Computer Applications
An electronics shop has announced a special discount on the purchase of Laptops as given below:
Category | Discount on Laptop |
---|---|
Up to ₹25,000 | 5.0% |
₹25,001 - ₹50,000 | 7.5% |
₹50,001 - ₹1,00,000 | 10.0% |
More than ₹1,00,000 | 15.0% |
Define a class Laptop described as follows:
Data members/instance variables:
- name
- price
- dis
- amt
Member Methods:
- A parameterised constructor to initialize the data members
- To accept the details (name of the customer and the price)
- To compute the discount
- To display the name, discount and amount to be paid after discount.
Write a main method to create an object of the class and call the member methods.
Java
Java Constructors
106 Likes
Answer
import java.util.Scanner;
public class Laptop
{
private String name;
private int price;
private double dis;
private double amt;
public Laptop(String s, int p)
{
name = s;
price = p;
}
public void compute() {
if (price <= 25000)
dis = price * 0.05;
else if (price <= 50000)
dis = price * 0.075;
else if (price <= 100000)
dis = price * 0.1;
else
dis = price * 0.15;
amt = price - dis;
}
public void display() {
System.out.println("Name: " + name);
System.out.println("Discount: " + dis);
System.out.println("Amount to be paid: " + amt);
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Customer Name: ");
String str = in.nextLine();
System.out.print("Enter Price: ");
int p = in.nextInt();
Laptop obj = new Laptop(str,p);
obj.compute();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
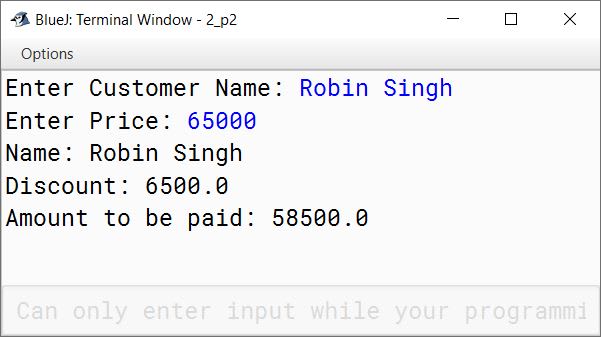
Answered By
58 Likes
Related Questions
Write a program by using a class with the following specifications:
Class name — Calculate
Instance variables:
- int num
- int f
- int rev
Member Methods:
- Calculate(int n) — to initialize num with n, f and rev with 0 (zero)
- int prime() — to return 1, if number is prime
- int reverse() — to return reverse of the number
- void display() — to check and print whether the number is a prime palindrome or not
Write a program by using a class in Java with the following specifications:
Class name — Stringop
Data members:
- String str
Member functions:
- Stringop() — to initialize str with NULL
- void accept() — to input a sentence
- void encode() — to replace and print each character of the string with the second next character in the ASCII table. For example, A with C, B with D and so on
- void print() — to print each word of the String in a separate line
Define a class Arrange described as below:
Data members/instance variables:
- String str (a word)
- String i
- int p (to store the length of the word)
- char ch;
Member Methods:
- A parameterised constructor to initialize the data member
- To accept the word
- To arrange all the alphabets of word in ascending order of their ASCII values without using the sorting technique
- To display the arranged alphabets.
Write a main method to create an object of the class and call the above member methods.
Write a program by using a class with the following specifications:
Class name — Hcflcm
Data members/instance variables:
- int a
- int b
Member functions:
- Hcflcm(int x, int y) — constructor to initialize a=x and b=y.
- void calculate( ) — to find and print hcf and lcm of both the numbers.