Assignment Questions
Question 1
Write a program to generate the following output.
*
* #
* # *
* # * #
* # * # *
Answer
public class KboatPattern
{
public static void main() {
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
if (j % 2 == 0)
System.out.print("# ");
else
System.out.print("* ");
}
System.out.println();
}
}
}
Output
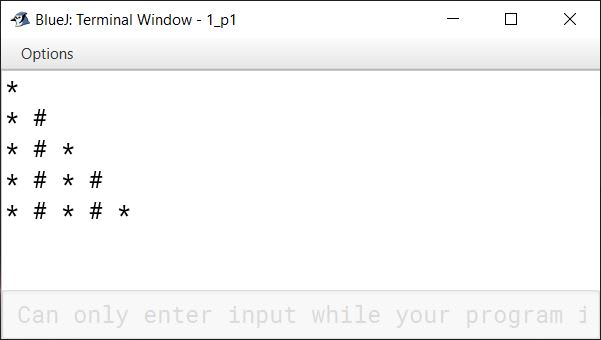
Question 2
Write a program to print the series given below.
5 55 555 5555 55555 555555
Answer
public class Kboat5Series
{
public static void main(String args[]) {
for (int i = 1; i <= 6; i++) {
for (int j = 0; j < i; j++) {
System.out.print('5');
}
System.out.print(' ');
}
}
}
Output
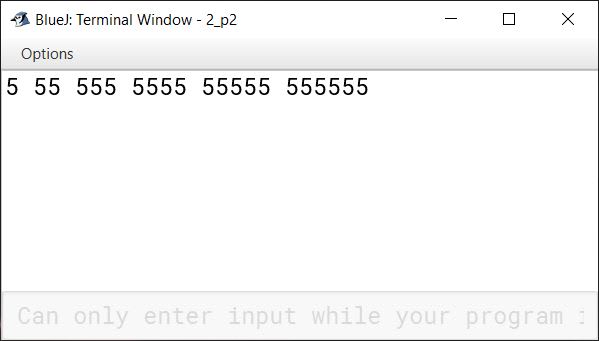
Question 3(i)
Write a program in Java to display the following patterns.
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
Answer
public class KboatPattern
{
public static void main(String args[]) {
int a = 1;
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(a++ + "\t");
}
System.out.println();
}
}
}
Output
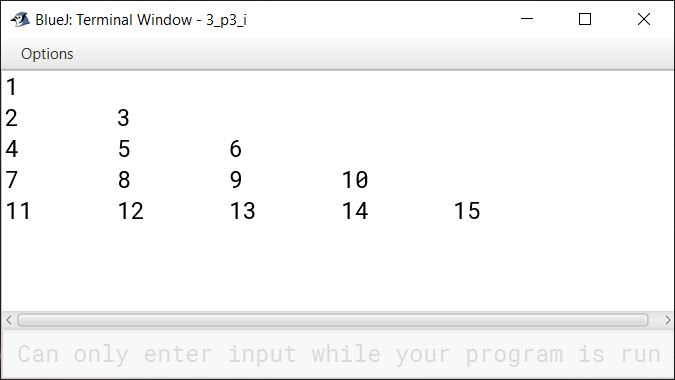
Question 3(ii)
Write a program in Java to display the following patterns.
1 * * * *
* 2 * * *
* * 3 * *
* * * 4 *
* * * * 5
Answer
public class KboatPattern
{
public static void main(String args[]) {
char ch = '*';
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= 5 ; j++) {
if(i == j)
System.out.print(i + " ");
else
System.out.print(ch + " ");
}
System.out.println();
}
}
}
Output
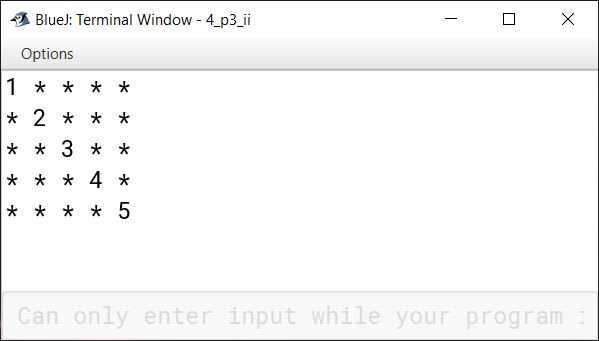
Question 3(iii)
Write a program in Java to display the following patterns.
#
* *
# # #
* * * *
# # # # #
Answer
public class KboatPattern
{
public static void main(String args[]) {
char ch1 = '#', ch2 = '*';
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
if (i % 2 == 0)
System.out.print(ch2 + " ");
else
System.out.print(ch1 + " ");
}
System.out.println();
}
}
}
Output
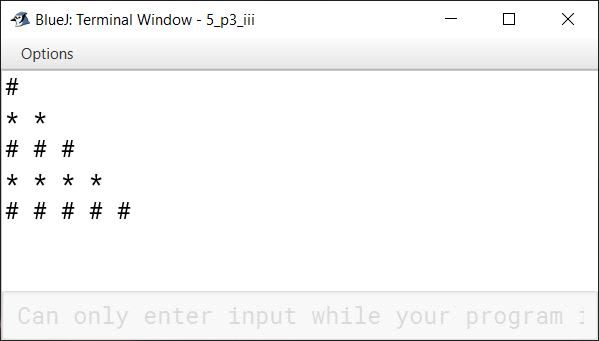
Question 3(iv)
Write a program in Java to display the following patterns.
1 * * * *
2 2 * * *
3 3 3 * *
4 4 4 4 *
5 5 5 5 5
Answer
public class KboatPattern
{
public static void main(String args[]) {
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(i);
}
for (int k = 1; k <= 5 - i; k++) {
System.out.print('*');
}
System.out.println();
}
}
}
Output
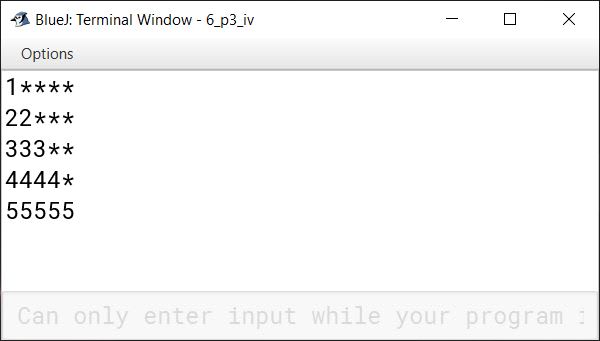
Question 3(v)
Write a program in Java to display the following patterns.
* * * * 5
* * * 4
* * 3
* 2
1
Answer
public class KboatPattern
{
public static void main(String args[]) {
char ch = '*';
for (int i = 5; i >= 1; i--) {
for (int j = i-1; j >= 1 ; j--)
System.out.print(ch + " ");
System.out.print(i);
System.out.println();
}
}
}
Output
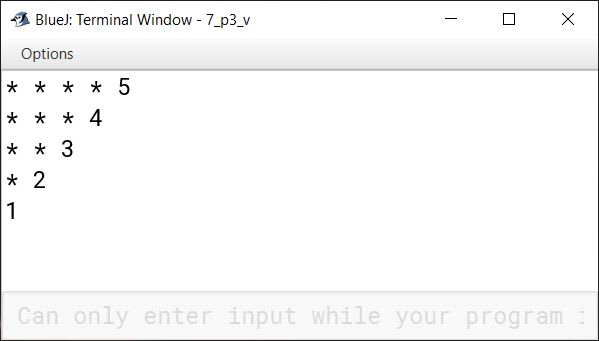
Question 3(vi)
Write a program in Java to display the following patterns.
A B C D E
A B C D
A B C
A B
A
Answer
public class KboatPattern
{
public static void main(String args[]) {
for (int i = 69; i >= 65; i--) {
for (int j = 65; j <= i; j++) {
System.out.print((char)j);
}
System.out.println();
}
}
}
Output
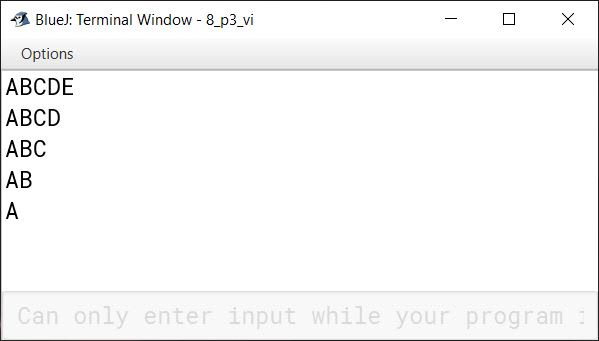
Question 3(vii)
Write a program in Java to display the following patterns.
5 4 3 2 1
4 3 2 1
3 2 1
2 1
1
Answer
public class KboatPattern
{
public static void main(String args[]) {
for (int i = 5; i >= 1; i--) {
for (int j = i; j >= 1; j--)
System.out.print( j + " ");
System.out.println();
}
}
}
Output
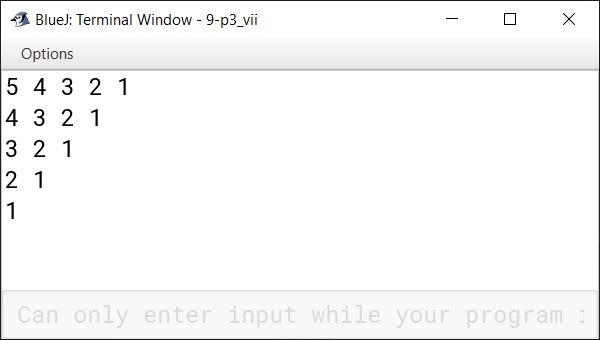
Question 3(viii)
Write a program in Java to display the following patterns.
J
J A
J A V
J A V A
Answer
public class KboatPattern
{
public static void main(String args[]) {
String str = "JAVA";
int len = str.length();
for(int i = 0; i < len; i++) {
for(int j = 0; j <= i; j++) {
System.out.print(str.charAt(j) + " ");
}
System.out.println();
}
}
}
Output
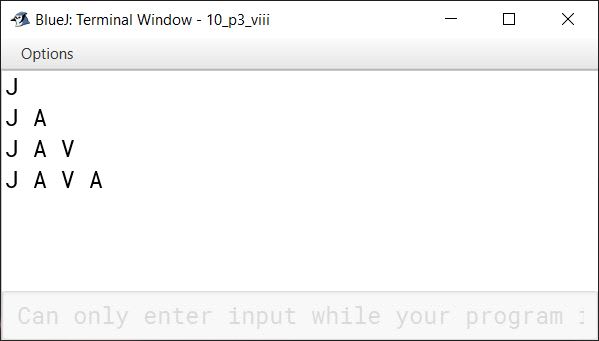
Question 3(ix)
Write a program in Java to display the following patterns.
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Answer
public class KboatPattern
{
public static void main(String args[]) {
for(int i = 5; i >= 1; i--) {
for(int j = 1; j <= i; j++)
System.out.print(j + " ");
System.out.println();
}
for(int i = 2; i <= 5; i++) {
for(int j = 1; j <= i; j++)
System.out.print(j + " ");
System.out.println();
}
}
}
Output
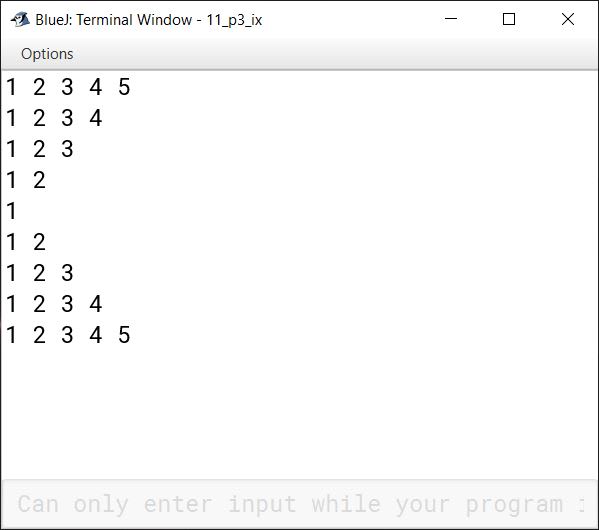
Question 3(x)
Write a program in Java to display the following patterns.
1 2 3 4 5
2 3 4 5
3 4 5
4 5
5
4 5
3 4 5
2 3 4 5
1 2 3 4 5
Answer
public class KboatPattern
{
public static void main(String args[]) {
for(int i = 1; i <= 5; i++) {
for (int j = 1; j < i; j++) {
System.out.print(" ");
}
for (int k = i; k <= 5; k++) {
System.out.print(k + " ");
}
System.out.println();
}
for(int i = 4; i >= 1 ; i--) {
for (int j = 1; j < i; j++) {
System.out.print(" ");
}
for (int k = i; k <= 5; k++) {
System.out.print(k + " ");
}
System.out.println();
}
}
}
Output
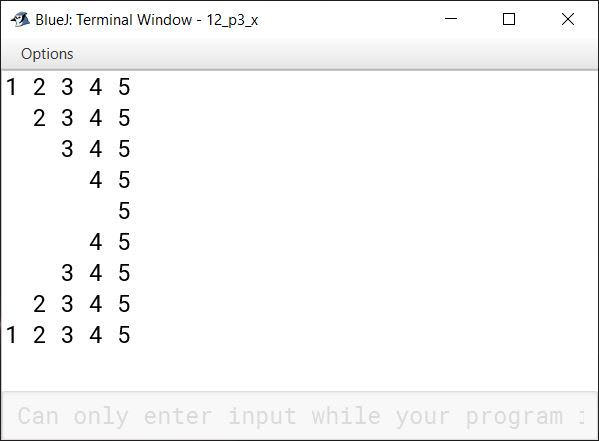
Question 4(i)
Distinguish between the following:
break statement and labelled break statement
Answer
break statement | labelled break statement |
---|---|
break statement is used to take the control out of the loop control structure immediately enclosing it. | Labelled break statement transfers program control out of the code block whose label is specified as its target. |
Syntax:break; | Syntax:break <label>; |
For example, for (int i = 1; i <= 3; i++) { for(int j = 1; j <= 2; j++) { if (i == 2) { break; } System.out.println("Iteration value of inner loop: " + j); } System.out.println(); } | For example, outer: for (int i = 1; i <= 3; i++) { for(int j = 1; j <= 2; j++) { if (i == 2) { System.out.println("Terminating the Outer-loop"); break outer; } System.out.println("i =" + i +" ; j =" + j); } |
Question 4(ii)
Distinguish between the following:
continue statement and labelled continue statement
Answer
continue statement | labelled continue statement |
---|---|
continue statement is used to skip the current iteration of a loop and move on to the next iteration. | A labelled continue statement skips the current iteration of an outer loop by using a label before the loop and including the same label in continue statement. |
Syntax:continue; | Syntax:continue <label>; |
For example, for (int i = 0; i <= 3; i++) { System.out.println("i =" + i); for(int j = 0; j < 3; j++) { if (i == 2) { continue; } System.out.print("j = " + j + " "); } System.out.println(); } | For example, outerLoop: for (int i = 0; i <= 3; i++) { for(int j = 0; j < 3; j++) { if (i == 2) { continue outerLoop; System.out.println("i =" + i +" ; j =" + j); } } } |
Question 5(i)
What will be the value of sum after each of the following nested loops is executed?
int sum = 0;
for (int i = 0; i <= 10; i++)
for (int j = 0; j <= 10; j++)
sum += i;
Answer
Sum = 605
Explanation
The outer loop executes 11 times. For each iteration of outer loop, the inner loop executes 11 times. For every value of j
, i
is added to sum 11 times. Consider the following table for the value of sum
with each value of i
and j
.
i | j | Sum | Remarks |
---|---|---|---|
0 | 0 - 10 | 0 | 0 is added to sum 11 times |
1 | 0 - 10 | 11 | 1 is added to sum 11 times ⇒ 0 + 11 = 11 |
2 | 0 - 10 | 33 | 2 is added to sum 11 times ⇒ 11 + 22 = 33 |
3 | 0 - 10 | 66 | 3 is added to sum 11 times ⇒ 33 + 33 = 66 |
4 | 0 - 10 | 110 | 4 is added to sum 11 times ⇒ 66 + 44 = 110 |
5 | 0 - 10 | 165 | 5 is added to sum 11 times ⇒ 110 + 55 = 165 |
6 | 0 - 10 | 231 | 6 is added to sum 11 times ⇒ 165 + 66 = 231 |
7 | 0 - 10 | 308 | 7 is added to sum 11 times ⇒ 231 + 77 = 308 |
8 | 0 - 10 | 396 | 8 is added to sum 11 times ⇒ 308 + 88 = 396 |
9 | 0 - 10 | 495 | 9 is added to sum 11 times ⇒ 396 + 99 = 495 |
10 | 0 - 10 | 605 | 10 is added to sum 11 times ⇒ 495 + 110 = 605 |
11 | Loop terminates |
Question 5(ii)
What will be the value of sum after each of the following nested loops is executed?
int sum = 0;
for (int i = 1; i <= 3; i++)
for (int j = 1; j <= 3; j++)
sum = sum + (i + j);
Answer
Sum = 36
Explanation
The outer loop executes 3 times. For each iteration of outer loop, the inner loop executes 3 times. For every value of j
, i + j
is added to sum 3 times. Consider the following table for the value of sum
with each value of i
and j
.
i | j | Sum | sum + (i + j) |
---|---|---|---|
1 | 1 | 2 | 0 + 1 + 1 |
2 | 5 | 2 + 1 + 2 | |
3 | 9 | 5 + 1 + 3 | |
2 | 1 | 12 | 9 + 2 + 1 |
2 | 16 | 12 + 2 + 2 | |
3 | 21 | 16 + 2 + 3 | |
3 | 1 | 25 | 21 + 3 + 1 |
2 | 30 | 25 + 3 + 2 | |
3 | 36 | 30 + 3 + 3 | |
4 | Loop terminates |
Question 6
Write a program to generate a triangle or an inverted triangle till n terms based upon the user's choice of triangle to be displayed.
Example 1
Input:
Type 1 for a triangle and type 2 for an inverted triangle
1
Enter the number of terms
5
Output:
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
Example 2
Input:
Type 1 for a triangle and type 2 for an inverted triangle
2
Enter the number of terms
6
Output:
6 6 6 6 6 6
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1
Answer
import java.util.Scanner;
public class KboatPattern
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type 1 for a triangle");
System.out.println("Type 2 for an inverted triangle");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
System.out.print("Enter the number of terms: ");
int n = in.nextInt();
switch (ch) {
case 1:
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(i + " ");
}
System.out.println();
}
break;
case 2:
for (int i = n; i > 0; i--) {
for (int j = 1; j <= i; j++) {
System.out.print(i + " ");
}
System.out.println();
}
break;
default:
System.out.println("Incorrect Choice");
}
}
}
Output
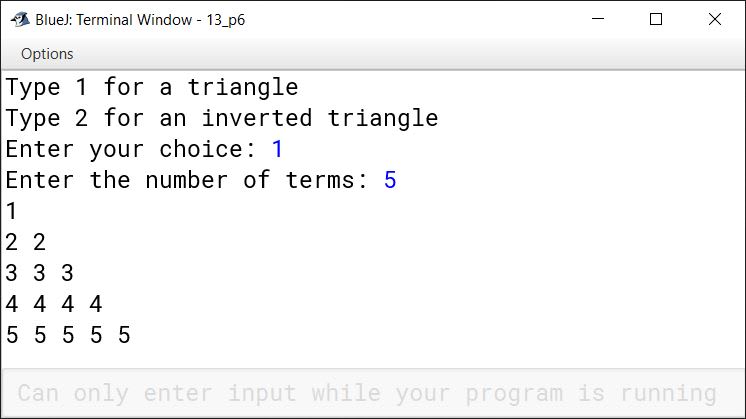
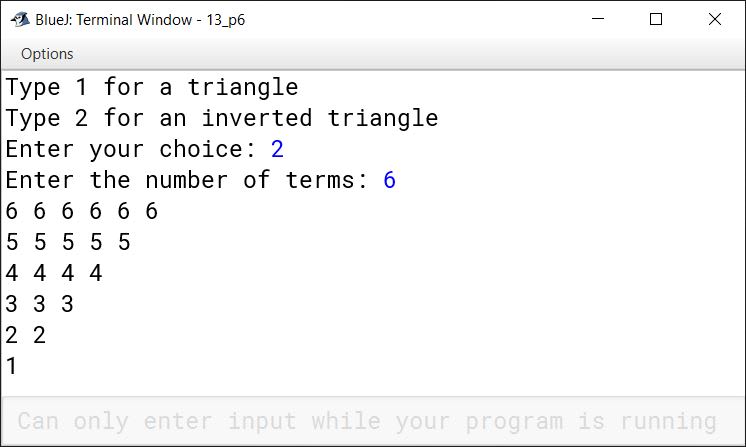