4. Pattern Decision:
Star Pattern (
ch == '*'
):- If
ch
is an asterisk ('*'
), the inner loop prints a star followed by a space ("* "), usingSystem.out.print("* ");
. - This creates a symmetric triangular pattern of stars with each row
i
havingi
stars.
- If
Number Pattern (
ch == 'N'
):- If
ch
is the letter'N'
(case-insensitive check is performed withCharacter.toLowerCase(ch)
), the inner loop prints the current row numberi
repeatedly,i
times, followed by a space. - Each element in the row is generated using
System.out.print(i + " ");
. This creates a symmetric triangular pattern of numbers.
- If
5. Row Termination:
- After the inner loop completes its cycles for one row,
System.out.println();
is used to move the cursor to the next line before continuing to the next iteration of the outer loop, presenting each row on a new line.
Method 2: generate(int n)
1. Initialization:
- The method initializes an integer variable
a
to1
. This variable is used to alternate the sign of each term in the series. - A
double
variablesum
is initialized to0
. This will hold the accumulated sum of the series.
2. Iteration Over Terms:
for (int i = 1; i <= n; i++)
: The loop iterates from1
ton
. Each iteration corresponds to computing one term of the series.- The variable
i
represents the current term index in the series.
3. Term Calculation:
double t = 1.0 / i;
: This calculates the reciprocal of the current indexi
, which is the base value of the current term in the series.t *= a;
: Multiplies the base value bya
, effectively alternating the sign oft
:- When
a
is1
, the term remains positive. - When
a
is-1
, the term becomes negative.
- When
- The alternation in signs achieves the series' structure of alternating addition and subtraction.
4. Accumulation of the Series:
sum += t;
: Adds the current termt
to the cumulativesum
. Since the terms alternate between positive and negative based ona
, the overall effect is an accumulation according to the series pattern.
5. Prepare for the Next Term:
a *= -1;
: Flips the sign ofa
for the next term. This ensures the alternation continues correctly through the iterations.
6. Return the Result:
- After the loop completes,
return sum;
provides the final computed sum of the series ton
terms.
Method 3: generate(double p, double q, double r)
- This method calculates the expression
(p * p) + (q * q) - (r * r)
. - It squares
p
andq
, adds these squares together, and subtracts the square ofr
from this sum. - The result of this calculation is stored in the variable
z
, which is then returned as the output.
Output
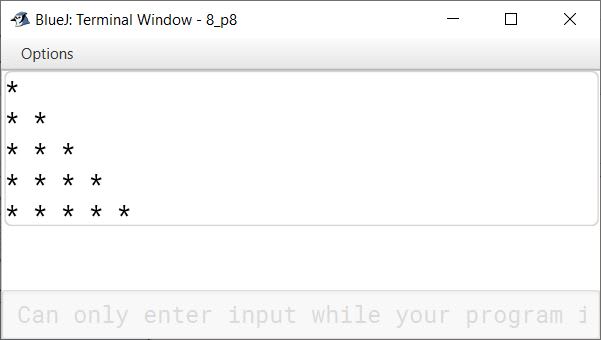
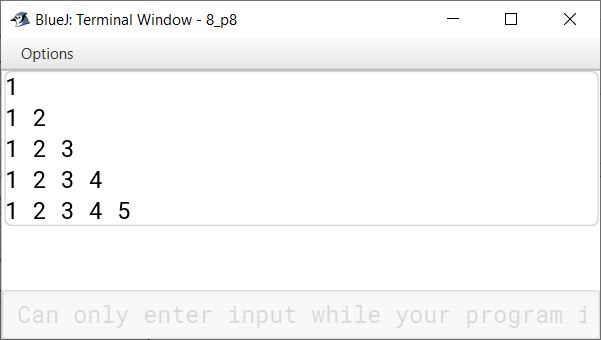
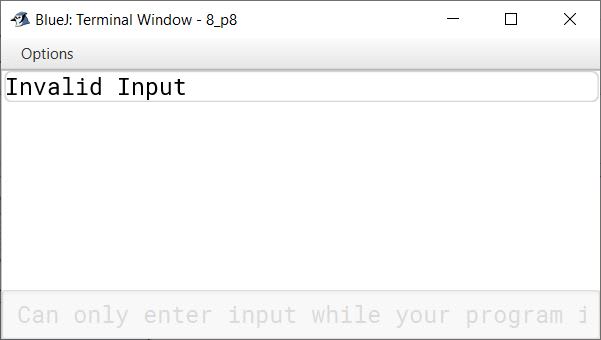
Answered By
2 Likes
Related Questions
Assertion (A): An argument is a value that is passed to a method when it is called.
Reason (R): Variables which are declared in a method prototype to receive values are called actual parameters
- Both Assertion (A) and Reason (R) are true and Reason (R) is a correct explanation of Assertion (A)
- Both Assertion (A) and Reason (R) are true and Reason (R) is not a correct explanation of Assertion(A)
- Assertion (A) is true and Reason (R) is false
- Assertion (A) is false and Reason (R) is true
Define a class to overload the method display() as follows:
void display(): To print the following format using nested loop.
1 2 1 2 1
1 2 1 2 1
1 2 1 2 1void display (int n, int m) : To print the quotient of the division of m and n if m is greater than n otherwise print the sum of twice n and thrice m.
double display (double a, double b, double c) — to print the value of z where
Which OOP principle implements method overloading?
Using switch statement write a menu driven program to overload a function series as follows:
(a) void series() — To find and display the sum of the following series:
S = 2 - 4 + 6 - 8 + 10 ….. - 20(b) void series(int n, int x) — To find and display the sum of the following series:
S = (x2 / 1!) + (x4 / 3!) + (x6 / 5!) + ……. to n terms