Computer Science
A new advanced Operating System, incorporating the latest hi-tech features has been designed by Opera Computer Systems. The task of generating copy protection code to prevent software privacy has been entrusted to the Security Department. The Security Department has decided to have codes containing a jumbled combination of alternate uppercase letters of the alphabet starting from A up to K (namely among A, C, E, G, I, K). The code may or may not be in the consecutive series of alphabets. Each code should not exceed 6 characters and there should be no repetition of characters. If it exceeds 6 characters, display an appropriate error message.
Write a program to input a code and its length. At the first instance of an error display "Invalid" stating the appropriate reason. In case of no error, display the message "Valid".
Sample Data:
Input:
n=4
ABCE
Output:
Invalid! Only alternate letters permitted!
Input:
n=4
AcIK
Output:
Invalid! Only uppercase letters permitted!
Input:
n = 7
Output:
Error! Length of String should not exceed 6 characters!
Input:
n=3
ACE
Output:
Valid
Input:
n=5
GEAIK
Output:
Valid
Java
Java String Handling
4 Likes
Answer
import java.util.Scanner;
public class KboatCodeCheck
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter length: ");
int len = in.nextInt();
in.nextLine();
if (len < 1 || len > 6) {
System.out.println("Error! Length of String should not exceed 6 characters!");
return;
}
System.out.println("Enter Code:");
String code = in.nextLine();
for (int i = 0; i < len; i++) {
char ch = code.charAt(i);
if (Character.isLowerCase(ch)) {
System.out.println("Invalid! Only uppercase letters permitted!");
return;
}
if (ch < 'A' || ch > 'K') {
System.out.println("Invalid! Only letters between A and K are permitted!");
return;
}
/*
* ASCII Code should be odd as we
* are taking alternate letters
* between A and K. We have already
* checked above that letter is
* between A and K. Now if we check
* for odd then it means that letter
* is one of A, C, E, G, I, K
*/
if (ch % 2 == 0) {
System.out.println("Invalid! Only alternate letters permitted!");
return;
}
/*
* Check for repetition
*/
int count = 0;
for (int j = 0; j < len; j++) {
if (ch == code.charAt(j)) {
count++;
}
}
if (count > 1) {
System.out.println("Invalid! Letter repetition is not permitted!");
return;
}
}
System.out.println("Valid");
}
}
Output
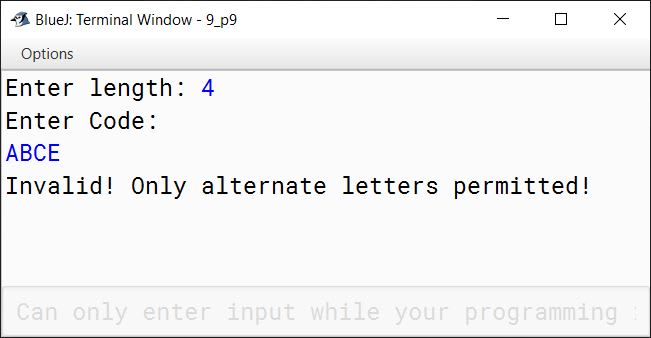
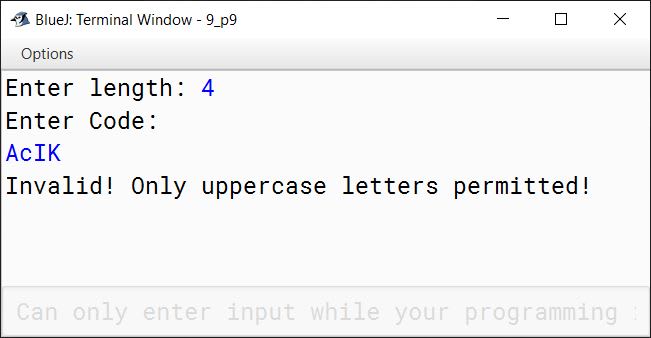
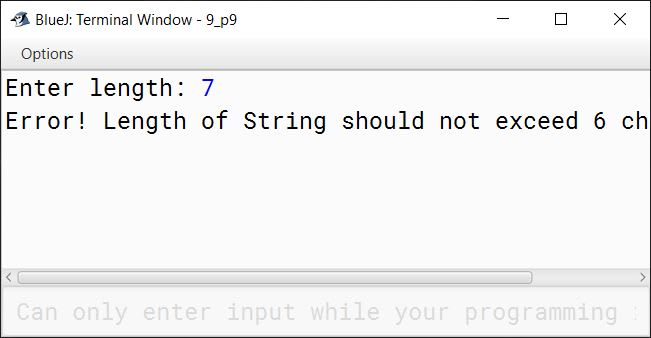
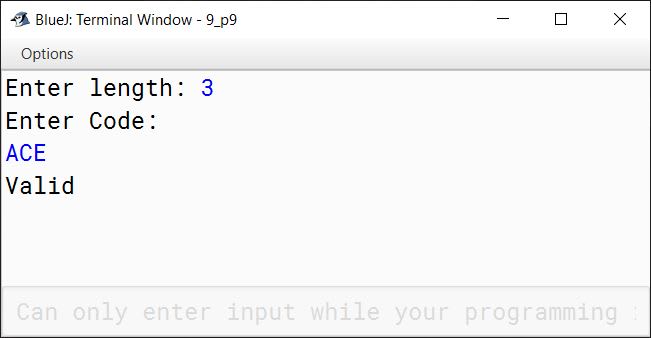
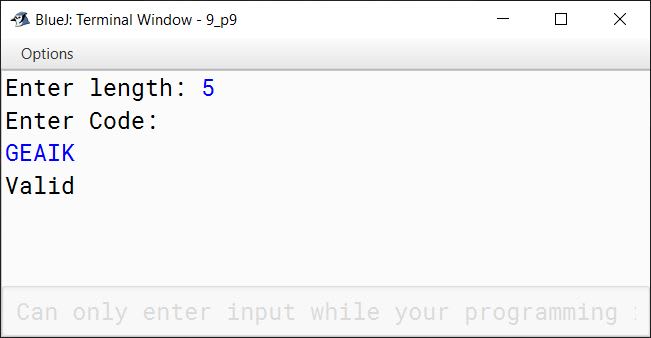
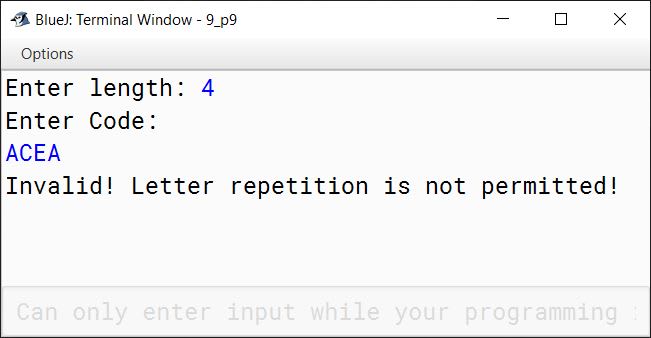
Answered By
3 Likes
Related Questions
While typing, a typist has created two or more consecutive blank spaces between the words of a sentence. Write a program in Java to eliminate multiple blanks between the words by a single blank.
Sample Input:
Indian Cricket team tour to AustraliaSample Output:
Indian Cricket team tour to AustraliaThe input in this question will consist of a number of lines of English text consisting of the letters of the English alphabet, the punctuation marks (') apostrophe, (.) full stop (, ) comma, (; ) semicolon, (:) colon and white space characters (blank, new line). Your task is to print the words of the text in reverse order without any punctuation marks other than blanks.
For example, consider the following input text:
This is a sample piece of text to illustrate this question.
If you are smart you will solve this right.The corresponding output would read as:
right this solve will you smart are you if question this illustrate to text of piece sample a is this
Note: Individual words are not reversed.
Input Format:
This first line of input contains a single integer n ( < = 20), indicating the number of lines in the input.
This is followed by lines of input text. Each line should accept a maximum of 80 characters.Output Format:
Output the text containing the input lines in reverse order without punctuations except blanks as illustrated above.
Test your program for the following data and some random data.
Sample Data:
Input:
2
Emotions controlled and directed to work, is character.
By Swami Vivekananda.Output:
Vivekananda Swami By character is work to directed and controlled EmotionsInput:
1
Do not judge a book by its cover.Output:
cover its by book a judge not DoRead a single sentence which terminates with a full stop (.). The words are to be separated with a single blank space and are in lower case. Arrange the words contained in the sentence according to the length of the words in ascending order. If two words are of the same length then the word occurring first in the input sentence should come first. For both, input and output the sentence must begin in upper case.
Test your program for given data and also some random data:
Input:
The lines are printed in reverse order.
Output:
In the are lines order printed reverse.Input:
Print the sentence in ascending order.
Output:
In the print order sentence ascending.Input:
I love my Country.
Output:
I my love Country.Write a program in Java to accept a four-letter word. Display all the probable four letter combinations such that no letter should be repeated in the output within each combination.
Sample Input:
PARKSample Output:
PAKR, PKAR, PRAK, APRK, ARPK, AKPR, and so on.