State True or False:
The Python interpreter handles logical errors during code execution.
Answer
False
Reason — The Python interpreter handles syntax errors during code execution, but it does not handle logical errors. Logical errors, also known as semantic errors, occur when the code is syntactically correct but does not produce the expected output due to incorrect logic or algorithm. These types of errors are caught during the testing and debugging phase, not during code execution by the interpreter.
Identify the output of the following code snippet:
text = "PYTHONPROGRAM"
text = text.replace('PY', '#')
print(text)
- #THONPROGRAM
- ##THON#ROGRAM
- #THON#ROGRAM
- #YTHON#ROGRAM
Answer
#THONPROGRAM
Reason — The replace()
method in Python searches for a specified substring and replaces it with another. In the given code, the substring 'PY' in the string "PYTHONPROGRAM" is replaced with the character '#'. As a result, the string "PYTHONPROGRAM" becomes "#THONPROGRAM", and that's the output displayed.
Which of the following expressions evaluates to False ?
- not(True) and False
- True or False
- not(False and True)
- True and not(False)
Answer
not(True) and False
Reason —
not(True) and False
evaluates toFalse and False
, which isFalse
.True or False
evaluates toTrue
.not(False and True)
evaluates tonot(False)
, which isTrue
.True and not(False)
evaluates toTrue and True
, which isTrue
.
What is the output of the expression ?
country = 'International'
print(country.split("n"))
('I', 'ter', 'atio', 'aI')
['I', 'ter', 'atio', 'al']
['I', 'n', 'ter', 'n', 'atio', 'n', 'al']
Error
Answer
['I', 'ter', 'atio', 'al']
Reason — The code defines a string country = 'International'
and uses the split("n")
method to split the string at each occurrence of the letter "n". This breaks the string into a list of parts: ['I', 'ter', 'atio', 'al']
, with each occurrence of "n" removed and the remaining substrings stored as separate elements in the list. The print()
function then outputs this list.
What will be the output of the following code snippet ?
message = "World Peace"
print(message[-2::-2])
Answer
ce lo
The code
message[-2::-2]
starts from the second-last (-2) character of the string "World Peace", which is "c".It moves backwards in steps of 2 to select every second character going towards the start of the string.
The traversal goes as follows:
- Index -2: Character is "c".
- Index -4: Character is "e".
- Index -6: Character is a space (" ").
- Index -8: Character is "l".
- Index -10: Character is "o".
The output is "ce lo".
What will be the output of the following code ?
tuple1 = (1, 2, 3)
tuple2 = tuple1
tuple1 += (4, )
print(tuple1 == tuple2)
- True
- False
- tuple1
- Error
Answer
False
Reason — In the above code, tuple1
is initially assigned the tuple (1, 2, 3), and tuple2
references the same tuple. When the line tuple1 += (4, )
is executed, it modifies tuple1
to (1, 2, 3, 4) because the +=
operator creates a new tuple rather than modifying the existing one. Thus, tuple1
now contains (1, 2, 3, 4), while tuple2
remains (1, 2, 3). Therefore, the expression tuple1 == tuple2
evaluates to False.
If my_dict is a dictionary as defined below, then which of the following statements will raise an exception ?
my_dict = {'apple' : 10, 'banana' : 20, 'orange' : 30}
- my_dict.get('orange')
- print(my_dict['apple', 'banana'])
- my_dict['apple'] = 20
- print(str(my_dict))
Answer
print(my_dict['apple', 'banana'])
Reason — In Python dictionaries, keys must be accessed individually or as a single key. The expression my_dict['apple', 'banana']
attempts to use a tuple as a key (i.e., ('apple', 'banana')), which does not exist in my_dict
. As a result, this will raise an Error. The other statements will not raise exceptions; my_dict.get('orange')
will return 30, my_dict['apple'] = 20
will update the value for the key 'apple', and print(str(my_dict))
will print the dictionary as a string.
What does the list.remove(x) method do in Python ?
- Removes the element at index x from the list
- Removes the first occurrence of value x from the list
- Removes all occurrences of value x from the list
- Removes the last occurrence of value x from the list
Answer
Removes the first occurrence of value x from the list.
Reason — The list.remove(x)
method in Python searches for the first occurrence of the value x
in the list and removes it. If the specified value is not found, it raises an Error.
If a table which has one Primary key and two alternate keys. How many Candidate keys will this table have ?
- 1
- 2
- 3
- 4
Answer
3
Reason — In a table, the primary key uniquely identifies each record, and alternate keys also uniquely identify records but are not the primary key. In this case, with one primary key and two alternate keys, the table will have three candidate keys in total: one primary key and two alternate keys. Candidate keys are potential keys that can uniquely identify records in a table.
Write the missing statement to complete the following code:
file = open("example.txt", "r")
data = file.read(100)
............... #Move the file pointer to the beginning of the file
next_data = file.read(50)
file.close()
Answer
file.seek(0)
Reason — To move the file pointer to the beginning of the file, we can use the seek()
method. When we pass 0 as the offset to this function, it moves the file pointer to the beginning of the file.
So the complete code is:
file = open("example.txt", "r")
data = file.read(100)
file.seek(0) # Move the file pointer to the beginning of the file
next_data = file.read(50)
file.close()
State whether the following statement is True or False:
The finally
block in Python is executed only if no exception occurs in the try block.
Answer
False
Reason — The finally
block in Python is always executed after the try
block, regardless of whether an exception occurred or not.
What will be the output of the following code ?
c = 10
def add():
global c
c = c + 2
print(c, end = '#')
add()
c = 15
print(c, end = '%')
- 12%15#
- 15#12%
- 12#15%
- 12%15#
Answer
12#15%
Reason —
- The variable
c
is initially set to 10. - The function
add()
is defined. Inside this function, theglobal
keyword is used to indicate that the variablec
refers to the global variable defined outside the function. - Inside
add()
: Whenadd()
is called:- The value of
c
(which is 10) is accessed. - It is then incremented by 2, making
c
equal to 12. - The new value of
c
(12) is printed, followed by a#
symbol.
- The value of
- After calling the function, the global
c
is now 12. However, it is then explicitly set to 15. - Finally, the new value of
c
(15) is printed, followed by a%
symbol.
Which SQL command can change the degree of an existing relation ?
Answer
The SQL command that can change the degree of an existing relation (table) is ALTER TABLE
.
What will be the output of the query ?
SELECT * FROM products WHERE product_name LIKE 'App%';
- Details of all products whose names start with 'App'
- Details of all products whose names end with 'App'
- Names of all products whose names start with 'App'
- Names of all products whose names end with 'App'
Answer
Details of all products whose names start with 'App'
Reason — The SELECT *
statement indicates that every column of data should be returned for each matching record. It uses the LIKE
operator with the pattern 'App%', where the %
wildcard represents any sequence of characters. Therefore, this query will return details of all products from the products
table where the product_name
starts with 'App', including any characters that may follow.
In which datatype the value stored is padded with spaces to fit the specified length.
- DATE
- VARCHAR
- FLOAT
- CHAR
Answer
CHAR
Reason — The CHAR
data type in SQL is used to store fixed-length strings. When a value is stored in a CHAR
field, it is padded with spaces to fit the specified length.
Which aggregate function can be used to find the cardinality of a table ?
- sum()
- count()
- avg()
- max()
Answer
count()
Reason — The count()
aggregate function is used to find the cardinality of a table, which refers to the number of rows in that table.
Which protocol is used to transfer files over the Internet ?
- HTTP
- FTP
- PPP
- HTTPS
Answer
FTP
Reason — The File Transfer Protocol (FTP) is used for transferring files over the Internet. It allows users to upload, download, and manage files on remote servers.
Which network device is used to connect two networks that use different protocols ?
- Modem
- Gateway
- Switch
- Repeater
Answer
Gateway
Reason — A gateway is a network device that connects two networks that use different protocols, enabling communication between them.
Which switching technique breaks data into smaller packets for transmission, allowing multiple packets to share the same network resources.
Answer
Packet switching is a method of data transmission where data is broken into smaller packets before being sent over the network. Each packet can take different routes to reach the destination, allowing multiple packets from various sources to share the same network resources simultaneously.
Assertion (A): Positional arguments in Python functions must be passed in the exact order in which they are defined in the function signature.
Reasoning (R): This is because Python functions automatically assign default values to positional arguments.
- Both A and R are true, and R is the correct explanation of A.
- Both A and R are true, and R is not the correct explanation of A.
- A is true but R is false.
- A is false but R is true.
Answer
A is true but R is false.
Explanation
Positional arguments must be passed in the order they are defined in the function signature in Python. Python does not automatically assign default values to positional arguments. Default values must be explicitly defined in the function signature, if not provided, the function will raise an error.
Assertion (A): A SELECT command in SQL can have both WHERE and HAVING clauses.
Reasoning (R): WHERE and HAVING clauses are used to check conditions, therefore, these can be used interchangeably.
- Both A and R are true, and R is the correct explanation of A.
- Both A and R are true, and R is not the correct explanation of A.
- A is true but R is false.
- A is false but R is true.
Answer
A is true but R is false.
Explanation
A SQL SELECT
command can have both WHERE
and HAVING
clauses. The WHERE
clause filters records before any grouping occurs, while the HAVING
clause filters records after grouping. Therefore, they cannot be used interchangeably as they operate at different stages of query execution.
How is a mutable object different from an immutable object in Python ?
Identify one mutable object and one immutable object from the following:
(1, 2), [1, 2], {1:1, 2:2}, '123'
Answer
In Python, a mutable object can be changed after it is created (e.g., lists, dictionaries), while an immutable object cannot be modified after its creation (e.g., tuples, strings).
An example of a mutable object from the given options is [1, 2] (list) and {1:1, 2:2} (dictionary). [Write any one]
An example of an immutable object from the options is '123' (string) and (1, 2) (tuple). [Write any one]
Give two examples of each of the following:
- Arithmetic operators
- Relational operators
Answer
Arithmetic Operators — +, -
Relational Operators — >, ==
If L1 = [1, 2, 3, 2, 1, 2, 4, 2, ...], and L2 = [10, 20, 30, ...], then
(Answer using builtin functions only)
(a) Write a statement to count the occurrences of 4 in L1.
OR
(b) Write a statement to sort the elements of list L1 in ascending order.(a) Write a statement to insert all the elements of L2 at the end of L1.
OR
(b) Write a statement to reverse the elements of list L2.
Answer
(a)
L1.count(4)
OR
(b)L1.sort()
L1.extend(L2)
OR
(b)L2.reverse()
Identify the correct output(s) of the following code. Also write the minimum and the maximum possible values of the variable b.
import random
a = "Wisdom"
b = random.randint(1, 6)
for i in range(0, b, 2):
print(a[i], end = '#')
- W#
- W#i#
- W#s#
- W#i#s#
Answer
W#, W#s#
Minimum and maximum possible values of the variable b
is 1, 6.
Reason — The code imports the random
module and initializes a string variable a
with the value "Wisdom". It then generates a random integer b
between 1 and 6, inclusive. A for
loop iterates over a range from 0 to b
in steps of 2, which means it only considers even indices (0, 2, 4) of the string a
. In each iteration, it prints the character at the current index i
of the string a
, followed by a '#'
character, without moving to a new line.
For possible values of b
:
For b = 1:
- Range:
range(0, 1, 2)
→ Only includes 0 - Step 1:
i = 0
→ Prints W# - Final Output:
W#
For b = 2:
- Range:
range(0, 2, 2)
→ Only includes 0 - Step 1:
i = 0
→ Prints W# - Final Output:
W#
For b = 3:
- Range:
range(0, 3, 2)
→ Includes 0, 2 - Step 1:
i = 0
→ Prints W# - Step 2:
i = 2
→ Prints s# - Final Output:
W#s#
For b = 4:
- Range:
range(0, 4, 2)
→ Includes 0, 2 - Step 1:
i = 0
→ Prints W# - Step 2:
i = 2
→ Prints s# - Final Output:
W#s#
For b = 5:
- Range:
range(0, 5, 2)
→ Includes 0, 2, 4 - Step 1:
i = 0
→ Prints W# - Step 2:
i = 2
→ Prints s# - Step 3:
i = 4
→ Prints o# - Final Output:
W#s#o#
For b = 6:
- Range:
range(0, 6, 2)
→ Includes 0, 2, 4 - Step 1:
i = 0
→ Prints W# - Step 2:
i = 2
→ Prints s# - Step 3:
i = 4
→ Prints o# - Final Output:
W#s#o#
The code provided below is intended to swap the first and last elements of a given tuple. However, there are syntax and logical errors in the code. Rewrite it after removing all errors. Underline all the corrections made.
def swap_first_last(tup)
if len(tup) < 2:
return tup
new_tup = (tup[-1],) + tup[1:-1] + (tup[0])
return new_tup
result = swap_first_last((1, 2, 3, 4))
print("Swapped tuple: " result)
Answer
def swap_first_last(tup) # Error 1
if len(tup) < 2:
return tup # Error 2
new_tup = (tup[-1],) + tup[1:-1] + (tup[0]) # Error 3
return new_tup
result = swap_first_last((1, 2, 3, 4))
print("Swapped tuple: " result) # Error 4
Error 1 — The function header is missing a colon (:) at the end, which is required to define a function in Python.
Error 2 — The return
statement needs to be indented because it is the part of the if
block.
Error 3 — Comma should be added after tup[0]
to ensure it is treated as a tuple. Without the comma, (tup[0])
is treated as an integer.
Error 4 — Comma should be added in the print statement to properly separate the string from the variable.
The corrected code is:
def swap_first_last(tup):
if len(tup) < 2:
return tup
new_tup = (tup[-1],) + tup[1:-1] + (tup[0],)
return new_tup
result = swap_first_last((1, 2, 3, 4))
print("Swapped tuple: ", result)
What constraint should be applied on a table column so that duplicate values are not allowed in that column, but NULL is allowed.
Answer
To prevent duplicate values in a table column while allowing NULL values, we should use the UNIQUE
constraint. The UNIQUE
constraint ensures that all values in the specified column are different, but it permits multiple NULLs since NULL is considered distinct from any other value.
What constraint should be applied on a table column so that NULL is not allowed in that column, but duplicate values are allowed.
Answer
To ensure that NULL values are not allowed in a table column while allowing duplicate values, we should use the NOT NULL
constraint. The NOT NULL
constraint ensures that every entry in the specified column must have a value (i.e., it cannot be NULL), but it allows duplicate values.
Write an SQL command to remove the Primary Key constraint from a table, named MOBILE. M_ID is the primary key of the table.
Answer
ALTER TABLE MOBILE
DROP PRIMARY KEY;
Write an SQL command to make the column M_ID the Primary Key of an already existing table, named MOBILE.
Answer
ALTER TABLE MOBILE
ADD PRIMARY KEY (M_ID);
List one advantage and one disadvantage of star topology.
Answer
Advantage
Ease of service — Network extension is easy.
Disadvantage
Central node dependency — Failure of switch/hub results in failure of the network.
Expand the term SMTP. What is the use of SMTP ?
Answer
SMTP stands for Simple Mail Transfer Protocol.
It is the standard protocol for sending emails across the Internet.
Write a Python function that displays all the words containing @cmail from a text file "Emails.txt".
Answer
Suppose the file "Emails.txt" contains the following text:
jagan@cmail.com
aliya@gmail.com
ali@cmail.net
sara@cmail.edu
mahi@yahoo.com.
def display_words():
file = open("Emails.txt", 'r')
content = file.read()
words = content.split()
for word in words:
if '@cmail' in word:
print(word, end = ' ')
file.close()
display_words()
jagan@cmail.com ali@cmail.net sara@cmail.edu
Write a Python function that finds and displays all the words longer than 5 characters from a text file "Words.txt".
Answer
Suppose the file "Words.txt" contains the following text:
The quick brown fox jumps over the lazy dog and runs swiftly through the forest
def display():
file = open("Words.txt", 'r')
content = file.read()
words = content.split()
for word in words:
if len(word) > 5:
print(word, end = ' ')
file.close()
display()
swiftly through forest
You have a stack named BooksStack that contains records of books. Each book record is represented as a list containing book_title, author_name, and publication_year.
Write the following user-defined functions in Python to perform the specified operations on the stack BooksStack:
push_book(BooksStack, new_book): This function takes the stack BooksStack and a new book record new_book as arguments and pushes the new book record onto the stack.
pop_book(BooksStack): This function pops the topmost book record from the stack and returns it. If the stack is already empty, the function should display "Underflow".
peep(BookStack): This function displays the topmost element of the stack without deleting it. If the stack is empty, the function should display 'None'.
Answer
1.
def push_book(BooksStack, new_book):
BooksStack.append(new_book)
2.
def pop_book(BooksStack):
if not BooksStack:
print("Underflow")
else:
return(BookStack.pop())
3.
def peep(BooksStack):
if not BooksStack:
print("None")
else:
print(BookStack[-1])
Write the definition of a user-defined function push_even(N)
which accepts a list of integers in a parameter N
and pushes all those integers which are even from the list N
into a Stack named EvenNumbers
.
Write function pop_even() to pop the topmost number from the stack and return it. If the stack is already empty, the function should display "Empty".
Write function Disp_even() to display all element of the stack without deleting them. If the stack is empty, the function should display 'None'.
For example: If the integers input into the list VALUES
are: [10, 5, 8, 3, 12]
Then the stack EvenNumbers
should store: [10, 8, 12]
Answer
def push_even(N):
EvenNumbers = []
for num in N:
if num % 2 == 0:
EvenNumbers.append(num)
return EvenNumbers
VALUES = []
for i in range(5):
VALUES.append(int(input("Enter an integer: ")))
EvenNumbers = push_even(VALUES)
def pop_even():
if not EvenNumbers:
print("Underflow")
else:
print(EvenNumbers.pop())
pop_even()
def Disp_even():
if not EvenNumbers:
print("None")
else:
print(EvenNumbers[-1])
Disp_even()
Enter an integer: 10
Enter an integer: 5
Enter an integer: 8
Enter an integer: 3
Enter an integer: 12
12
8
Predict the output of the following code:
d = {"apple": 15, "banana": 7, "cherry": 9}
str1 = ""
for key in d:
str1 = str1 + str(d[key]) + "@" + "\n"
str2 = str1[:-1]
print(str2)
Answer
15@
7@
9@
Initialization:
- A dictionary
d
is created with three key-value pairs. - An empty string
str1
is initialized.
Iteration:
The
for
loop iterates over each key in the dictionary:
- For "apple":
str1
becomes "15@\n" (value 15 followed by "@" and a newline). - For "banana":
str1
becomes "15@\n7@\n" (adding value 7 followed by "@" and a newline). - For "cherry":
str1
becomes "15@\n7@\n9@\n" (adding value 9 followed by "@" and a newline).
The line
str2 = str1[:-1]
removes the last newline character fromstr1
, resulting instr2
being "15@\n7@\n9@".Finally,
print(str2)
outputs the content ofstr2
.
Predict the output of the following code:
line = [4, 9, 12, 6, 20]
for I in line:
for j in range(1, I%5):
print(j, '#', end="")
print()
Answer
1 #2 #3 #
1 #2 #3 #
1 #
The list
line
contains the values [4, 9, 12, 6, 20].The outer loop iterates over each element (
I
) in theline
list.First Iteration (I = 4):
I % 5 = 4 % 5 = 4
Inner Loop Range:range(1, 4)
→ This generates the numbers 1, 2, 3.
Output:1 #2 #3 #
Second Iteration (I = 9):
I % 5 = 9 % 5 = 4
Inner Loop Range:range(1, 4)
→ This generates the numbers 1, 2, 3.
Output:1 #2 #3 #
Third Iteration (I = 12):
I % 5 = 12 % 5 = 2
Inner Loop Range:range(1, 2)
→ This generates the number 1.
Output:1 #
Fourth Iteration (I = 6):
I % 5 = 6 % 5 = 1
Inner Loop Range:range(1, 1)
→ This generates no numbers (empty range).
Print: No output for this iteration (just a new line is printed).Fifth Iteration (I = 20):
I % 5 = 20 % 5 = 0
Inner Loop Range:range(1, 0)
→ This generates no numbers (empty range).
Print: No output for this iteration (just a new line is printed).
The final output of the code will be:
1 #2 #3 #
1 #2 #3 #
1 #
Consider the table ORDERS as given below
O_Id | C_Name | Product | Quantity | Price |
---|---|---|---|---|
1001 | Jitendra | Laptop | 1 | 12000 |
1002 | Mustafa | Smartphone | 2 | 10000 |
1003 | Dhwani | Headphone | 1 | 1500 |
Note: The table contains many more records than shown here.
A) Write the following queries:
(I) To display the total Quantity for each Product, excluding Products with total Quantity less than 5.
(II) To display the orders table sorted by total price in descending order.
(III) To display the distinct customer names from the Orders table.
(IV) Display the sum of Price of all the orders for which the quantity is null.
OR
B) Write the output
(I) Select c_name, sum(quantity) as total_quantity from orders group by c_name;
(II) Select * from orders where product like '%phone%';
(III) Select o_id, c_name, product, quantity, price from orders where price between 1500 and 12000;
(IV) Select max(price) from orders;
Answer
(A)
(I)
SELECT Product, SUM(Quantity)
FROM ORDERS
GROUP BY Product
HAVING SUM(Quantity) >= 5;
(II)
SELECT *
FROM ORDERS
ORDER BY Price DESC;
(III)
SELECT DISTINCT C_Name
FROM ORDERS;
(IV)
SELECT SUM(Price) AS Total_Price
FROM ORDERS
WHERE Quantity IS NULL;
OR
(B)
(I) Select c_name, sum(quantity) as total_quantity from orders group by c_name;
+----------+----------------+
| c_name | total_quantity |
+----------+----------------+
| Jitendra | 1 |
| Mustafa | 2 |
| Dhwani | 1 |
+----------+----------------+
(II) Select * from orders where product like '%phone%';
+------+---------+------------+----------+-------+
| O_Id | C_Name | Product | Quantity | Price |
+------+---------+------------+----------+-------+
| 1002 | Mustafa | Smartphone | 2 | 10000 |
| 1003 | Dhwani | Headphone | 1 | 1500 |
+------+---------+------------+----------+-------+
(III) Select o_id, c_name, product, quantity, price from orders where price between 1500 and 12000;
+------+----------+------------+----------+-------+
| o_id | c_name | product | quantity | price |
+------+----------+------------+----------+-------+
| 1001 | Jitendra | Laptop | 1 | 12000 |
| 1002 | Mustafa | Smartphone | 2 | 10000 |
| 1003 | Dhwani | Headphone | 1 | 1500 |
+------+----------+------------+----------+-------+
(IV) Select max(price) from orders;
+------------+
| max(price) |
+------------+
| 12000 |
+------------+
A csv file "Happiness.csv" contains the data of a survey. Each record of the file contains the following data:
- Name of a country
- Population of the country
- Sample Size (Number of persons who participated in the survey in that country)
- Happy (Number of persons who accepted that they were Happy)
For example, a sample record of the file may be:
[‘Signiland’, 5673000, 5000, 3426]
Write the following Python functions to perform the specified operations on this file:
(I) Read all the data from the file in the form of a list and display all those records for which the population is more than 5000000.
(II) Count the number of records in the file.
Answer
Let the "Happiness.csv" file contain the following data:
Signiland, 5673000, 5000, 3426
Happiland, 4500000, 4000, 3200
Joyland, 8000000, 6000, 5000
Cheerland, 3000000, 3500, 2500
(I)
def show():
file = open("Happiness.csv",'r')
records=csv.reader(file)
for i in records:
if int(i[1])>5000000:
print(i)
file.close()
['Signiland', '5673000', '5000', '3426']
['Joyland', '8000000', '6000', '5000']
(II)
def Count_records():
f = open("Happiness.csv",'r')
records=csv.reader(f)
count=0
for i in records:
count+=1
print(count)
f.close()
4
Saman has been entrusted with the management of Law University Database. He needs to access some information from FACULTY and COURSES tables for a survey analysis. Help him extract the following information by writing the desired SQL queries as mentioned below.
Table: FACULTY
F_ID | FName | LName | Hire_Date | Salary |
---|---|---|---|---|
102 | Amit | Mishra | 12-10-1998 | 12000 |
103 | Nitin | Vyas | 24-12-1994 | 8000 |
104 | Rakshit | Soni | 18-5-2001 | 14000 |
105 | Rashmi | Malhotra | 11-9-2004 | 11000 |
106 | Sulekha | Srivastava | 5-6-2006 | 10000 |
Table: COURSES
C_ID | F_ID | CName | Fees |
---|---|---|---|
C21 | 102 | Grid Computing | 40000 |
C22 | 106 | System Design | 16000 |
C23 | 104 | Computer Security | 8000 |
C24 | 106 | Human Biology | 15000 |
C25 | 102 | Computer Network | 20000 |
C26 | 105 | Visual Basic | 6000 |
(I) To display complete details (from both the tables) of those Faculties whose salary is less than 12000.
(II) To display the details of courses whose fees is in the range of 20000 to 50000 (both values included).
(III) To increase the fees of all courses by 500 which have "Computer" in their Course names.
(IV)
(A) To display names (FName and LName) of faculty taking System Design.
OR
(B) To display the Cartesian Product of these two tables.
Answer
(I)
SELECT * FROM FACULTY, COURSES
WHERE Salary < 12000 AND FACULTY.F_ID = COURSES.F_ID;
(II)
SELECT *
FROM COURSES
WHERE Fees BETWEEN 20000 AND 50000;
(III)
UPDATE COURSES
SET Fees = Fees + 500
WHERE CName LIKE '%Computer%';
(IV)
(A)
SELECT FName, LName
FROM FACULTY, COURSES
WHERE CName = 'System Design' AND
FACULTY.F_ID = COURSES.F_ID;
OR
(B)
SELECT *
FROM FACULTY, COURSES;
A table, named STATIONERY, in ITEMDB database, has the following structure:
Field | Type |
---|---|
itemNo | int(11) |
itemName | varchar(15) |
price | float |
qty | int(11) |
Write the following Python function to perform the specified operation:
AddAndDisplay(): To input details of an item and store it in the table STATIONERY. The function should then retrieve and display all records from the STATIONERY table where the Price is greater than 120.
Assume the following for Python-Database connectivity:
Host: localhost, User: root, Password: Pencil
Answer
import mysql.connector
def AddAndDisplay():
db = mysql.connector.connect(
host="localhost",
user="root",
password="Pencil",
database="ITEMDB"
)
cursor = db.cursor()
item_no = int(input("Enter Item Number: "))
item_name = input("Enter Item Name: ")
price = float(input("Enter Price: "))
qty = int(input("Enter Quantity: "))
insert_query = "INSERT INTO STATIONERY VALUES ({}, {}, {}, {})"
insert_query = insert_query.format(item_no, item_name, price, qty)
cursor.execute(insert_query)
db.commit()
select_query = "SELECT * FROM STATIONERY WHERE price > 120"
cursor.execute(select_query)
results = cursor.fetchall()
for record in results:
print(record)
AddAndDisplay()
Surya is a manager working in a recruitment agency. He needs to manage the records of various candidates. For this, he wants the following information of each candidate to be stored:
- Candidate_ID – integer
- Candidate_Name – string
- Designation – string
- Experience – float
You, as a programmer of the company, have been assigned to do this job for Surya.
(I) Write a function to input the data of a candidate and append it in a binary file.
(II) Write a function to update the data of candidates whose experience is more than 10 years and change their designation to "Senior Manager".
(III) Write a function to read the data from the binary file and display the data of all those candidates who are not "Senior Manager".
Answer
(I)
import pickle
def input_candidates():
candidates = []
n = int(input("Enter the number of candidates you want to add: "))
for i in range(n):
candidate_id = int(input("Enter Candidate ID: "))
candidate_name = input("Enter Candidate Name: ")
designation = input("Enter Designation: ")
experience = float(input("Enter Experience (in years): "))
candidates.append([candidate_id, candidate_name, designation,
experience])
return candidates
candidates_list = input_candidates()
def append_candidate_data(candidates):
with open('candidates.bin', 'ab') as file:
for candidate in candidates:
pickle.dump(candidate, file)
print("Candidate data appended successfully.")
append_candidate_data(candidates_list)
(II)
import pickle
def update_senior_manager():
updated_candidates = []
try:
with open('candidates.bin', 'rb') as file:
while True:
try:
candidate = pickle.load(file)
if candidate[3] > 10: # If experience > 10 years
candidate[2] = 'Senior Manager'
updated_candidates.append(candidate)
except EOFError:
break # End of file reached
except FileNotFoundError:
print("No candidate data found. Please add candidates first.")
return
with open('candidates.bin', 'wb') as file:
for candidate in updated_candidates:
pickle.dump(candidate, file)
print("Candidates updated to Senior Manager where applicable.")
update_senior_manager()
(III)
import pickle
def display_non_senior_managers():
try:
with open('candidates.bin', 'rb') as file:
while True:
try:
candidate = pickle.load(file)
if candidate[2] != 'Senior Manager': # Check if not Senior Manager
print(f"Candidate ID: {candidate[0]}")
print(f"Candidate Name: {candidate[1]}")
print(f"Designation: {candidate[2]}")
print(f"Experience: {candidate[3]}")
print("--------------------")
except EOFError:
break # End of file reached
except FileNotFoundError:
print("No candidate data found. Please add candidates first.")
display_non_senior_managers()
Event Horizon Enterprises is an event planning organization. It is planning to set up its India campus in Mumbai with its head office in Delhi. The Mumbai campus will have four blocks/buildings - ADMIN, FOOD, MEDIA, DECORATORS. You, as a network expert, need to suggest the best network-related solutions for them to resolve the issues/problems mentioned in points (I) to (V), keeping in mind the distances between various blocks/buildings and other given parameters.
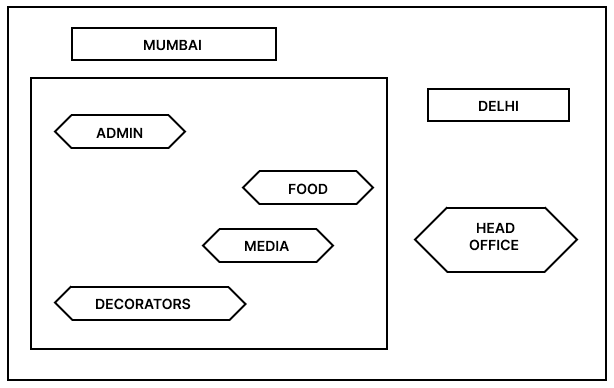
Block to Block distances (in Mtrs.)
From | To | Distance |
---|---|---|
ADMIN | FOOD | 42 m |
ADMIN | MEDIA | 96 m |
ADMIN | DECORATORS | 48 m |
FOOD | MEDIA | 58 m |
FOOD | DECORATORS | 46 m |
MEDIA | DECORATORS | 42 m |
Distance of Delhi Head Office from Mumbai Campus = 1500 km
Number of computers in each of the blocks/Center is as follows:
ADMIN | 30 |
FOOD | 18 |
MEDIA | 25 |
DECORATORS | 20 |
DELHI HEAD OFFICE | 18 |
(I) Suggest the most appropriate location of the server inside the MUMBAI campus. Justify your choice.
(II) Which hardware device will you suggest to connect all the computers within each building?
(III) Draw the cable layout to efficiently connect various buildings within the MUMBAI campus. Which cable would you suggest for the most efficient data transfer over the network?
(IV) Is there a requirement of a repeater in the given cable layout? Why/ Why not?
(V)
(A) What would be your recommendation for enabling live visual communication between the Admin Office at the Mumbai campus and the DELHI Head Office from the following options:
a) Video Conferencing
b) Email
c) Telephony
d) Instant Messaging
OR
(B) What type of network (PAN, LAN, MAN, or WAN) will be set up among the computers connected in the MUMBAI campus ?
Answer
(I) The most appropriate location for the server inside the MUMBAI campus is ADMIN block because it has maximum number of computers.
(II) Switch is used to connect all the computers within each building.
(III) Cable layout connecting various buildings within the MUMBAI campus is shown below:
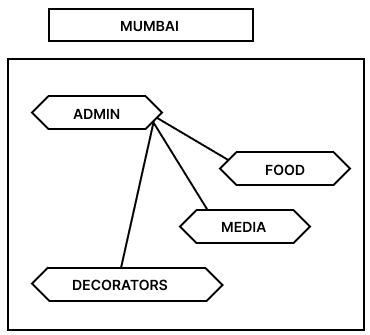
For the most efficient data transfer over the network, optical fiber cables are recommended.
(IV) There is no requirement for a repeater as optical fiber cables can transmit signals over longer distances.
(V)
(A) Video Conferencing
Video conferencing is recommended for live communication between the Mumbai and Delhi offices due to its real-time interaction and flexibility making it ideal for effective discussions across distant locations.
OR
(B) The type of network that will be set up among the computers connected in the MUMBAI campus is LAN (Local Area Network).