Computer Applications
Define a class with the following specifications
Class name : employee
Member variables
eno : employee number
ename : name of the employee
age : age of the employee
basic : basic salary (Declare the variables using appropriate data types)
Member methods
void accept( ) : accept the details using scanner class
void calculate( ) : to calculate the net salary as per the given specifications
net = basic + hra + da - pf
hra = 18.5% of basic
da = 17.45% of basic
pf = 8.10% of basic
if the age of the employee is above 50 he/she gets an additional allowance of ₹5000
void print( ) : to print the details as per the following format
eno ename age basic net
void main( ) : to create an object of the class and invoke the methods
Java
Java Classes
31 Likes
Answer
import java.util.Scanner;
public class employee
{
private int eno;
private String ename;
private int age;
private double basic;
private double net;
public void accept()
{
Scanner in = new Scanner(System.in);
System.out.print("Enter name : ");
ename = in.nextLine();
System.out.print("Enter employee no : ");
eno = in.nextInt();
System.out.print("Enter age : ");
age = in.nextInt();
System.out.print("Enter basic salary : ");
basic = in.nextDouble();
}
public void calculate()
{
double hra, da, pf;
hra = 18.5/100.0 * basic;
da = 17.45/100.0 * basic;
pf = 8.10/100.0 * basic;
net = basic + hra + da - pf;
if(age > 50)
net += 5000;
}
public void print()
{
System.out.println(eno + "\t" + ename + "\t" + age + "\t Rs."
+ basic + "\t Rs." + net );
}
public static void main(String args[])
{
employee ob = new employee();
ob.accept();
ob.calculate();
ob.print();
}
}
Output
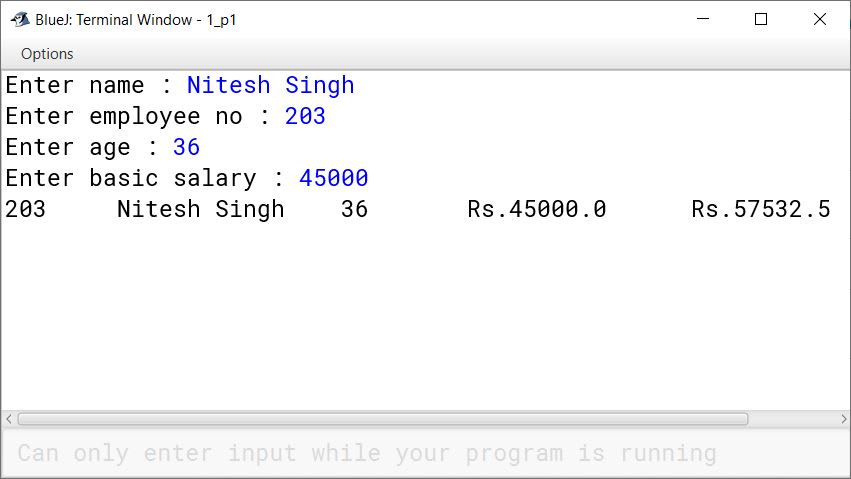
Answered By
15 Likes
Related Questions
Define a class to accept the elements of an array from the user and check for the occurrence of positive number, negative number and zero.
Example
Input Array: 12, -89, -56, 0, 45, 56
Output:
3 Positive Numbers
2 Negative Numbers
1 ZeroDefine a class to accept a number and check whether the number is valid number or not. A valid number is a number in which the eventual sum of digits of the number is equal to 1.
e.g., 352 = 3 + 5 + 2 = 10 = 1 + 0 = 1
Then 352 is a valid number.
Write the code to print following patterns
(i)
1 2 6 3 7 10 4 8 11 13 5 9 12 14 15
(ii)
A A A A A A A A B B A A C C C A D D D D E E E E E
Define a class Anagram to accept two words from the user and check whether they are anagram of each other or not.
An anagram of a word is another word that contains the same characters, only the order of characters is different.
For example, NOTE and TONE are anagram of each other.