What will be the output of the function given below?
>>import math
>>abs(math.sqrt(25))
- Error
- 5
- -5
- 5.0
Answer
5.0
Reason — The given code calculates the square root of 25 using the math.sqrt()
function from the math
module, resulting in 5.0 since sqrt()
returns a floating-point number. Applying abs()
to a positive number like 5.0 doesn't alter the value, thus the final output remains 5.0.
A ............... function allows us to write a single record into each row of a CSV file.
Answer
A writerow
function allows us to write a single record into each row of a CSV file.
Does the Primary key of a table accept null or duplicate value? Justify.
Answer
A primary key in a table does not accept null or duplicate values. A primary key is a set of one or more attributes/fields that uniquely identifies a tuple/row in a table. It must contain unique values, i.e., non-redundant, and cannot be re-declared or left null.
Identify the Domain name and URL from the following: http://www.income.in/home.aboutus.html
Answer
The domain name is www.income.in
indicating the network domain hosting the website, while the URL is http://www.income.in/home.aboutus.html
, specifying the specific page or resource within that domain.
Which protocol is used to create a connection with a remote machine?
Answer
Telnet protocol is used to create a connection with a remote machine.
Which of the following cannot be returned by random.randrange(4) ?
- 0
- 3
- 2.3
- None of these
Answer
2.3
Reason — The random.randrange(4)
function returns a randomly selected integer from the range [0, 1, 2, 3], which includes integers only. It cannot return a floating-point number like 2.3.
Find errors (if any) in the following code and rewrite the code underlining each correction:
x = int("Enter value of x:")
for in range[0, 10]:
if x = y
print("They are equal")
else:
Print("They are unequal")
Answer
x = int("Enter value of x:") #Error 1
for in range[0, 10]: #Error 2
if x = y #Error 3
print("They are equal")
else:
Print("They are unequal") #Error 4
Error 1 — The input()
function is missing from the code to prompt the user for input.
Error 2 — The syntax of the range()
function uses parentheses () rather than square brackets [] and the variable y
is missing in the for
loop declaration (for y in range(0, 10):)
.
Error 3 — The comparison operator in the if
statement has = (assignment) instead of == (equality comparison) and colon (:) is missing at the end.
Error 4 — The print function should be written with a lowercase 'p' for proper syntax.
The corrected code is:
x = int(input("Enter value of x:"))
for y in range(0, 10):
if x == y:
print("They are equal")
else:
print("They are unequal")
Mr. William wants to remove all the rows from table INVENTORY to release the storage space but he does not want to remove the structure of the table. What MySQL statement should he use?
Answer
DELETE FROM INVENTORY;
This statement will delete all rows from the INVENTORY table, freeing up storage space, but it will not remove the structure or schema of the table.
A computer or a device that requests resources or data from the server is called ............... .
Answer
A computer or device that requests resources or data from a server is called client.
The readlines() method returns ............... (a list of lines/a list of single characters).
Answer
The readlines()
method returns a list of lines from a file.
Write two advantages of using an Optical Fibre cable over an Ethernet cable to connect two service stations which are 200 m away from each other.
Answer
The two advantages of using an Optical Fibre cable over an Ethernet cable are as follows:
- Optical Fibre cables provide higher bandwidth compared to Ethernet cables.
- Optical Fibre cables can transmit data over longer distances without experiencing signal degradation or loss compared to Ethernet cables.
What is the use of "w" in file handling?
Answer
In file handling, the "w" mode is used to open a file for writing. If the file exists, it will be overwritten. If the file does not exist, a new file will be created with the specified name.
Rewrite the following SQL statement after correcting the error(s). Underline the corrections made.
INSERT IN STUDENT (RNO, MARKS)
VALUE (5, 78.5);
Answer
INSERT IN STUDENT (RNO, MARKS) -- Error 1
VALUE (5, 78.5); -- Error 2
Error 1 — The correct syntax in SQL for inserting data into a table is "INSERT INTO", not "INSERT IN".
Error 2 — The correct keyword used in SQL to specify the values being inserted into the table is "VALUES", not "VALUE".
The corrected code is:
INSERT INTO STUDENT(RNO, MARKS)
VALUES (5, 78.5);
Write a function Push() which takes number as argument and add in a stack "MyValue".
MyValue = []
def Push(number):
MyValue.append(number)
print(number, "pushed to the stack.")
Push(5)
Push(10)
Push(15)
5 pushed to the stack.
10 pushed to the stack.
15 pushed to the stack.
Write the output of the following code:
for i in range(5):
print(i)
Answer
0
1
2
3
4
Expand the following:
(a) LIFO
(b) FIFO
Answer
(a) LIFO — Last-In First-Out
(b) FIFO — First-In First-Out
Assertion (A): Program should check for Overflow condition before executing Push operation on stack and similarly check for Underflow condition before executing Pop operation.
Reasoning (R): In stack, Underflow condition signifies that there is no element available in the stack while Overflow condition means no further element can be pushed in the stack.
- Both A and R are true and R is the correct explanation of A.
- Both A and R are true but R is not the correct explanation of A.
- A is true but R is false.
- A is false but R is true.
Answer
Both A and R are true and R is the correct explanation of A.
Explanation
It is essential to check for overflow conditions before executing a Push operation on a stack to ensure that the stack has enough space to accommodate the new element. Similarly, checking for underflow conditions before executing a Pop operation is necessary to prevent deleting elements from an empty stack. An underflow condition indicates an empty stack, while an overflow condition indicates a stack that has reached its maximum capacity and cannot accept more elements.
Assertion (A): ('x' and 'y' or not 7) is a valid expression in Python.
Reason (R): In Python, strings and numbers can be treated as Boolean values.
- Both A and R are true and R is the correct explanation of A.
- Both A and R are true but R is not the correct explanation of A.
- A is true but R is false.
- A is false but R is true.
Answer
Both A and R are true and R is the correct explanation of A.
Explanation
In the expression ('x' and 'y' or not 7), both 'x' and 'y' are non-empty string literals, hence they are evaluated as True, and 7 is a non-zero number, also evaluated as True. Therefore, this expression results in 'y'. In Python, when evaluating expressions in a Boolean context, such as conditions in if statements, non-empty strings, non-zero numbers, and non-None values are considered True, while empty strings, numeric zero (0), and None are considered False.
Define Primary Key.
Answer
A primary key is a set of one or more attributes or fields that uniquely identify a tuple or row in a table.
Define Foreign Key.
Answer
A foreign key is a non-key attribute whose value is derived from the primary key of another table in a database.
Define Alternate Key.
Answer
A candidate key that is not the primary key is called an alternate key.
Define Candidate Key.
Answer
All attribute combinations inside a relation that can serve as primary key are candidate keys as they are candidates for the primary key position.
Write push(rollno)
and pop()
method in Python:
push(rollno)
— to add roll number in Stack
pop()
— to remove roll number from Stack
stack = []
def push(rollno):
stack.append(rollno)
print("Roll number", rollno, "added to the stack.")
def pop():
if not is_empty():
rollno = stack.pop()
print("Roll number", rollno, "removed from the stack.")
else:
print("Stack is empty, cannot perform pop operation.")
def is_empty():
return len(stack) == 0
push(11)
push(12)
push(13)
pop()
pop()
Roll number 11 added to the stack.
Roll number 12 added to the stack.
Roll number 13 added to the stack.
Roll number 13 removed from the stack.
Roll number 12 removed from the stack.
Write the output of the following code with justification if the contents of the file "VIVO.txt" are:
"Welcome to Python Programming!"
F1 = open("VIVO.txt", "r")
size = len(F1.read())
print(size)
data = F1.read(5)
print(data)
Answer
The given code opens a file named "VIVO.txt" in read mode and reads its entire content into a variable F1
. It then calculates the length of this content using len()
and prints the result, which is 30 characters long. However, when it tries to read the next 5 characters from the file using F1.read(5)
, no characters are left to read since the file pointer is at the end the file. As a result, data remains an empty string.
30
Simrat has written a code to input an integer and display its first 10 multiples (from 1 to 10). Her code is having errors. Rewrite the correct code and underline the corrections made.
n = int(input(Enter an integer:))
for i in range(1, 11):
m = n * j
print(m; End = '')
Answer
n = int(input(Enter an integer:)) # Error 1
for i in range(1, 11):
m = n * j # Error 2
print(m; End = '') # Error 3
Error 1 — The quotes around the input message are missing.
Error 2 — The for loop body lacks proper indentation, and the loop variable should be 'i' instead of 'j'.
Error 3 — In Python, a comma (,) is used to separate arguments not semicolon (;), and the first letter of end in the print() function should be lowercase.
The corrected code is :
n = int(input("Enter an integer:"))
for i in range(1, 11):
m = n * i
print(m, end = ' ')
Given is a Python string declaration:
voice = "Python for All Learners"
Write the output of:
print(voice[20 : : -2])
Answer
ere l o otP
voice[20 : : -2]: Starting from index 20, the expression goes till the end of the string in reverse order with a step size of -2.
Write the output of the code given below:
d1 = {"A" : "Avocado", "B" : "Banana"}
d2 = {'B' : 'Bag', 'C' : 'Couch'}
d2.update(d1)
print(len(d2))
Answer
3
The code initialize two dictionaries, d1
and d2
, with key-value pairs. Then, it updates d2
with the key-value pairs from d1
, overwriting the value of key 'B' in d2
with 'Banana' from d1
and adding the new key-value pair 'A' mapped to 'Avocado'. Finally, it prints the length of d2
, which is 3 since d2
now has three key-value pairs after the update operation.
What will the following snippet print?
L5 = [1500, 1800, 1600, 1200, 1900]
begin = 1
sum = 0
for c in range(begin, 4):
sum = sum + L5[c]
print(c, ':', sum)
sum = sum + L5[0] * 10
print("sum is", sum)
Answer
1 : 1800
sum is 16800
2 : 18400
sum is 33400
3 : 34600
sum is 49600
The given code initializes a list L5
with five elements. It then sets begin
to 1 and sum
to 0. The loop iterates for values 1, 2, and 3. In each iteration, the code adds the element at index c
of L5
to sum
, prints the c
and updated sum
, and then adds ten times the first element of L5
to sum
and prints the value of sum
.
Consider the following two SQL commands with reference to a table, named MOVIES, having a column named Director:
(a) Select Distinct Director from MOVIES;
(b) Select Director from MOVIES;
- In which case will these two commands produce the same result?
- In which case will these two commands produce different results?
Answer
The two SQL commands will produce the same result when there are no duplicate values in the Director column of the MOVIES table. If every Director value in the table is unique, both commands will return the same set of distinct Director values.
The two SQL commands will produce different results when there are duplicate values in the Director column of the MOVIES table. The first command with DISTINCT will only return unique Director values, omitting duplicates, whereas the second command without DISTINCT will return all Director values, including duplicates.
Write a function WordsList(S), where S is a string. The function returns a list, named Words, that stores all the words from the string which contain 'r'.
For example, if S is "Dew drops were shining in the morning", then the list Words should be: ['drops', 'were', 'morning']
def WordsList(S):
words = S.split()
Words = []
for word in words:
if 'r' in word:
Words.append(word)
return Words
S = "Dew drops were shining in the morning"
print(WordsList(S))
['drops', 'were', 'morning']
In a database BANK, there are two tables with a sample data given below:
Table: EMPLOYEE
ENo | EName | Salary | Zone | Age | Grade | Dept |
---|---|---|---|---|---|---|
1 | Mona | 70000 | East | 40 | A | 10 |
2 | Mukhtar | 71000 | West | 45 | B | 20 |
3 | Nalini | 60000 | East | 26 | A | 10 |
4 | Sanaj | 65000 | South | 36 | A | 20 |
5 | Surya | 58000 | North | 30 | B | 30 |
Table: DEPARTMENT
Dept | DName | HOD |
---|---|---|
10 | Computers | 1 |
20 | Economics | 2 |
30 | English | 5 |
(a) To display ENo, EName, Salary and corresponding DName of all the employees whose age is between 25 and 35 (both values inclusive).
(b) To display DName and corresponding EName from the tables DEPARTMENT and EMPLOYEE (Hint: HOD of DEPARTMENT table should be matched with ENo of EMPLOYEE table for getting the desired result).
(c) To display EName, Salary, Zone and Income Tax (Note: Income tax to be calculated as 30% of salary) of all the employees with appropriate column headings.
Answer
(a)
SELECT E.ENO, E.ENAME, E.SALARY, D.DNAME
FROM EMPLOYEE E, DEPARTMENT D
WHERE E.DEPT = D.DEPT AND (E.AGE BETWEEN 25 AND 35);
+-----+--------+--------+-----------+
| ENO | ENAME | SALARY | DNAME |
+-----+--------+--------+-----------+
| 3 | NALINI | 60000 | COMPUTERS |
| 5 | SURYA | 58000 | ENGLISH |
+-----+--------+--------+-----------+
(b)
SELECT D.DNAME, E.ENAME
FROM EMPLOYEE E, DEPARTMENT D
WHERE E.ENO = D.HOD;
+-----------+---------+
| DNAME | ENAME |
+-----------+---------+
| COMPUTERS | MONA |
| ECONOMICS | MUKHTAR |
| ENGLISH | SURYA |
+-----------+---------+
(c)
SELECT ENAME, SALARY, ZONE, (SALARY * 30/100) AS INCOME_TAX
FROM EMPLOYEE;
+---------+--------+-------+------------+
| ENAME | SALARY | ZONE | INCOME_TAX |
+---------+--------+-------+------------+
| MONA | 70000 | EAST | 21000.0000 |
| MUKHTAR | 71000 | WEST | 21300.0000 |
| NALINI | 60000 | EAST | 18000.0000 |
| SANAJ | 65000 | SOUTH | 19500.0000 |
| SURYA | 58000 | NORTH | 17400.0000 |
+---------+--------+-------+------------+
Write a function begEnd() in Python to read lines from text file 'TESTFILE.TXT' and display the first and the last character of every line of the file (ignoring the leading and trailing white space characters).
Example: If the file content is as follows:
An apple a day keeps the doctor away.
We all pray for everyone's safety
A marked difference will come in our country.
Then begEnd () function should display the output as: A. Wy A.
def begEnd():
file = open('TESTFILE.TXT', 'r')
f = file.readlines()
for line in f:
line = line.strip()
if line:
first_char = line[0]
last_char = line[-1]
print(first_char + last_char, end=' ')
begEnd()
A. Wy A.
Write a function reverseFile() in Python to read lines from text file 'TESTFILE.TXT' and display the file content in reverse order so that the last line is displayed first and the first line is displayed at the end.
def reverseFile():
file = open('TESTFILE.TXT', 'r')
lines = file.readlines()
line_count = len(lines)
for i in range(line_count - 1, -1, -1):
print(lines[i].strip())
reverseFile()
A marked difference will come in our country.
We all pray for everyone's safety
An apple a day keeps the doctor away.
Consider the following table STUDENT.
NO | NAME | AGE | DEPARTMENT | FEE | SEX |
---|---|---|---|---|---|
1 | PANKAJ | 24 | COMPUTER | 120 | M |
2 | SHALINI | 21 | HISTORY | 200 | F |
3 | SANJAY | 22 | HINDI | 300 | M |
4 | SUDHA | 25 | HISTORY | 400 | F |
Write a Python code to search a record as per given NO (number) using MySQL connectivity and print the data.
import mysql.connector
mydb = mysql.connector.connect(host = "localhost",
user = "root",
passwd = "tiger",
database = "kboat_cbse_12")
mycursor = mydb.cursor()
NO = int(input("Enter the number to search: "))
mycursor.execute("SELECT * FROM STUDENT WHERE NO = {}".format(NO))
myrecord = mycursor.fetchone()
if myrecord != None:
print(myrecord)
else:
print("No such student found")
Enter the number to search: 2
(2, 'SHALINI', 21, 'HISTORY', 200, 'F')
Write a Python code to insert a new record as per given table Student (No, Name, Age, Department, Fee, Sex) using MySQL connectivity.
import mysql.connector
mydb = mysql.connector.connect(host = "localhost",
user = "root",
passwd = "kboat123",
database = "kboat_cbse_12")
mycursor = mydb.cursor()
mycursor.execute("INSERT INTO STUDENT VALUES(5, 'ANANYA', 23, 'COMPUTER', 450, 'F')")
mydb.commit()
print("Records added")
mydb.close()
Records added
A binary file "salary.Dat" has structure [employee id, employee name, salary]. Write a function countrec() in Python that would read contents of the file "salary.Dat" and display the details of those employees whose salary is above 20000.
Answer
Let the "salary.dat" file contain following sample data:
[[101, 'Aditi', 25000], [102, 'Jatin', 19000], [103, 'Minaz', 28000]]
import pickle
def countrec(file_name):
f1 = open(file_name, 'rb')
while True:
try:
employees = pickle.load(f1)
for employee in employees:
if (employee[2] > 20000):
print("Employee ID:", employee[0], "Name:", employee[1], "Salary:", employee[2])
except EOFError:
break
f1.close()
countrec("salary.dat")
Employee ID: 101 Name: Aditi Salary: 25000
Employee ID: 103 Name: Minaz Salary: 28000
A departmental store "ABC" is considering maintaining their inventory using SQL to store data and maintain basic transactions. As a database manager, Mehak has to create two tables as Customer & Transaction:
Table: CUSTOMER
CNo | CNAME | ADDRESS |
---|---|---|
101 | Richa Jain | Delhi |
102 | Surbhi Sinha | Chennai |
103 | Lisa Thomas | Bengaluru |
104 | Imran Ali | Delhi |
105 | Roshan Singh | Chennai |
Table: TRANSACTION
Dept | CNO | AMOUNT | TYPE | DOT |
---|---|---|---|---|
T001 | 101 | 1500 | Credit | 2017-11-23 |
T002 | 103 | 2000 | Debit | 2017-05-12 |
T003 | 102 | 3000 | Credit | 2017-06-10 |
1004 | 103 | 12000 | Credit | 2017-09-12 |
T005 | 101 | 1000 | Debit | 2017-09-05 |
(i) Identify the attribute best suited to be declared as a Primary key in customer table.
(ii) Help Mehak to display details of all transactions of TYPE Credit from Table TRANSACTION.
(iii) Mehak wants to display all CNO, CNAME and DOT (date of transaction) of those CUSTOMERS from tables CUSTOMER and TRANSACTION who have done transactions more than or equal to 2000 for the Balance Sheet.
(iv) Mehak wants to display the last date of transaction (DOT) from the table TRANSACTION for the customer having CNO as 103. Which command will she use?
Answer
(i) The attribute best suited to be declared as a Primary Key in the CUSTOMER table is CNo (Customer Number). It uniquely identifies each customer in the table.
(ii)
SELECT * FROM TRANSACTION
WHERE TYPE = "Credit";
+------+-----+--------+--------+------------+
| DEPT | CNO | AMOUNT | TYPE | DOT |
+------+-----+--------+--------+------------+
| T001 | 101 | 1500 | Credit | 2017-11-23 |
| T003 | 102 | 3000 | Credit | 2017-06-10 |
| T004 | 103 | 12000 | Credit | 2017-09-12 |
+------+-----+--------+--------+------------+
(iii)
SELECT C.CNO, C.CNAME, T.DOT
FROM CUSTOMER C, TRANSACTION T
WHERE C.CNO = T.CNO AND T.AMOUNT >= 2000;
+-----+--------------+------------+
| CNO | CNAME | DOT |
+-----+--------------+------------+
| 103 | LISA THOMAS | 2017-05-12 |
| 102 | SURBHI SINHA | 2017-06-10 |
| 103 | LISA THOMAS | 2017-09-12 |
+-----+--------------+------------+
(iv) Mehak will use "MAX()" function to display the last date of transaction (DOT) from the table TRANSACTION for the customer having CNO as 103.
SELECT MAX(DOT) AS Last_Transaction_Date
FROM TRANSACTION
WHERE CNO = 103;
+-----------------------+
| Last_Transaction_Date |
+-----------------------+
| 2017-09-12 |
+-----------------------+
Sonia was writing a code to insert multiple rows in the cust.csv file. By mistake her younger sister removed some parts of it. Now she is totally confused about used module and other options:
from csv import writer
with ............... ("cust.csv", "a", newline = "\n") as f: #line 1
dt = writer(...............) #line 2
while True: #line 3
sno= int (input ("Enter Serial No: ") )
cust_name = input ("Enter customer name: ")
city = input ("Enter city: ")
amt = int (input ("Enter amount: ") )
dt. ............... ( [sno, cust name, city, amt] ) #line 4
print ("Record has been added.")
print ("Want to add more record?Type YES!!!")
ch = input() #line 5
ch = ch.upper()
if ch=="YES":
print("*************************")
else:
break
Attempt any 4 out of the given questions:
(i) Name the method Sonia should use in Line 1.
(ii) Which object Sonia should use to connect the file in Line 2?
(iii) What is the use of Line 3?
(iv) Name the method she should use in Line 4.
(v) What is the use of Line 5?
Answer
(i) Sonia should use the "open" method to connect to the "cust.csv" file in append mode.
(ii) Sonia should use the file object "f" to connect to the file.
(iii) Line 3 starts a while loop using while True:
. This creates an infinite loop that allows Sonia to repeatedly input customer data and add records to the CSV file until she chooses to stop.
(iv) Sonia should use the "writerow" method to write a single row (list of values) to the CSV file.
(v) Line 5 reads the Sonia's input for whether she want to add more records to the CSV file.
To provide telemedicine facility in a hilly state, a computer network is to be set up to connect hospitals in 6 small villages (V1, V2, ..., V6) to the base hospital (H) in the state capital. This is shown in the following diagram.
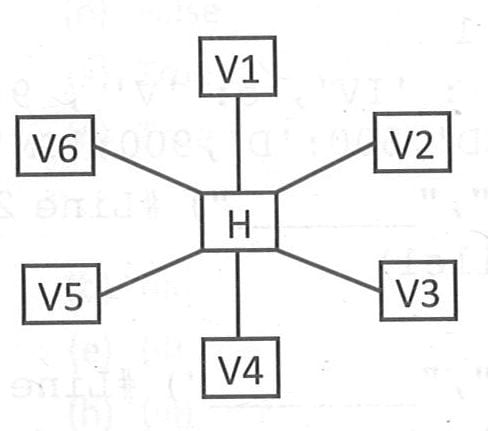
No village is more than 20 km away from the state capital. Imagine yourself as a computer consultant for this project and answer the following questions with justification:
(i) Out of the following, what kind of link should be provided to set up this network: Microwave Link, Radio Link, Wired Link?
(ii) Many a time doctors at the village hospital have to consult senior doctors at the base hospital. How should they contact them—through email, SMS, telephone or video conference?
(iii) Out of SMTP and POP3, which protocol is used to receive emails?
(iv) Expand the following terms:
(a) VoIP
(b) HTTPS
(v) Mention any two advantages of using Star topology.
Answer
(i) Microwave links would be the most suitable choice for setting up the network because of their resilience to hilly terrain, high reliability, and cost-effective deployment compared to wired and radio links.
(ii) Video conference would be the best communication method for doctors at the village hospital to consult senior doctors at the base hospital. Video conferences allow for real-time interaction, visual assessment of patients, and the sharing of medical data, making them more effective for remote consultations compared to email, SMS, or telephone calls.
(iii) POP3 (Post Office Protocol version 3) is used to receive emails. POP3 allows users to download emails from a server to their local device and manage them offline.
(iv)
(a) VoIP — Voice over Internet Protocol
(b) HTTPS — Hypertext Transfer Protocol Secure
(v) The advantages of star topology are as follows:
- It is easy to detect faults in this network as all computers are dependent on the central hub.
- The rate of data transfer is fast as all the data packets or messages are transferred through central hub.
Write the output of the code given below:
p=8
def sum(q=5, r=6) :
p=(r+q)**2
print(p, end= '#')
sum(p)
sum(r=3,q=2)
Answer
196#25#
In the given code, the variable p
is initially set to 8, and a function sum
is defined with two parameters q
and r
, each having default values. When the function sum(p)
is called, it overrides the default value of q
with the value of p
, resulting in q
becoming 8. Inside the function, p
is calculated as the square of the sum of q
and r
, which evaluates to 196. The function then prints 196# due to the end='#' parameter in the print statement. Subsequently, the function sum(r=3, q=2)
is called with specific values for q
and r
, which are 2 and 3 respectively. Inside this call, p
is calculated as 25 and printed as 25#. Therefore, the output of the code is 196#25#.
The code given below accepts the roll number of a student and deletes the record of that student from the table STUDENT. The structure of a record of table Student is: RollNo — integer; Name — string; Class — integer; Marks — integer.
Note the following to establish connectivity between Python and MySQL:
• Username is root, Password is A5_b23.
• The table exists in a MySQL database named SCHOOL.
Write the following missing statements to complete the code:
#Statement 1— to create the cursor object
#Statement 2 — to execute the query
#Statement 3 — to make the deletion in the database permanent
import mysql.connector as mysql
def sql data():
conn=mysql.connect(host="localhost",
user="root",
password=" ",
database="school")
............... #Statement 1
rno=int(input("Enter Roll Number : "))
qry="delete from student where RollNo={}".format(rno)
............... #Statement 2
............... #Statement 3
print ("Record deleted")
Answer
#Statement 1 — cursor = conn.cursor()
#Statement 2 — cursor.execute(qry)
#Statement 3 — conn.commit()
The completed code is as follows:
import mysql.connector as mysql
def sql_data():
conn = mysql.connect(host = "localhost",
user = "root",
password = "A5_b23",
database = "school")
cursor = conn.cursor() # Statement 1 - to create the cursor object
rno = int(input("Enter Roll Number: "))
qry = "DELETE FROM student WHERE RollNo={}".format(rno)
cursor.execute(qry) # Statement 2 - to execute the query
conn.commit() # Statement 3 - to make the deletion in the database permanent
print("Record deleted")
What is meant by serialization and deserialization in the context of binary files?
Answer
Serialization is the process of transforming data or an object in memory (RAM) into a stream of bytes called byte streams. These byte streams, in a binary file, can then be stored on a disk, in a database, or sent through a network. The serialization process is also called pickling.
Deserialization or unpickling is the inverse of the pickling process, where a byte stream is converted back into a Python object.
Shreya Sharma, a student of SMS School, has written a program to store Roman numbers and find their equivalents using a dictionary. She has written the following code. As a programmer, help her to successfully execute the given task.
import ............... #Line 1
numericals = {1: 'I', 4 : 'IV', 5: 'V', 9: 'IX',
10: 'X', 40: 'XL', 50: 'L', 90: 'XC',
100: 'C', 400: 'CD', 500: 'D', 900: 'CM',
1000: 'M'}
file1 = open("roman.dat", "...............") #Line 2
pickle.dump(numerals, file1)
file1.close()
file2 = open("roman.dat", " ............... ") #Line 3
num = pickle.load(file2)
file2. ............... #Line 4
n = 0
while n != -1:
print("Enter 1,4,5,9,10,40,50,90,100,400,500,900,1000:")
print("or enter -1 to exit")
n = int(input("Enter numbers"))
if n! = -1:
print("Equivalent roman number of this numeral is : ", num [n])
else:
print("Thank You")
(i) Name the module she should import in Line 1.
(ii) In which mode Shreya should open the file to add data into the file in Line 2.
(iii) Fill in the blank in Line 3 to read the data from a binary file.
(iv) Fill in the blank in Line 4 to close the file.
Answer
(i) Shreya should import the "pickle" module in Line 1 to work with binary file.
(ii) Shreya should open the file in write-binary mode ("wb") in Line 2 to add data to the file.
(iii) Shreya should fill in Line 3 with "rb" to read data from a binary file.
(iv) Shreya should use close() in Line 4 to close the file after reading the data.